How to Serialize a JGraphT Simple Graph to JSON?
JGraphT is a powerful Java library designed for graph-based algorithms and data structures. It supports various types of graphs, such as directed, undirected, weighted, and unweighted.
However, when working with graphs in JGraphT, there may come a time when you need to serialize a graph structure into a JSON format for storage, transfer, or integration with other systems. In this article, we’ll walk through the process of serializing a SimpleGraph from JGraphT into JSON.
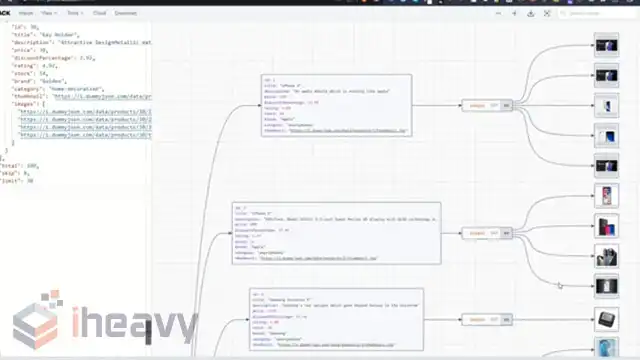
Why Do We Need to Serialize a Graph to JSON?
Serializing a graph to JSON is useful for several reasons:
Data Storage: JSON is a lightweight data format, ideal for storing graph data in files or databases.
Data Transfer: JSON is widely used in APIs, making it easy to transfer graph data between systems or services.
Interoperability: JSON is a standard format supported by many programming languages, enabling easy integration with other software systems.
How Do You Serialize a JGraphT Simple Graph to JSON
Serializing a JGraphT graph to JSON is a 3-step process as described below. However, this method is mostly suitable for ‘simple’ graphs as the title says. With that out of the way, let’s get started.
Prerequisites
To follow along with this tutorial, you’ll need:
JGraphT library: Make sure you have JGraphT added to your project. If you are using Maven, add the following dependency to your pom.xml:
<dependency>
<groupId>org.jgrapht</groupId>
<artifactId>jgrapht-core</artifactId>
<version>1.5.1</version>
</dependency>
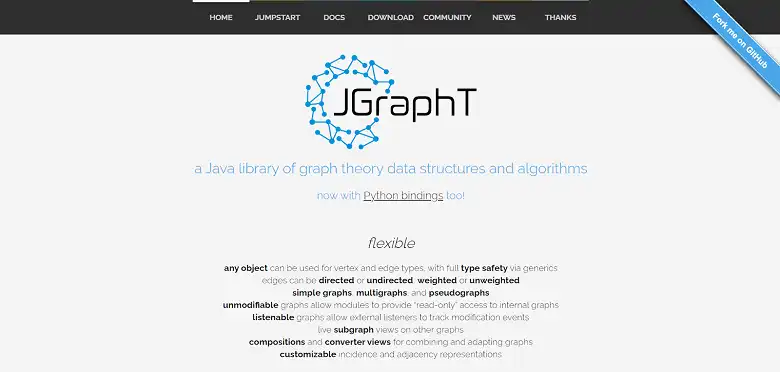
A JSON library: For JSON serialization, you can use libraries like Gson, Jackson, or any other JSON library. For this example, we’ll use Gson. Add the Gson dependency to your pom.xml:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
Step 1: Creating a Simple Graph
First, let’s create a simple undirected graph using JGraphT. We will use DefaultEdge for the edges and strings for the vertex labels.
import org.jgrapht.graph.DefaultEdge;
import org.jgrapht.graph.SimpleGraph;
public class GraphToJsonExample {
public static void main(String[] args) {
SimpleGraph<String, DefaultEdge> graph = new SimpleGraph<>(DefaultEdge.class);
// Add some vertices
graph.addVertex("A");
graph.addVertex("B");
graph.addVertex("C");
// Add some edges
graph.addEdge("A", "B");
graph.addEdge("B", "C");
graph.addEdge("C", "A");
// Now, we will serialize this graph to JSON.
}
}
creates a simple undirected graph using the JGraphT library. It consists of three vertices (A, B, C) and three edges connecting them in a triangular shape.
Step 2: Serialize the Graph to JSON
To serialize the graph, we’ll represent the graph structure in a format that can be easily converted to JSON. A typical approach involves creating a custom class to represent the graph, including its vertices and edges.
import com.google.gson.Gson;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
class GraphJson {
List<String> vertices = new ArrayList<>();
List<EdgeJson> edges = new ArrayList<>();
}
class EdgeJson {
String source;
String target;
EdgeJson(String source, String target) {
this.source = source;
this.target = target;
}
}
public class GraphToJsonExample {
public static void main(String[] args) {
SimpleGraph<String, DefaultEdge> graph = new SimpleGraph<>(DefaultEdge.class);
// Add vertices and edges
graph.addVertex("A");
graph.addVertex("B");
graph.addVertex("C");
graph.addEdge("A", "B");
graph.addEdge("B", "C");
graph.addEdge("C", "A");
// Convert the graph to a custom JSON serializable format
GraphJson graphJson = new GraphJson();
// Add vertices to the graphJson object
Set<String> vertexSet = graph.vertexSet();
graphJson.vertices.addAll(vertexSet);
// Add edges to the graphJson object
Set<DefaultEdge> edgeSet = graph.edgeSet();
for (DefaultEdge edge : edgeSet) {
String source = graph.getEdgeSource(edge);
String target = graph.getEdgeTarget(edge);
graphJson.edges.add(new EdgeJson(source, target));
}
// Convert the GraphJson object to a JSON string using Gson
Gson gson = new Gson();
String jsonString = gson.toJson(graphJson);
// Output the JSON string
System.out.println(jsonString);
}
}
Step 3: Running the Code
When you run the above code, you will see the graph serialized into a JSON format printed in the console. The output should look something like this:
{
"vertices": ["A", "B", "C"],
"edges": [
{"source": "A", "target": "B"},
{"source": "B", "target": "C"},
{"source": "C", "target": "A"}
]
}
Frequently Asked Questions
Can I use other JSON libraries instead of Gson?
Yes, you can use other JSON libraries like Jackson, JSON.simple, or Moshi. The general process remains the same, but the syntax for converting objects to JSON will differ based on the library.
Can I serialize large graphs to JSON?
Serializing very large graphs can result in significant memory usage and large JSON files. For very large graphs, consider using streaming JSON serializers or breaking the graph into smaller parts for serialization.
Conclusion
Serializing a JGraphT SimpleGraph to JSON is a straightforward process. By using a JSON library like Gson, you can easily convert the graph structure into a JSON string. This serialized format is useful for storing, transmitting, or processing graph data in different environments or programming languages.
This approach is flexible and can be extended to more complex graphs or different graph types within the JGraphT library.