RSA_PKCS1_PADDING is No Longer Supported for Private Decryption: Understanding the Changes
RSA_PKCS1_PADDING has been a widely used padding scheme in cryptographic operations, particularly for RSA encryption and decryption. However, recent advancements and security considerations have led to its deprecation for private key decryption in many cryptographic libraries.
This article goes into the reasons behind this change, provides alternative methods for secure encryption and decryption, and offers practical examples with explanations.
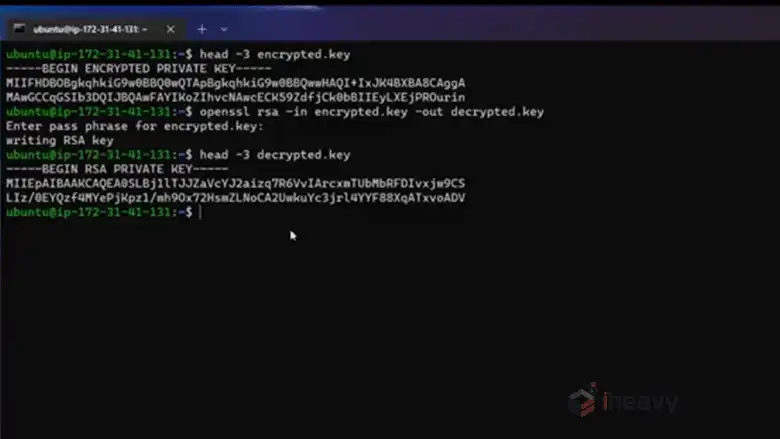
Why is RSA_PKCS1_PADDING No Longer Supported?
The reasons why RSA_PKCS1_PADDING is no longer supported for private decryption are:
Security Vulnerabilities
RSA_PKCS1_PADDING is susceptible to certain types of attacks, such as the Bleichenbacher attack, which exploits the padding scheme to recover plaintext from encrypted data.
This attack involves sending a large number of ciphertexts to be decrypted, analyzing the responses, and eventually deducing the plaintext. The padding’s structure allows attackers to gain information about the plaintext, leading to vulnerabilities that can be exploited in practical scenarios.
Transition to Safer Alternatives
Due to these vulnerabilities, cryptographic libraries and standards have moved towards more secure padding schemes. RSA-OAEP (Optimal Asymmetric Encryption Padding) is one such alternative, offering better security guarantees and resistance to padding oracle attacks.
The cryptographic community and standards bodies like NIST have recommended replacing RSA_PKCS1_PADDING with RSA-OAEP for better security.
What are the Alternatives to RSA_PKCS1_PADDING?
Here’s an alternative to RSA_PKCS1_PADDING that you can consider using.
RSA-OAEP
RSA-OAEP (Optimal Asymmetric Encryption Padding) is a padding scheme designed to address the vulnerabilities in RSA_PKCS1_PADDING. It uses a combination of hashing and randomization to provide stronger security guarantees. Here’s an example of how RSA-OAEP can be used in practice.
Example 1: RSA Encryption and Decryption Using RSA-OAEP
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
from Crypto.Random import get_random_bytes
# Generate RSA keys
key = RSA.generate(2048)
public_key = key.publickey()
# Message to be encrypted
message = b'Hello, RSA-OAEP!'
# Encrypting the message using RSA-OAEP
cipher = PKCS1_OAEP.new(public_key)
encrypted_message = cipher.encrypt(message)
print("Encrypted Message:", encrypted_message)
# Decrypting the message using RSA-OAEP
decipher = PKCS1_OAEP.new(key)
decrypted_message = decipher.decrypt(encrypted_message)
print("Decrypted Message:", decrypted_message.decode())
Explanation:
- Key Generation: We generate a pair of RSA keys using a 2048-bit key size. The public key is extracted for encryption purposes.
- Encryption: The message “Hello, RSA-OAEP!” is encrypted using RSA-OAEP. This involves adding randomness and hashing to protect against certain attacks.
- Decryption: The encrypted message is decrypted using the private key and RSA-OAEP, ensuring the original message is retrieved securely.
Example 2: RSA Encryption with Hybrid Approach
Another approach to encryption is using a hybrid system where RSA is used to encrypt a symmetric key, and the symmetric key is used to encrypt the actual message. This method combines the security of RSA with the efficiency of symmetric encryption.
from Crypto.Cipher import AES
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
from Crypto.Random import get_random_bytes
# Generate RSA keys
key = RSA.generate(2048)
public_key = key.publickey()
# Generate AES session key
session_key = get_random_bytes(16) # AES key length
# Encrypt the session key with the public RSA key
rsa_cipher = PKCS1_OAEP.new(public_key)
encrypted_session_key = rsa_cipher.encrypt(session_key)
# Message to be encrypted
message = b'This is a secret message.'
# Encrypt the message with the AES session key
aes_cipher = AES.new(session_key, AES.MODE_EAX)
ciphertext, tag = aes_cipher.encrypt_and_digest(message)
print("Encrypted Session Key:", encrypted_session_key)
print("Ciphertext:", ciphertext)
print("Nonce:", aes_cipher.nonce)
print("Tag:", tag)
# Decrypt the session key with the private RSA key
rsa_decipher = PKCS1_OAEP.new(key)
decrypted_session_key = rsa_decipher.decrypt(encrypted_session_key)
# Decrypt the message with the AES session key
aes_decipher = AES.new(decrypted_session_key, AES.MODE_EAX, nonce=aes_cipher.nonce)
decrypted_message = aes_decipher.decrypt_and_verify(ciphertext, tag)
print("Decrypted Message:", decrypted_message.decode())
Explanation:
- Session Key Generation: An AES session key is generated randomly. This key will be used for symmetric encryption.
- Encrypting the Session Key: The session key is encrypted using the RSA public key, ensuring it can be securely shared.
- Message Encryption: The actual message is encrypted using AES with the session key. AES.MODE_EAX is used for encryption, providing both confidentiality and authenticity.
- Decrypting the Session Key: The encrypted session key is decrypted using the RSA private key.
- Message Decryption: The message is decrypted using the decrypted session key, ensuring the original message is retrieved.
Example 3: Handling Large Data with RSA and AES
When dealing with large data, RSA encryption can be inefficient. A common solution is to use RSA to encrypt an AES key and use AES for encrypting the data. This combination allows for efficient encryption of large datasets.
from Crypto.Cipher import AES
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
from Crypto.Random import get_random_bytes
def encrypt_data(data, public_key):
# Generate AES session key
session_key = get_random_bytes(16) # AES key length
# Encrypt the session key with the public RSA key
rsa_cipher = PKCS1_OAEP.new(public_key)
encrypted_session_key = rsa_cipher.encrypt(session_key)
# Encrypt the data with the AES session key
aes_cipher = AES.new(session_key, AES.MODE_EAX)
ciphertext, tag = aes_cipher.encrypt_and_digest(data)
return encrypted_session_key, ciphertext, aes_cipher.nonce, tag
def decrypt_data(encrypted_session_key, ciphertext, nonce, tag, private_key):
# Decrypt the session key with the private RSA key
rsa_decipher = PKCS1_OAEP.new(private_key)
decrypted_session_key = rsa_decipher.decrypt(encrypted_session_key)
# Decrypt the data with the AES session key
aes_decipher = AES.new(decrypted_session_key, AES.MODE_EAX, nonce=nonce)
decrypted_data = aes_decipher.decrypt_and_verify(ciphertext, tag)
return decrypted_data
# Generate RSA keys
key = RSA.generate(2048)
public_key = key.publickey()
# Data to be encrypted
large_data = b'This is a large dataset.' * 1024
# Encrypting large data
encrypted_key, ciphertext, nonce, tag = encrypt_data(large_data, public_key)
# Decrypting large data
decrypted_data = decrypt_data(encrypted_key, ciphertext, nonce, tag, key)
print("Decrypted Data:", decrypted_data.decode())
Explanation:
- Encrypting Large Data: The encrypt_data function encrypts large datasets using a hybrid approach, where the AES session key is encrypted with RSA, and the data is encrypted with AES.
- Decrypting Large Data: The decrypt_data function decrypts the data by first decrypting the AES session key using RSA and then decrypting the data with AES.
Frequently Asked Questions
Why is RSA-OAEP considered more secure than RSA_PKCS1_PADDING?
RSA-OAEP provides better security guarantees by using a combination of hashing and randomization. This makes it resistant to padding oracle attacks, which RSA_PKCS1_PADDING is susceptible to.
Can I still use RSA_PKCS1_PADDING for encryption?
While some systems may still support RSA_PKCS1_PADDING for encryption, it is not recommended due to its vulnerabilities. Switching to RSA-OAEP or other secure alternatives is advised.
Conclusion
As cryptography continues to evolve, staying informed about best practices and emerging standards is essential for maintaining robust security in your applications. The deprecation of RSA_PKCS1_PADDING for private key decryption marks a significant shift in cryptographic practices, driven by the need for more secure alternatives.