SQL Query to Get the Latest Record by Timestamp
Retrieving the latest record by timestamp is a common requirement in SQL queries, especially when working with time-series data or when you need to fetch the most recent entry from a database table. Whether you are dealing with logs, transactions, or any other time-dependent data, knowing how to construct efficient SQL queries to get the latest record is crucial.
This article provides a comprehensive guide on writing SQL queries to fetch the latest record by timestamp, covering different scenarios and optimizations to ensure the best performance.
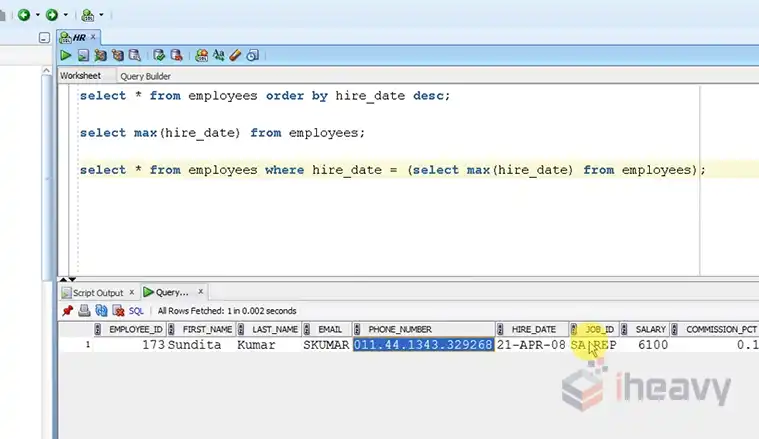
How to Get the Latest Timestamp Record in SQL?
When dealing with time-series data or records that are timestamped, it is essential to know the structure of your table. Typically, a table will have a timestamp column indicating the time when the record was inserted or updated. To fetch the most recent record, you need to identify this column.
Here’s a sample table structure for illustration:
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATETIME,
Amount DECIMAL(10, 2)
);
In the Orders table, OrderDate represents the timestamp column. To get the latest order, you will need to query based on this column.
Fetching the Latest Record by Timestamp
To fetch the latest record by timestamp, you can use the ORDER BY clause combined with the LIMIT clause in SQL. The ORDER BY clause sorts the records based on the specified column, and LIMIT restricts the number of returned records.
Basic SQL Query to Get the Latest Record:
SELECT *
FROM Orders
ORDER BY OrderDate DESC
LIMIT 1;
Explanation:
- SELECT * retrieves all columns from the Orders table.
- ORDER BY OrderDate DESC sorts the records by the OrderDate column in descending order, placing the most recent date first.
- LIMIT 1 ensures that only the first record, which is the latest one, is returned.
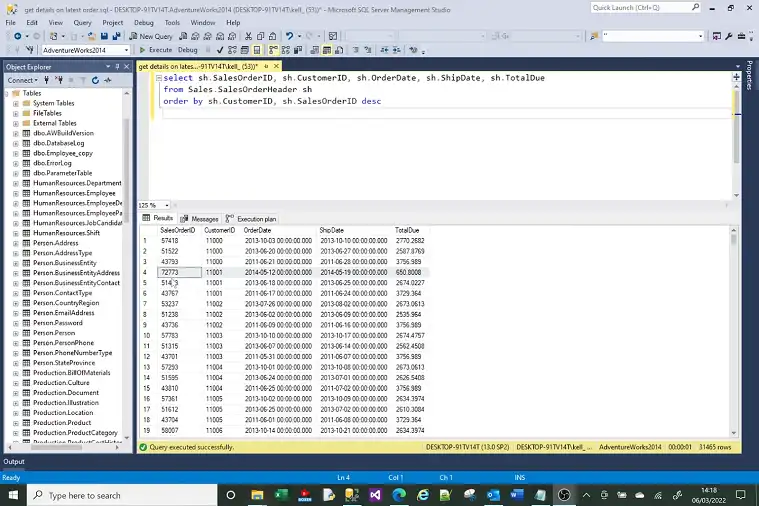
Fetching the Latest Record for Each Group
Sometimes, you may need to retrieve the latest record for each group, such as the latest order for each customer. This requirement adds complexity, but it can still be efficiently handled in SQL.
SQL Query to Get the Latest Record for Each Customer:
SELECT o1.*
FROM Orders o1
JOIN (
SELECT CustomerID, MAX(OrderDate) AS LatestOrder
FROM Orders
GROUP BY CustomerID
) o2 ON o1.CustomerID = o2.CustomerID AND o1.OrderDate = o2.LatestOrder;
Explanation:
- A subquery (o2) is used to find the MAX(OrderDate) for each CustomerID. This subquery retrieves the latest OrderDate for each customer.
- The main query (o1) then joins this result back to the Orders table to fetch the complete record corresponding to the latest order for each customer.
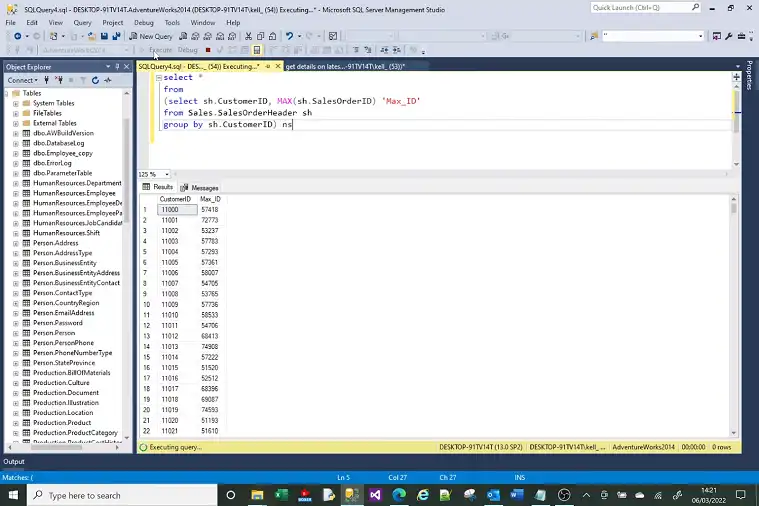
How to Optimize My Query to Retrieve the Latest Record?
Fetching the latest records by timestamp can be optimized with the following tips:
Indexing: Ensure that the timestamp column (OrderDate in this example) is indexed. Indexing significantly improves query performance by allowing the database engine to quickly locate records based on the indexed column.
Avoid Using SELECT *: Instead of selecting all columns, specify only the necessary columns. This reduces the amount of data processed and can speed up the query.
Consider Window Functions: In SQL, window functions provide a powerful way to perform calculations across a set of table rows related to the current row. The ROW_NUMBER() function can be particularly useful for getting the latest record by timestamp.
SQL Query Using Window Function:
SELECT OrderID, CustomerID, OrderDate, Amount
FROM (
SELECT OrderID, CustomerID, OrderDate, Amount,
ROW_NUMBER() OVER (PARTITION BY CustomerID ORDER BY OrderDate DESC) AS RowNum
FROM Orders
) sub
WHERE RowNum = 1;
Explanation:
- The ROW_NUMBER() function assigns a unique sequential integer to rows within a partition of a result set, starting at 1 for the first row in each partition.
- PARTITION BY CustomerID creates a partition for each CustomerID.
- ORDER BY OrderDate DESC orders the rows within each partition by OrderDate in descending order.
- The outer query filters the rows where RowNum = 1, effectively returning the latest record for each customer.
Handling Ties
In some cases, multiple records might have the same latest timestamp. If you want to handle ties explicitly, consider using additional columns to break the tie. For instance, you can order by OrderDate and then by OrderID.
SQL Query to Handle Ties:
SELECT OrderID, CustomerID, OrderDate, Amount
FROM (
SELECT OrderID, CustomerID, OrderDate, Amount,
ROW_NUMBER() OVER (PARTITION BY CustomerID ORDER BY OrderDate DESC, OrderID DESC) AS RowNum
FROM Orders
) sub
WHERE RowNum = 1;
Adding OrderID DESC in the ORDER BY clause breaks the tie by selecting the record with the highest OrderID if multiple records have the same OrderDate.
Frequently Asked Questions
How to recover updated records in SQL Server?
Open SSMS, connect to your SQL Server, right-click the database, and choose “Restore.” Select “From device,” then locate the backup file.
How do I fetch the latest record if my table doesn’t have a timestamp column?
If your table lacks a timestamp column, you’ll need to rely on an alternative column that indicates the order of entries, such as an auto-incrementing ID or a column that stores the last update time. If such a column does not exist, adding one or modifying your table schema might be necessary to keep track of record order.
How can I fetch the latest records from multiple tables in a single query?
You can use JOIN statements to fetch the latest records from multiple tables, as long as there is a common column to join on.
Conclusion
Writing SQL queries to fetch the latest record by timestamp is a common requirement that can be handled effectively with various SQL techniques. Whether you are fetching a single latest record, the latest record per group, or dealing with ties, understanding and applying the right approach ensures efficient and accurate results.