How to Replace a CASE Statement in SQL
The CASE statement is a powerful tool in SQL for creating conditional logic within your queries. It is similar to if-else statements in programming languages, allowing you to return different values based on specific conditions.
However, there are scenarios where you might want to explore alternatives to CASE statements for simplicity, performance, or readability. In this article, we’ll delve into various methods to replace the CASE statement in SQL, including using conditional aggregation, common table expressions (CTEs), and COALESCE.
What is the CASE Statement?
Before exploring alternatives, let’s quickly review how the CASE statement works.
Basic Structure
SELECT
column1,
column2,
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE result3
END AS alias_name
FROM
table_name;
Example
Suppose you have a table named orders with columns order_id, amount, and status, and you want to categorize orders as “High”, “Medium”, or “Low” based on their amounts.
SELECT
order_id,
amount,
CASE
WHEN amount > 1000 THEN 'High'
WHEN amount BETWEEN 500 AND 1000 THEN 'Medium'
ELSE 'Low'
END AS order_category
FROM
orders;
In this example, the CASE statement categorizes orders based on their amounts.
What Is the Alternative to SQL CASE Statement?
In this section, we’ll explore some common alternatives to the CASE statement and discuss when and why you might choose them.
1. Using Conditional Aggregation
Conditional aggregation can often be used as an alternative to CASE statements, especially when you want to perform aggregate operations based on conditions. This method leverages aggregate functions like SUM, COUNT, MAX, etc., in conjunction with conditions.
Example
Let’s categorize orders again but use conditional aggregation to calculate the total amount for each category.
SELECT
SUM(CASE WHEN amount > 1000 THEN amount ELSE 0 END) AS high_orders_total,
SUM(CASE WHEN amount BETWEEN 500 AND 1000 THEN amount ELSE 0 END) AS medium_orders_total,
SUM(CASE WHEN amount < 500 THEN amount ELSE 0 END) AS low_orders_total
FROM
orders;
This query sums the amount for each category using conditional logic within the SUM function. If a condition is met, the amount is added to the corresponding total.
2. Using COALESCE
COALESCE is a function that returns the first non-null value from a list of expressions. It can sometimes be used as a simple alternative to CASE for handling null values or selecting default values.
Example
Assume we have a column discount_code that might contain null values. We want to replace nulls with “No Code”.
SELECT
order_id,
amount,
COALESCE(discount_code, 'No Code') AS discount_status
FROM
orders;
The COALESCE function checks if discount_code is null and returns “No Code” if it is. Otherwise, it returns the actual discount code.
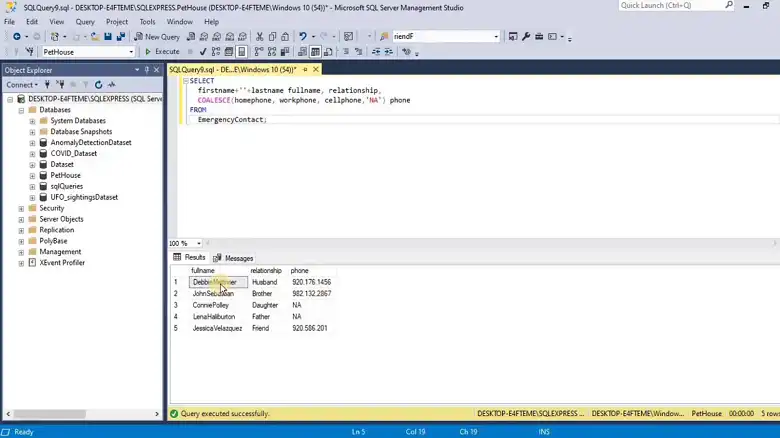
3. Using Common Table Expressions (CTEs)
CTEs allow you to define temporary result sets that can be referred to within a SELECT, INSERT, UPDATE, or DELETE statement. They can simplify complex queries and improve readability.
Example
Let’s use a CTE to categorize orders and then select from the CTE.
WITH OrderCategories AS (
SELECT
order_id,
amount,
CASE
WHEN amount > 1000 THEN 'High'
WHEN amount BETWEEN 500 AND 1000 THEN 'Medium'
ELSE 'Low'
END AS order_category
FROM
orders
)
SELECT
order_id,
amount,
order_category
FROM
OrderCategories;
The CTE OrderCategories performs the categorization, and the final SELECT retrieves the categorized data. This approach improves query organization and readability.
4. Using Nested Queries or Subqueries
Nested queries or subqueries can sometimes replace CASE statements, especially when dealing with complex conditions.
Example
Suppose you want to categorize orders based on their status as well as amount.
SELECT
order_id,
amount,
(SELECT
CASE
WHEN status = 'completed' AND amount > 1000 THEN 'High'
WHEN status = 'completed' AND amount BETWEEN 500 AND 1000 THEN 'Medium'
ELSE 'Low'
END
FROM
orders AS inner_order
WHERE
inner_order.order_id = outer_order.order_id
) AS order_category
FROM
orders AS outer_order;
In this query, a subquery evaluates conditions to determine the order category. This method can be useful when dealing with multiple conditions across different columns.
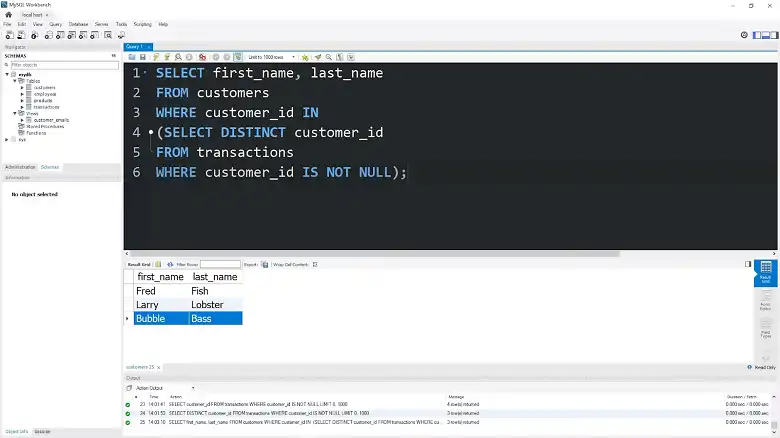
Frequently Asked Questions
What are the performance implications of using CASE statements?
While CASE statements are generally efficient, they can affect performance if used excessively or with complex logic. Alternatives like conditional aggregation or CTEs can sometimes optimize performance by reducing complexity.
When to use case instead of if in SQL?
The IF statement is best suited for simple decisions where there are only two possible outcomes. On the other hand, the CASE statement is more versatile, allowing you to evaluate multiple conditions and produce different results based on those conditions.
How does COALESCE differ from CASE?
COALESCE is specifically designed to handle null values by returning the first non-null value from a list. It is more concise than CASE when dealing with null checks and default values but less flexible for other types of conditional logic.
Conclusion
While CASE statements are a versatile tool in SQL, exploring alternatives can lead to more efficient and readable queries. Conditional aggregation, COALESCE, CTEs, and nested queries offer various approaches to implementing conditional logic, each with its advantages and use cases.
By understanding these alternatives, you can choose the best method for your specific SQL queries and enhance the performance and maintainability of your database interactions.