Why is My JavaScript Class Not Working?
JavaScript classes, introduced in ECMAScript 6 (ES6), provide a more straightforward and syntactically appealing way to create objects and manage inheritance.
However, there are instances when you may run into issues where your JavaScript classes don’t work as expected. This article will explore some common pitfalls and how to resolve them.
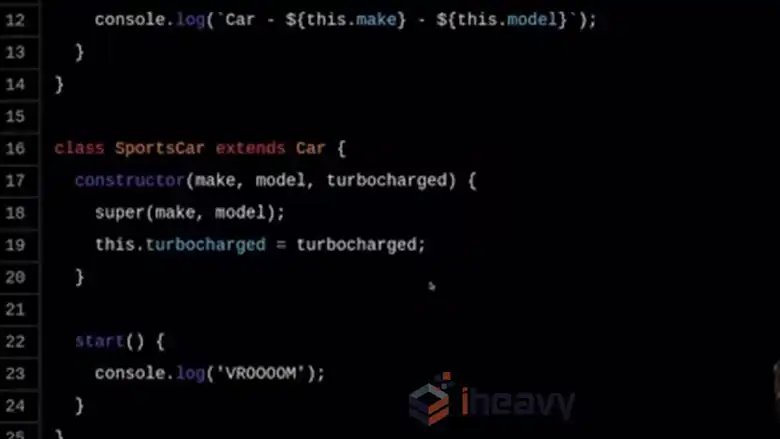
How Class Works in JavaScript
Before diving into the problems, let’s quickly recap what a JavaScript class is. A class is essentially a blueprint for creating objects with predefined properties and methods. Here’s a simple example:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
const john = new Person('John', 30);
john.greet(); // Output: Hello, my name is John and I am 30 years old.
Why doesn’t My JavaScript Class Work?
Below are common issues why JavaScript classes might not work and ways to fix them.
a) Syntax Errors
One of the most common reasons a JavaScript class might not work is due to syntax errors. JavaScript has specific syntax rules for defining classes. For example:
Missing the constructor method: Every class must have a constructor method if you want to initialize properties when creating an instance.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
Incorrect method definitions: Methods in a class should not use the function keyword.
class Person {
greet() { // Correct: No 'function' keyword needed
console.log('Hello!');
}
}
Using the wrong this context: The this keyword in JavaScript can sometimes be tricky. Inside a class method, this refers to the instance of the class, but if a method is used as a callback or event handler, the context of this might change.
class Button {
constructor(label) {
this.label = label;
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log(`Button ${this.label} clicked`);
}
}
const btn = new Button('Submit');
document.querySelector('button').addEventListener('click', btn.handleClick);
If you don’t bind this in the constructor or use an arrow function, this will refer to the button element instead of the class instance.
b) Inheritance Issues
JavaScript classes support inheritance, allowing one class to inherit properties and methods from another. However, mistakes during inheritance can cause the class to fail.
Forgetting to call super(): When a class extends another class, you must call super() in the constructor before accessing this.
class Animal {
constructor(name) {
this.name = name;
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name); // Must call super() before accessing 'this'
this.breed = breed;
}
}
Incorrect method overriding: If a subclass method needs to override a superclass method, ensure that the method signature (parameters) is correct. Otherwise, the superclass method might not behave as expected.
c) Not Understanding Class Properties
JavaScript class properties (fields) are still a relatively new feature. Unlike methods, these properties are defined directly within the class body, and their behavior can sometimes be misunderstood.
Uninitialized class fields: If a class field is not initialized, it might lead to unexpected behavior or undefined errors.
class Counter {
count = 0; // Class field
increment() {
this.count++;
}
}
const counter = new Counter();
counter.increment();
console.log(counter.count); // Output: 1
Static properties and methods: Static properties and methods belong to the class itself, not the instances. If you try to access a static method through an instance, it won’t work.
d) Not Handling Async Code Correctly
When dealing with asynchronous operations inside a class, it’s crucial to manage async/await or Promise correctly.
Forgetting to use await: If you forget to use await for an asynchronous method, it returns a Promise instead of the expected value.
class DataFetcher {
async fetchData() {
const data = await fetch('https://api.example.com/data');
return data.json();
}
}
const fetcher = new DataFetcher();
fetcher.fetchData().then(data => console.log(data));
Frequently Asked Questions
How to access a class in JS?
To find an element with a specific class in a JavaScript document, you can use the `document.querySelector()` method and specify the class name within it.
What should I use instead of classes in JavaScript?
An alternative to using classes in JavaScript is the factory pattern. This approach involves creating objects through specialized functions called factory functions, rather than using constructors or classes. Factory functions can offer advantages in certain situations.
Conclusion
JavaScript classes are a powerful tool, but they require careful attention to detail to ensure they work correctly. Whether it’s syntax errors, inheritance issues, or problems with asynchronous code, taking the time to debug and understand these areas will help you write more robust and reliable JavaScript classes.