Understanding “java.sql.SQLException | Exhausted Resultset” in Java SQL
When working with databases in Java applications, encountering exceptions like “java.sql.SQLException: Exhausted Resultset” is not uncommon. This error typically occurs when attempting to access a result set beyond its available data. In this article, we’ll explore the causes of this exception, how to handle it, and some frequently asked questions about it.
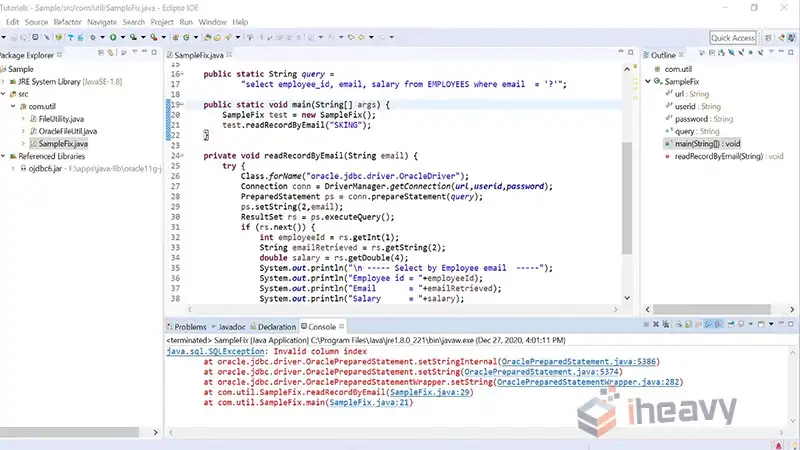
Understanding “Exhausted Resultset” Exception
The “Exhausted Resultset” exception indicates that all the rows in the result set have been traversed, and there are no more rows left to retrieve. This situation can occur when iterating through the result set using methods like next() without checking if there are more rows available.
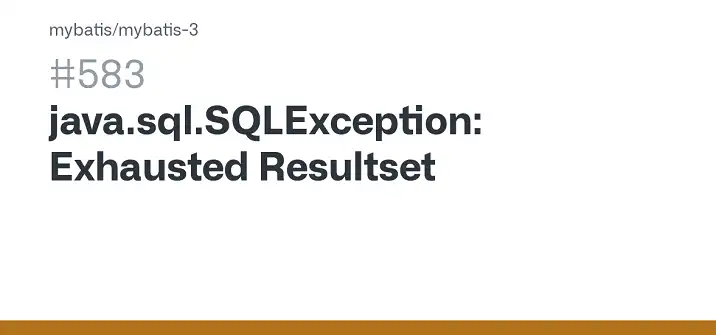
Causes of the Exception
There are several common reasons why you might encounter the “Exhausted Resultset” exception:
Iterating Beyond Available Rows
If your code attempts to retrieve more rows from the result set than are actually present, you’ll encounter this exception. It’s essential to check if there are more rows available using the next() method before attempting to access them.
Closing Resultset Prematurely
If the result set is closed before all rows have been processed, attempting to access additional rows will result in the “Exhausted Resultset” exception. Make sure to close result sets only after you’ve finished processing all rows.
Concurrency Issues
If multiple threads are accessing the same result set concurrently without proper synchronization, it can lead to unexpected behavior, including the “Exhausted Resultset” exception. Ensure proper synchronization to prevent concurrent access issues.
Handling the Exception
To prevent the “Exhausted Resultset” exception, follow these best practices
Step 1
Always check if there are more rows available before iterating through the result set using the next() method. You can use a loop like while (resultSet.next()) to iterate through all rows safely.
Step 2
Close result sets, statements, and connections in a finally block to ensure they are properly closed, even if an exception occurs during processing.
Step 3
Use try-with-resources statements when working with JDBC resources to automatically close them after use, reducing the risk of resource leaks.
Frequently Asked Questions (FAQ)
How can I avoid the “Exhausted Resultset” exception when iterating through a result set?
To avoid this exception, always check if there are more rows available using the next() method before attempting to access them. Use a loop like while (resultSet.next()) to safely iterate through all rows.
What should I do if I encounter the “Exhausted Resultset” exception in my Java application?
If you encounter this exception, review your code to ensure that result sets are being accessed and closed properly. Check for any concurrency issues or logic errors that may be causing premature closure of result sets.
Can database configuration or server settings affect the occurrence of the “Exhausted Resultset” exception?
While the exception is typically caused by issues in application code, database configuration or server settings could potentially contribute to its occurrence. Ensure that your database connection pool settings and database configuration are properly configured to handle the expected workload and concurrency.
Conclusion
The “java.sql.SQLException: Exhausted Resultset” exception in Java SQL indicates that all rows in a result set have been traversed, and there are no more rows left to retrieve. By following best practices for result set iteration, resource management, and concurrency handling, you can effectively prevent and handle this exception in your Java applications.