Using Pandas to Check for Not Null Values | General Discussion
Pandas is a powerful and flexible data manipulation library for Python. One common operation when working with data is checking for non-null values within a DataFrame. Understanding how to handle null and non-null values effectively is crucial for data cleaning, transformation, and analysis. This article will guide you through the methods available in Pandas to check for non-null values, along with practical examples and best practices.
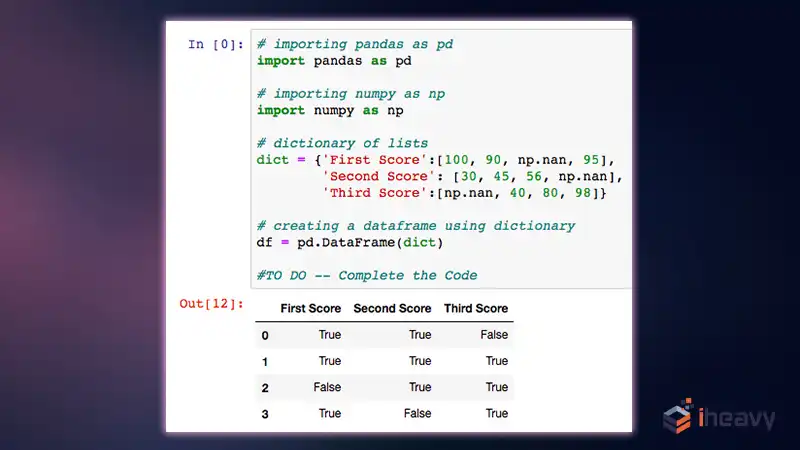
Understanding Null and Non-Null Values in Pandas
In Pandas, null values are represented by NaN (Not a Number). These values can occur due to missing data, calculations that result in undefined values, or explicit insertion of NaN in the data. Checking for non-null values allows you to identify and work with the valid data in your DataFrame.
Methods to Check for Non-Null Values
Using notnull()
The notnull() function is a built-in Pandas method that returns a DataFrame of boolean values indicating whether each element is not null. This method can be applied to both Series and DataFrames.
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, None, 4],
'B': [None, 2, 3, 4]}
df = pd.DataFrame(data)
# Checking for non-null values
non_null_df = df.notnull()
print(non_null_df)
Using notna()
The notna() function is an alias for notnull(). It provides the same functionality, allowing you to check for non-null values in a DataFrame or Series.
# Checking for non-null values using notna()
non_null_df = df.notna()
print(non_null_df)
Filtering Non-Null Rows
To filter and retain only the rows with non-null values in specific columns, you can use the dropna() method or boolean indexing.
Using dropna()
# Dropping rows with any null values
clean_df = df.dropna()
print(clean_df)
# Dropping rows with null values in specific columns
clean_df_specific = df.dropna(subset=['A'])
print(clean_df_specific)
Using Boolean Indexing
# Filtering rows with non-null values in column ‘A’
filtered_df = df[df['A'].notnull()]
print(filtered_df)
Applying notnull() in Conditional Statements
You can use notnull() in conditional statements to perform operations based on the presence of non-null values.
# Adding a new column based on non-null condition
df['C'] = df['A'].notnull()
print(df)
Best Practices
Consistent Handling of Null Values
Ensure consistent handling of null values across your DataFrame to maintain data integrity.
Combine with Other Methods
Use notnull() or notna() in combination with other Pandas methods like fillna() to handle and clean your data effectively.
Performance Considerations
For large DataFrames, consider the performance impact of these operations and use efficient methods to optimize your code.
Frequently Asked Questions
What is the difference between notnull() and notna()?
notnull() and notna() are aliases in Pandas, providing the same functionality. Both methods return a boolean DataFrame indicating non-null values.
Can I use notnull() with a specific column in a DataFrame?
Yes, you can apply notnull() to a specific column to check for non-null values in that column.
non_null_column = df['A'].notnull()
print(non_null_column)
How do I handle null values in my DataFrame?
Pandas provides several methods to handle null values, such as dropna() to remove them and fillna() to replace them with specific values.
# Replacing null values with a specific value
df_filled = df.fillna(0)
print(df_filled)
What should I consider when working with large DataFrames?
For large DataFrames, consider the performance impact of operations involving null values. Use efficient methods and optimize your code to handle large datasets effectively.
How do I check for null values in my DataFrame?
To check for null values, use the isnull() or isna() methods, which return a boolean DataFrame indicating null values.
null_df = df.isnull()
print(null_df)
Conclusion
Checking for non-null values is a fundamental operation when working with data in Pandas. By using methods like notnull() and notna(), you can effectively identify and handle non-null values in your DataFrame. Understanding and managing null and non-null values ensures the integrity and reliability of your data analysis and processing tasks.