Why Queries Can Only Use Data from One Table at a Time
When working with SQL databases, understanding how queries interact with tables is crucial. Queries typically pull data from a single table. This restriction allows for more straightforward data retrieval, maintenance, and optimization of query performance.
However, combining data from multiple tables is often necessary in real-world applications. SQL provides various methods to merge data from different tables, each with distinct uses and performance considerations.
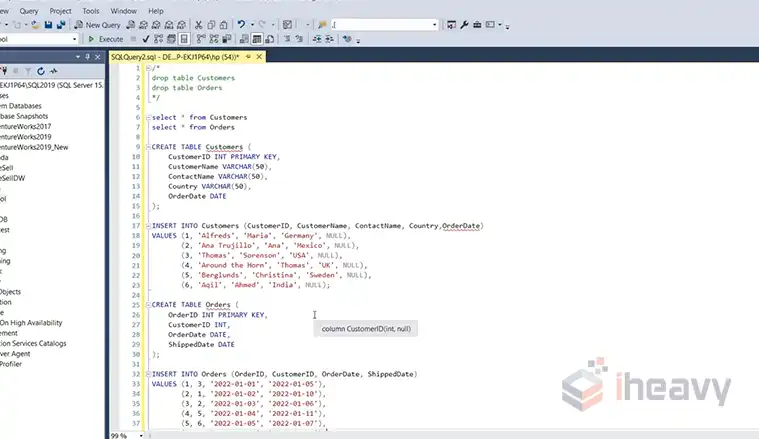
Can You Query Multiple Tables at Once?
Most basic SQL queries retrieve data from one table at a time. These queries are simple and effective for accessing data stored within a single table. For instance, if you want to retrieve all employee names from an “Employees” table, you could use a SELECT statement.
SELECT name FROM Employees;
This query fetches all values in the name column from the “Employees” table. It’s straightforward and efficient, focusing on a single data source.
The restriction of querying one table at a time stems from the need for simplicity and performance. Queries that access only one table are generally faster and easier to optimize. They reduce the complexity involved in data retrieval and ensure that databases can maintain integrity and performance without unnecessary overhead.
How Do I Query Data from Two Tables in a Single Query?
Combining data from more than one table requires special techniques. In SQL, this is achieved using JOIN operations, subqueries, or set operators. Each approach has its specific use cases and benefits. Before diving into these methods, let’s briefly look at why they are necessary.
1. Using JOIN Operations
JOINs are one of the most common ways to combine data from multiple tables. A JOIN operation allows you to create a relationship between two tables based on a related column. There are several types of JOINs, including INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN.
INNER JOIN: This type of JOIN returns only the rows with matching values in both tables.
SELECT Employees.name, Departments.department_name
FROM Employees
INNER JOIN Departments ON Employees.department_id = Departments.id;
This query retrieves the names of employees alongside their corresponding department names. The INNER JOIN ensures that only those employees who have a matching department ID in both the “Employees” and “Departments” tables are selected.
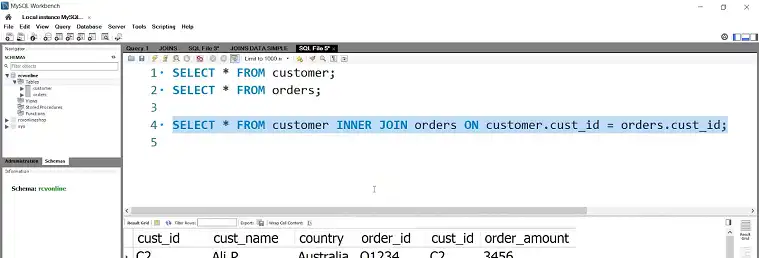
LEFT JOIN: Also known as a LEFT OUTER JOIN, this operation returns all rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for columns from the right table.
SELECT Employees.name, Departments.department_name
FROM Employees
LEFT JOIN Departments ON Employees.department_id = Departments.id;
Here, the query returns all employee names and their department names, including those employees who are not associated with any department. This method is particularly useful for identifying employees who are not assigned to any department.
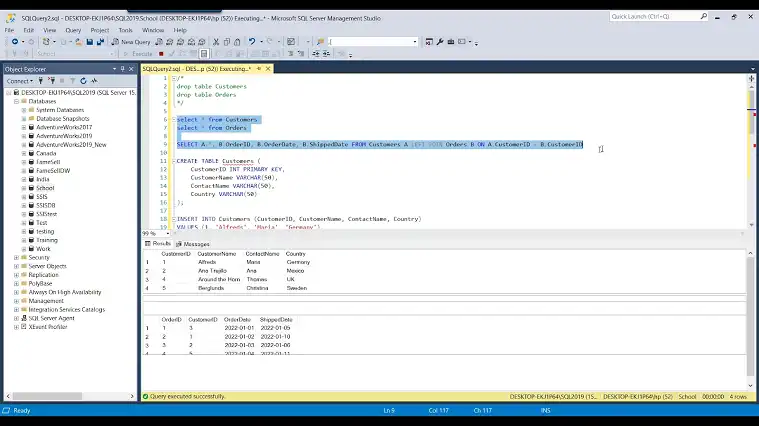
2. Using Subqueries
A subquery, or inner query, is a query nested inside another query. Subqueries can be used to retrieve data that will be used in the main query as a condition to further refine the data retrieved.
SELECT name
FROM Employees
WHERE department_id = (SELECT id FROM Departments WHERE department_name = 'Sales');
In this example, the inner query retrieves the department ID for the ‘Sales’ department, and the outer query uses this ID to find all employees in that department. Subqueries provide a powerful way to filter data dynamically based on another set of criteria.
3. Using Set Operators
Set operators like UNION, INTERSECT, and EXCEPT allow you to perform operations on the result sets of two or more queries. These operators are useful when you need to merge the results of multiple queries that select from different tables but have similar structures.
UNION: Combines the results of two or more queries into a single result set, removing duplicates.
SELECT name FROM Employees
UNION
SELECT name FROM Contractors;
This query merges the names of employees and contractors into one list without any duplicates. Both queries must have the same number of columns and corresponding data types for the UNION to work.
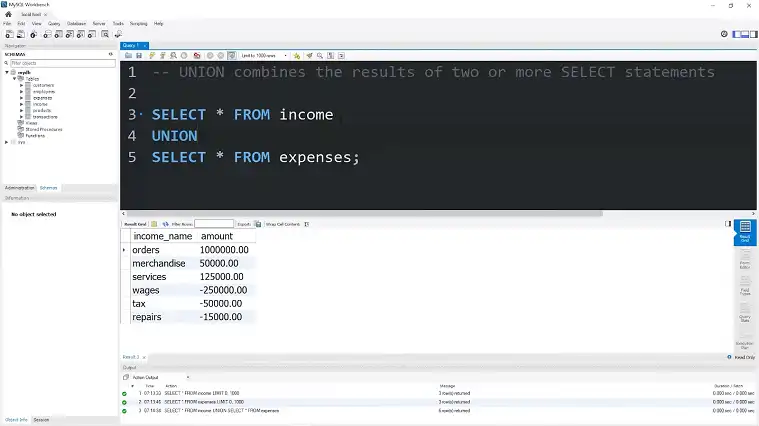
INTERSECT: Returns only the rows that are present in both result sets.
SELECT name FROM Employees
INTERSECT
SELECT name FROM Contractors;
This operation finds names that exist in both the “Employees” and “Contractors” tables. It’s useful when you need to identify common entries between two datasets.
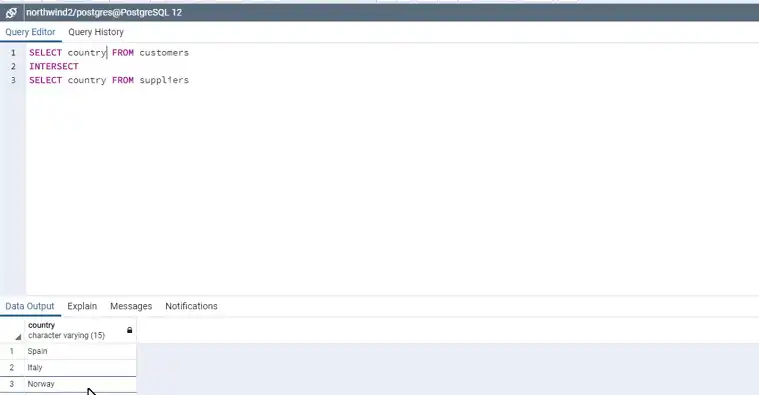
Frequently Asked Questions
How many tables can be joined in a single query?
A single join statement can combine two tables. To join more than two tables, you can chain multiple join statements together, creating a more complex connection between the tables.
Can a query really only use data from one table at a time?
Although a simple SQL query can retrieve data from a single table, SQL provides tools like JOINs, subqueries, and set operators to combine data from multiple tables. So, while basic queries might focus on one table, more complex queries can access data from various sources.
Conclusion
While it’s true that a basic SQL query can focus on a single table, SQL offers powerful mechanisms to combine data from multiple tables. By mastering techniques like JOINs, subqueries, and set operators, you can create complex queries that extract valuable insights from your relational database.
The choice of method depends on your specific requirements and the relationships between the tables involved. You can try out different approaches to find the most efficient and effective way to combine data from multiple sources.