How to Simulate Lost Update in MySQL
Simulating a lost update in MySQL involves demonstrating how concurrent transactions can lead to lost updates when one transaction overwrites changes made by another. This phenomenon is critical to understand for database design, particularly in applications where data integrity and consistency are paramount.
By using transaction isolation levels, particularly the default READ COMMITTED level, one can effectively simulate this scenario. This article explores different methods to create and observe lost updates in MySQL, highlighting how transactions interact with each other under various conditions.
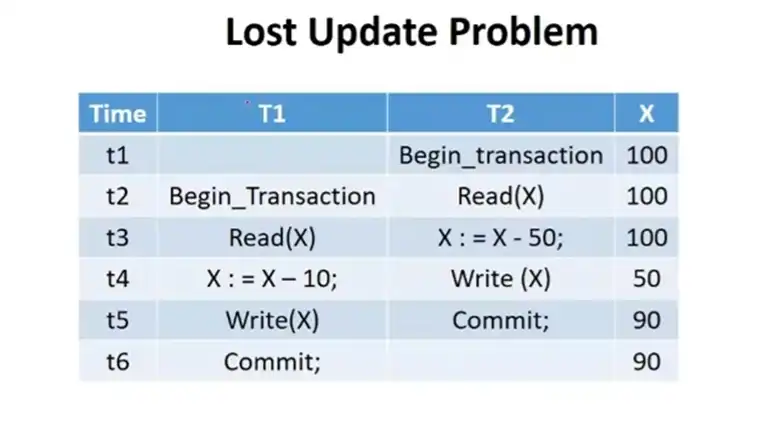
What Is Lost Update in SQL?
A lost update is a concurrency control problem in database systems where two or more transactions access the same data and modify it, leading to a loss of data. This typically occurs when transactions are not properly isolated from each other.
Example:
Consider two transactions, T1 and T2, both trying to update the same bank account balance.
- Transaction T1 reads the current balance (e.g., $100).
- Transaction T2 also reads the current balance (e.g., $100).
- Transaction T1 updates the balance to $200.
- Transaction T2 updates the balance to $150.
In this scenario, both transactions assume the initial balance was $100 and make their updates based on that assumption. However, the final balance will be either $150 or $200, depending on the order in which the transactions commit. This leads to a loss of the update made by one of the transactions.
Simulating Lost Update in MySQL
To begin, a simple table is required to illustrate the concept of lost updates. This setup involves creating a table that will be modified concurrently by multiple transactions.
CREATE TABLE accounts (
id INT PRIMARY KEY,
balance DECIMAL(10, 2)
);
INSERT INTO accounts (id, balance) VALUES (1, 100.00);
The accounts table contains an id and a balance field, which allows for the simulation of concurrent updates.
Method 1: Concurrent Transactions
The first method to simulate a lost update involves executing two transactions simultaneously. This can be done using two separate sessions or terminal windows.
Session 1: Start a transaction and update the balance.
START TRANSACTION;
UPDATE accounts SET balance = balance + 50 WHERE id = 1;
-- At this point, the balance is temporarily 150.00 but not yet committed.
Session 2: Immediately after the first transaction begins, start another transaction and update the same balance.
START TRANSACTION;
UPDATE accounts SET balance = balance - 30 WHERE id = 1;
-- This transaction will modify the balance based on the initial value of 100.00.
After both transactions are executed but before committing either, the following occurs:
- Session 1’s balance is still 150.00 (not committed).
- Session 2 calculates its update based on the original balance of 100.00, resulting in a new balance of 70.00.
When both transactions are committed, the last transaction to commit determines the final state of the data.
COMMIT; -- Assuming Session 1 commits after Session 2, the balance will be 70.00.
In this scenario, the update made by Session 1 was lost because Session 2 overwrote it. This illustrates a classic lost update problem.
Method 2: Using Transaction Isolation Levels
MySQL provides several transaction isolation levels that affect how transactions interact. By setting the isolation level to READ COMMITTED, we can further explore how lost updates can occur.
1. Set the Isolation Level: Change the isolation level in both sessions.
SET SESSION TRANSACTION ISOLATION LEVEL READ COMMITTED;
2. Repeat the Concurrent Transaction Steps: Execute the same steps as in Method 1.
The lost update scenario will manifest similarly because both sessions will read the initial balance of 100.00. This level allows the first transaction to read committed data, yet if it tries to update after the second transaction has modified the balance, it results in lost updates.
Frequently Asked Questions
What causes a lost update in MySQL?
A lost update occurs when two or more transactions read the same data and then write back changes based on the initial read, causing some updates to be overwritten without being applied.
How can MySQL prevent lost updates?
To prevent lost updates, a transaction isolation level of at least REPEATABLE READ is required, which uses shared locks to prevent other transactions from modifying the data.
Conclusion
Simulating lost updates in MySQL is a crucial exercise for developers to grasp the implications of concurrent transactions. By using concurrent transactions and exploring different isolation levels, you can observe how data integrity can be compromised without proper handling of transaction management.