MySQL Query Optimization Techniques
MySQL is one of the most popular open-source relational database management systems (RDBMS) used by developers worldwide. As data grows in volume and complexity, optimizing MySQL queries becomes crucial for ensuring applications run efficiently and effectively.
This article will explore various techniques and best practices for optimizing MySQL queries, helping you improve performance and reduce response times.
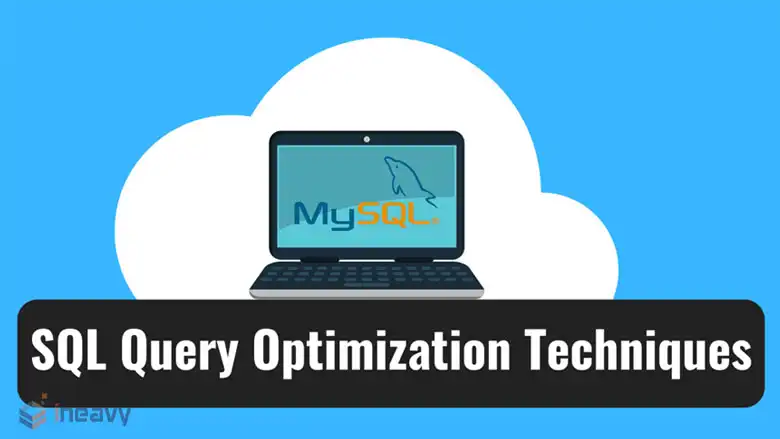
How Can You Optimize a MySQL Query for Better Performance?
Below are some techniques and strategies to improve query speed, reduce resource consumption, and enhance overall database efficiency.
How to Analyze MySQL Query Performance?
Before optimizing any query, it’s essential to understand how MySQL executes it. The EXPLAIN statement provides insights into how MySQL plans to execute a query. By analyzing the execution plan, you can identify potential bottlenecks and areas for improvement.
Example:
EXPLAIN SELECT * FROM orders WHERE customer_id = 1234;
The output will show information such as:
- Select Type: The type of query (e.g., simple, primary, subquery).
- Table: The table being accessed.
- Type: The join type (e.g., ALL, index, range).
- Possible Keys: Indexes that may be used.
- Key: The actual index used.
- Rows: Estimated rows to be scanned.
- Extra: Additional information (e.g., “Using where”).
Actionable Steps:
- Look for ALL in the type column, indicating a full table scan.
- Ensure the query uses indexes effectively.
- Minimize the number of rows scanned by optimizing conditions.
How to Optimize MySQL Query with Index?
Indexes are one of the most effective tools for speeding up query performance. They help MySQL quickly locate the data without scanning the entire table. However, over-indexing can also lead to performance issues, especially during write operations.
Best Practices:
- Create Indexes on Columns Used in WHERE Clauses: This can significantly speed up data retrieval.
- Use Composite Indexes for queries involving multiple columns.
- Avoid Unnecessary Indexes: Each index consumes additional disk space and affects write performance.
- Use Indexes for JOINs: Ensure that the columns used in JOIN operations are indexed.
Example:
CREATE INDEX idx_customer_id ON orders(customer_id);
Considerations:
- Unique Indexes: Use unique indexes for columns with unique values to improve lookup speed.
- Full-Text Indexes: For text-heavy search fields, consider using full-text indexes.
Optimize JOIN Operations
JOINs are common in SQL queries but can become performance bottlenecks if not optimized properly.
Techniques:
- Use Smaller Result Sets: Filter data before joining to minimize the number of rows processed.
- Index Joined Columns: Ensure that columns used in JOIN conditions have appropriate indexes.
- Choose the Right JOIN Type: Understand the difference between INNER JOIN, LEFT JOIN, RIGHT JOIN, etc., and use them judiciously.
Example:
SELECT o.order_id, c.name
FROM orders o
JOIN customers c ON o.customer_id = c.id
WHERE o.order_date > '2024-01-01';
Actionable Steps:
- Analyze the query plan to ensure indexes are used efficiently.
- Reorder joins to process smaller tables first, reducing the data load.
Limit the Result Set
Retrieving only the necessary data can drastically reduce query execution time. Use the LIMIT clause to restrict the number of rows returned.
Example:
SELECT * FROM orders ORDER BY order_date DESC LIMIT 10;
Benefits:
- Reduces the amount of data processed and transferred.
- Improves response times for applications requiring only a subset of data.
Optimize Subqueries and Derived Tables
Subqueries and derived tables can sometimes lead to inefficient execution plans. Consider alternatives such as JOINs or common table expressions (CTEs).
Techniques:
Rewrite Subqueries as JOINs: This can sometimes improve performance by leveraging indexes better.
-- Subquery
SELECT * FROM orders WHERE customer_id IN (SELECT id FROM customers WHERE status = 'active');
-- Rewritten as JOIN
SELECT o.* FROM orders o JOIN customers c ON o.customer_id = c.id WHERE c.status = 'active';
Use CTEs for Complex Queries: CTEs can enhance readability and sometimes performance.
WITH active_customers AS (
SELECT id FROM customers WHERE status = 'active'
)
SELECT * FROM orders WHERE customer_id IN (SELECT id FROM active_customers);
Avoid Using SELECT *
Using SELECT * retrieves all columns from a table, which can be inefficient if you only need a few columns. Specify only the necessary columns to reduce the amount of data processed and transferred.
Example:
-- Inefficient
SELECT * FROM orders WHERE order_date > '2024-01-01';
-- Optimized
SELECT order_id, order_date FROM orders WHERE order_date > '2024-01-01';
Optimize Data Types
Choosing the right data types for your columns can have a significant impact on performance.
Best Practices:
- Use Smaller Data Types: Choose the smallest data type that can hold your data. For example, use TINYINT instead of INT for small numbers.
- Use Fixed-Length Types: For columns with fixed-length data (e.g., CHAR), use fixed-length types for better performance.
- Normalize Your Data: Avoid storing redundant data and use foreign keys to reference related tables.
Example:
-- Instead of using INT for a column that holds age, use TINYINT
CREATE TABLE users (
id INT PRIMARY KEY,
age TINYINT,
...
);
Caching and Query Results
Leveraging caching can significantly improve performance, especially for read-heavy applications.
Techniques:
Use Query Caching: MySQL’s query cache stores the result of a query and reuses it when the same query is executed, reducing processing time.
-- Enable query cache
SET GLOBAL query_cache_size = 1048576; -- Set cache size to 1MB
Use External Caching Solutions: Integrate with caching layers like Memcached or Redis for frequently accessed data.
Considerations:
MySQL query caching can be limited in scenarios with frequent data changes. External caching solutions may offer more flexibility.
Use Partitioning for Large Tables
Partitioning divides a large table into smaller, more manageable pieces. This can improve query performance by reducing the amount of data scanned.
Types of Partitioning:
- Range Partitioning: Divide data based on ranges of values.
- List Partitioning: Use a predefined list of values for partitioning.
- Hash Partitioning: Use a hash function to distribute data evenly.
- Key Partitioning: Use a key to partition data.
Example:
CREATE TABLE orders (
order_id INT,
order_date DATE,
...
)
PARTITION BY RANGE (YEAR(order_date)) (
PARTITION p2022 VALUES LESS THAN (2023),
PARTITION p2023 VALUES LESS THAN (2024)
);
Benefits:
- Faster query performance for specific partitions.
- Improved maintenance and management of large datasets.
Monitor and Tune Server Performance
Regularly monitoring MySQL server performance is crucial for identifying potential issues and ensuring optimal performance.
Tools:
- MySQL Performance Schema: Provides insights into server performance and bottlenecks.
- MySQL Enterprise Monitor: Offers advanced monitoring and analysis features.
- Third-Party Tools: Solutions like Percona Monitoring and Management (PMM) provide comprehensive insights into MySQL performance.
Best Practices:
- Tune MySQL Configuration: Adjust settings like innodb_buffer_pool_size, query_cache_size, and max_connections based on your workload.
- Analyze Slow Query Logs: Identify and optimize slow-running queries.
Frequently Asked Questions
What is a query optimizer in MySQL?
MySQL offers several mechanisms for influencing query optimization, including system variables that control query plan generation, toggleable optimization techniques, explicit hints for index and optimizer behavior, and a configurable cost-based optimizer model.
What is the optimization tool for MySQL?
Database Performance Analyzer (DPA) is a specialized tool designed to monitor and optimize MySQL databases in development, testing, and production environments with minimal performance overhead (less than 1%).
Conclusion
Optimizing MySQL queries is a continuous process that requires careful analysis and understanding of your database schema, workload, and specific use cases. By implementing the techniques discussed in this article, you can improve query performance, reduce response times, and ensure your applications run smoothly even as data volumes grow.