SQL Server Check If Table Exists | [Answered]
In SQL Server, ensuring that a table exists before performing operations on it is a common requirement for database administrators and developers. Whether you’re creating, altering, or querying tables, verifying their existence can prevent errors and enhance the robustness of your SQL scripts.
This article will help you discover several methods to check if a table exists in SQL Server, detailing both basic and advanced techniques. So, without further ado, let’s get started.
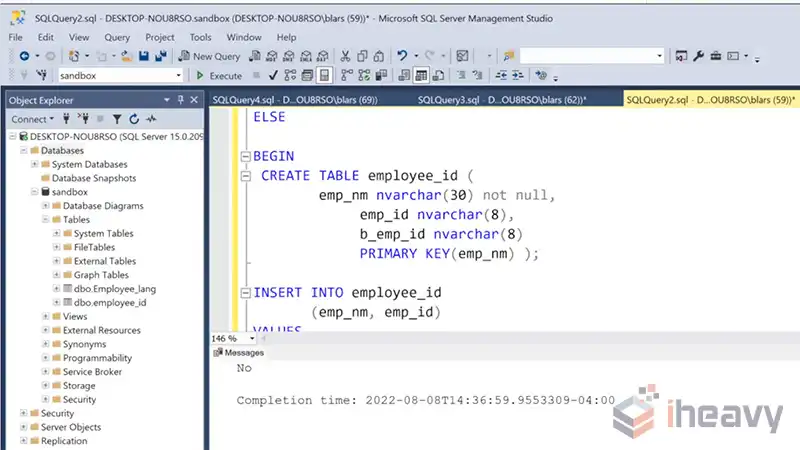
How to Check if a Table Exists in SQL Server
SQL Server provides various methods to check for the existence of a table, each with its own use case and advantages. Let’s see how you can effectively use them in your SQL scripts.
Method 1: Using INFORMATION_SCHEMA.TABLES
The INFORMATION_SCHEMA views provide metadata about database objects. One such view is INFORMATION_SCHEMA.TABLES, which contains information about all tables in the database.
Here’s a simple query to check if a table exists:
IF EXISTS (SELECT 1 FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = 'schema_name'
AND TABLE_NAME = 'table_name')
BEGIN
PRINT 'Table exists'
END
ELSE
BEGIN
PRINT 'Table does not exist'
END
In this query:
- Replace ‘schema_name’ with the schema of the table (e.g., dbo).
- Replace ‘table_name’ with the name of the table you’re checking for.
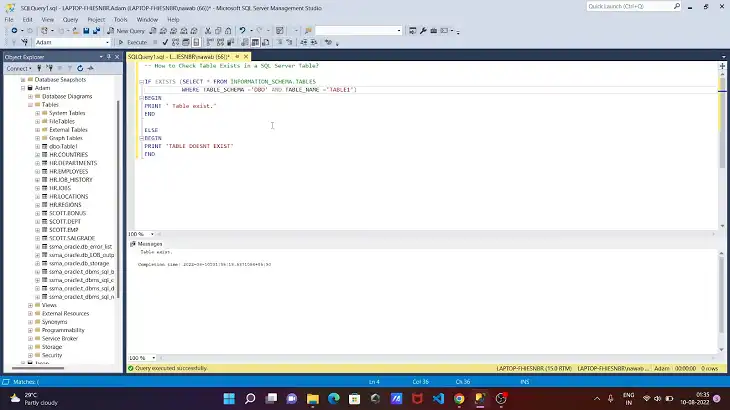
Method 2: Using sys.tables
The sys.tables catalog view is another way to check for the existence of a table. This view provides a list of all user-defined tables in the database.
IF EXISTS (SELECT 1 FROM sys.tables
WHERE schema_id = SCHEMA_ID('schema_name')
AND name = 'table_name')
BEGIN
PRINT 'Table exists'
END
ELSE
BEGIN
PRINT 'Table does not exist'
END
In this query:
- Replace ‘schema_name’ with the schema of the table.
- Replace ‘table_name’ with the name of the table.
Method 3: Using OBJECT_ID
The OBJECT_ID function returns the database object ID for a specified object name. This method is efficient and often used in practice.
IF OBJECT_ID('schema_name.table_name', 'U') IS NOT NULL
BEGIN
PRINT 'Table exists'
END
ELSE
BEGIN
PRINT 'Table does not exist'
END
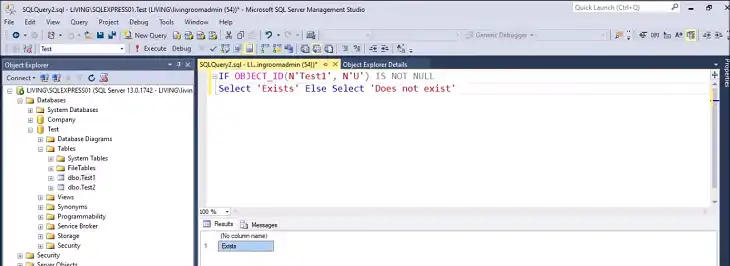
Method 4: Combining with Dynamic SQL
For scenarios where table names or schemas are dynamic, combining OBJECT_ID with dynamic SQL can be useful. This approach allows you to construct SQL statements dynamically and execute them.
DECLARE @schema_name NVARCHAR(128) = 'schema_name';
DECLARE @table_name NVARCHAR(128) = 'table_name';
DECLARE @sql NVARCHAR(MAX);
SET @sql = N'IF OBJECT_ID(N''' + @schema_name + '.' + @table_name + ''', ''U'') IS NOT NULL
PRINT ''Table exists''
ELSE
PRINT ''Table does not exist''';
EXEC sp_executesql @sql;
In this query:
- Replace ‘schema_name’ and ‘table_name’ with the respective schema and table names.
Frequently Asked Questions
What happens if I check for a table that exists in a different schema?
Make sure you specify the correct schema name in your queries. If you omit the schema, the check might return false negatives.
How can I check if a table exists in a specific schema?
When using OBJECT_ID or other methods, you should include the schema name to ensure you’re checking for the table in the correct schema.
Can I check for the existence of a table across multiple databases?
Yes, you can check for a table’s existence across multiple databases by prefixing the database name in your queries.
Conclusion
Checking if a table exists in SQL Server is a common task that can be accomplished using various methods like INFORMATION_SCHEMA.TABLES, OBJECT_ID(), and sys.objects. Each method has its own use case and benefits. Experiment with these methods to find the one that best fits your needs. If you have questions or feedback, feel free to ask. Thank you for reading!