Preserve Leading Zeros SQL Data Types
Preserving leading zeros in SQL data types can be essential for various applications, such as managing codes, identifiers, or numbers with specific formats.
In this article, we will explore why preserving leading zeros is important, the common SQL data types used to store these values, and the methods to ensure they are maintained when stored and retrieved from a database.
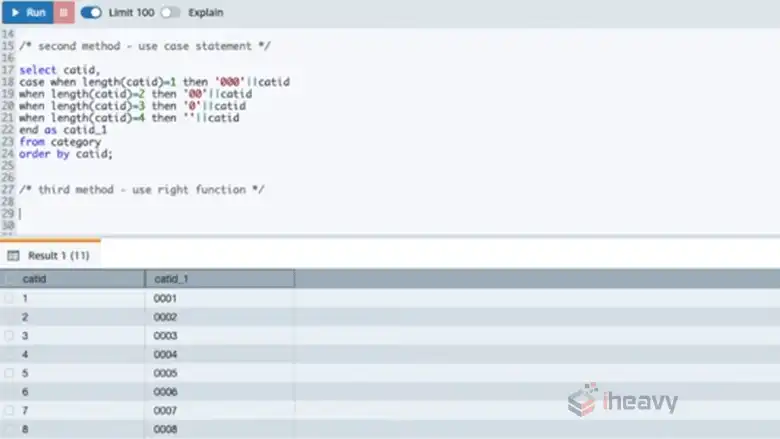
Why Preserving Leading Zeros Matters?
Leading zeros are crucial in data fields such as:
- Zip Codes: Postal codes in some regions include leading zeros (e.g., 00123).
- Product Codes: SKU numbers often have fixed lengths, where leading zeros are used (e.g., 00056789).
- Identifiers: Employee IDs, account numbers, or other identifiers might need to preserve formatting (e.g., 001234).
Failure to preserve leading zeros can lead to data inaccuracies, formatting issues, and misunderstandings, especially when data is exported to other systems or presented to users.
Common SQL Data Types and Challenges
In SQL, data types are essential in defining how data is stored. Here are some common data types and the challenges they present when dealing with leading zeros:
1. Integer
- Challenge: Integer data types inherently do not store leading zeros. For instance, storing 007 as an integer would result in 7.
- Use Case: Suitable for calculations but not for storing numbers where formatting is crucial.
2. String (CHAR, VARCHAR)
- Solution: String data types can store leading zeros without any issues.
- Use Case: Ideal for data fields where the format is essential, like zip codes or product codes.
3. Decimal/Numeric
- Challenge: Although these types store numbers with decimal precision, they do not preserve leading zeros for integer parts.
- Use Case: Primarily used for financial calculations and precise numerical data.
How Do You Keep Leading Zeros in SQL?
To ensure leading zeros are preserved, consider the following strategies:
1. Use String Data Types
When the number format is essential, using string data types is the simplest way to preserve leading zeros. Here’s an example using VARCHAR in SQL:
CREATE TABLE products (
product_code VARCHAR(10)
);
INSERT INTO products (product_code)
VALUES ('00123'), ('00056789');
In this example, product_code is stored as a VARCHAR, ensuring that any leading zeros are preserved.
2. Format Numbers on Retrieval
If the numbers are stored without leading zeros, you can format them when retrieving. Here’s an example using MySQL:
SELECT LPAD(zip_code, 5, '0') AS formatted_zip_code
FROM addresses;
The LPAD function pads the zip_code with zeros to a total length of 5.
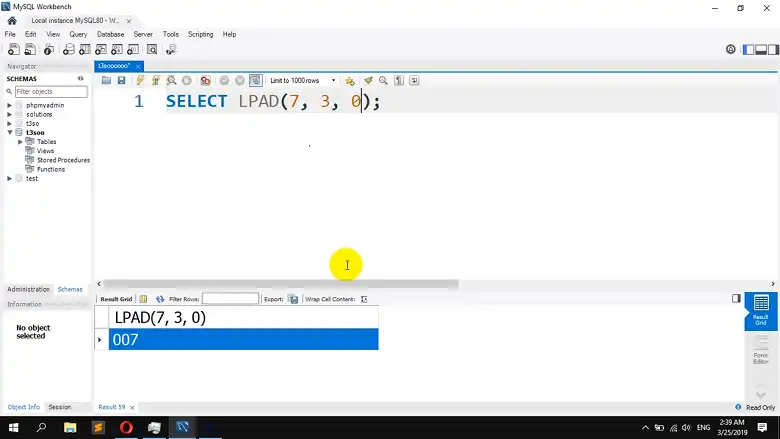
3. Use a Fixed-Length String (CHAR)
For data fields with a consistent length, using CHAR ensures the data maintains its intended format:
CREATE TABLE accounts (
account_number CHAR(10)
);
INSERT INTO accounts (account_number)
VALUES ('0001234567');
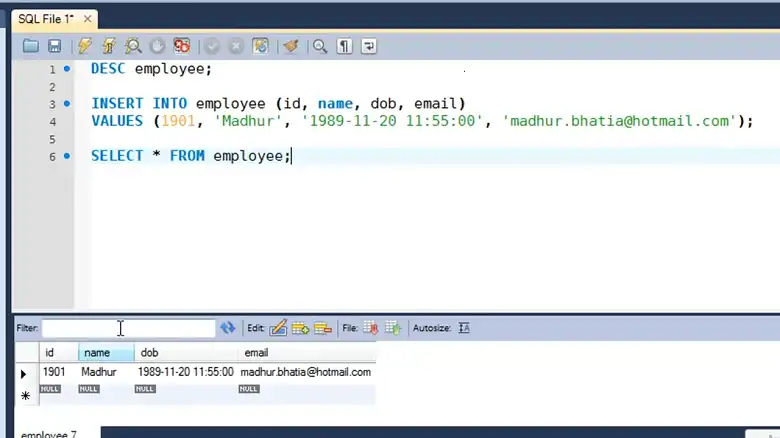
4. Client-Side Padding
In some cases, handling leading zeros might be more efficient on the client side, especially if the database is used for calculations. For instance, you could use a programming language to format numbers before insertion or after retrieval.
Here’s a Python example using the Pandas library:
import pandas as pd
# Assume df is a DataFrame with a column named ‘product_code’
df[‘product_code’] = df[‘product_code’].apply(lambda x: f'{int(x):08d}’)
5. Use of SQL Functions
SQL provides functions to help maintain formatting:
- LPAD: Pads the left side of a string with characters.
- RPAD: Pads the right side of a string with characters.
- FORMAT: Formats a number as a string.
Example Using LPAD
SELECT LPAD(employee_id, 6, '0') AS formatted_id
FROM employees;
This query formats employee_id to always be 6 characters long, padding with zeros as needed.
Frequently Asked Questions
What does preserving leading zeros mean?
Leading zeros can act as a security measure by preventing fraudulent alterations. For instance, by including leading zeros in check amounts, it becomes significantly harder for someone to modify the value without detection.
What data type to store 1 or 0 in SQL?
To store raw binary data, such as image files or encrypted information, SQL offers the BINARY and VARBINARY data types. BINARY stores fixed-length binary sequences, while VARBINARY handles variable-length binary data.
Conclusion
Preserving leading zeros in SQL is crucial for ensuring data integrity and accuracy in applications where the format of numbers is important. By choosing the appropriate data types and implementing strategies for formatting and retrieval, you can effectively maintain the required formatting of numbers in your database.