Pandas GroupBy Without Aggregation | Explained
The groupby() function in Pandas splits data into groups based on some criteria. Aggregation functions such as count(), max(), min(), mean(), std(), and describe() operate on these groups to provide summary statistics. Typically, these functions are combined to get multiple aggregation results on specific columns.
However, there are cases where you might want to use groupby without directly applying aggregation. This approach can be beneficial when you need to process grouped data separately before performing any aggregation.
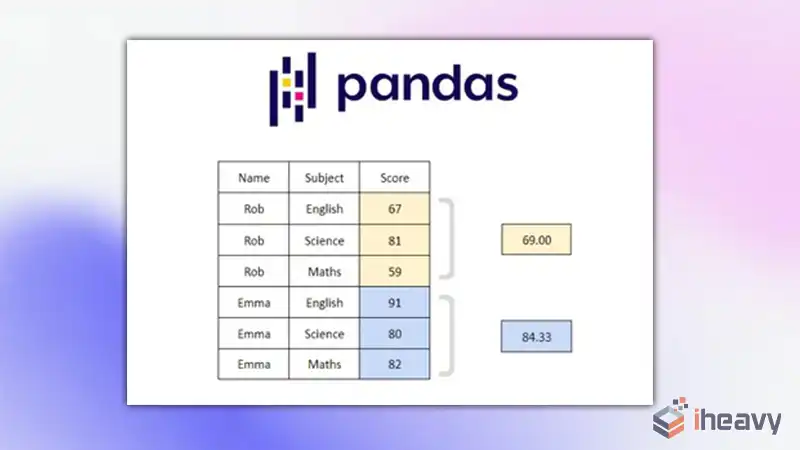
How to Use Pandas Groupby Without Aggregation
Let’s explore this concept using a different dataset from the seaborn library: the tips dataset, which contains information about tips given in a restaurant. We’ll focus on grouping the data by the day column without immediate aggregation.
First, let’s import the necessary libraries and load the dataset:
import pandas as pd
import seaborn as sns
# Load the tips dataset
df = sns.load_dataset('tips')
# Display the first few rows of the DataFrame
print(df.head())
We can see various columns, including total_bill, tip, sex, smoker, day, time, and size.
To understand the data better, let’s look at the summary of the day and total_bill columns:
print(df['day'].describe())
print(df['total_bill'].describe())
Next, let’s use groupby to split the data by day and then define a function that calculates the mean of the total_bill and tip for each group. This function will add these means as new columns in the original DataFrame:
# Select relevant columns
df1 = df[['total_bill', 'tip', 'day']]
# Function to compute means for each group and add them as new columns
def add_mean_columns(group):
total_bill_mean = group['total_bill'].mean()
tip_mean = group['tip'].mean()
group['Mean total bill'] = total_bill_mean
group['Mean tip'] = tip_mean
return group
# Applying the function to each group
df2 = df1.groupby('day').apply(add_mean_columns)
print(df2.head(10))
In this example, we added the mean total_bill and tip for each day as new columns in the DataFrame. This allows us to preserve the original data while adding group-specific statistics.
Frequently Asked Questions
Can you group by without aggregate?
We can use GROUP BY without using an aggregate function. In this context, GROUP BY behaves much like a DISTINCT clause, ensuring that the output includes only unique values and excludes duplicates from the result set.
What is the difference between aggregate and group by?
The aggregate function is specified within the SELECT statement, where its result appears as an extra column. Meanwhile, the GROUP BY clause determines how the output should be grouped based on specific columns. It’s common to combine the GROUP BY clause with the WHERE and HAVING clauses to filter the results.
Conclusion
Using groupby without aggregation in Pandas allows for more flexible data manipulation. It enables us to perform operations on grouped data separately before reducing them to summary statistics. This method is particularly useful when you need to retain the original data while adding meaningful group-specific information.