How to Select All But One Column in SQL | Explained
When working with SQL databases, there are times when you need to select all columns from a table except one. This scenario often arises when dealing with large tables where manually specifying each column except one can be cumbersome and error-prone. This article explores various methods to achieve this efficiently.
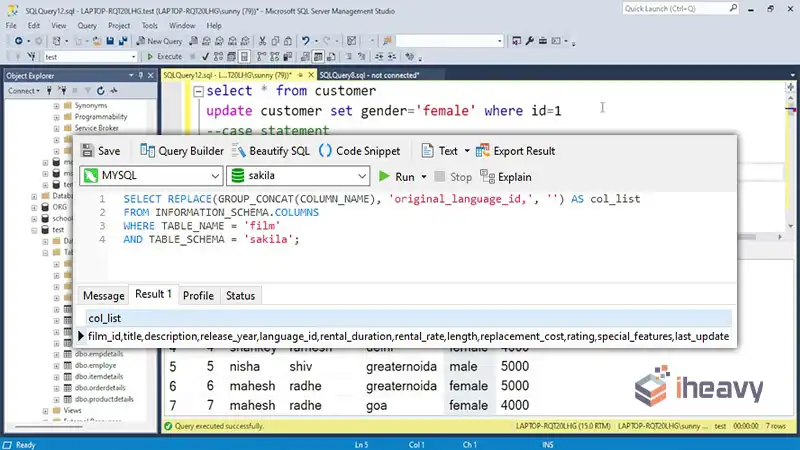
Why Would You Need to Select All But One Column
Selecting all columns except one can be useful in several situations:
Data Privacy
To exclude sensitive data such as passwords or personal information.
Performance Optimization
To exclude large text or binary fields that are not needed.
Simplified Queries
To keep queries clean and manageable without listing all columns explicitly.
Methods to Select All But One Column
Using Explicit Column Names
The most straightforward method is to explicitly list all columns you want to include in the SELECT statement, excluding the one you don’t need.
SELECT column1, column2, column3
FROM table_name;
While this method is simple, it can become impractical with tables that have many columns.
Using Dynamic SQL
For larger tables, dynamic SQL can be used to programmatically generate the column list, excluding the unwanted column.
DECLARE @columns NVARCHAR(MAX);
SELECT @columns = STRING_AGG(column_name, ', ')
FROM information_schema.columns
WHERE table_name = 'table_name' AND column_name != 'unwanted_column';
DECLARE @sql NVARCHAR(MAX);
SET @sql = 'SELECT ' + @columns + ' FROM table_name';
EXEC sp_executesql @sql;
This script dynamically builds the column list and executes the query. It is particularly useful when dealing with tables with many columns.
Using Views
Creating a view can be a practical solution if you need to exclude the same column(s) regularly.
CREATE VIEW view_name AS
SELECT column1, column2, column3
FROM table_name;
You can then use the view to select all columns except the excluded one.
SELECT * FROM view_name;
Using ORM and Code Logic
In application code, Object-Relational Mappers (ORMs) can handle such scenarios efficiently. For instance, in Python’s SQLAlchemy:
from sqlalchemy import create_engine, MetaData, Table, select
engine = create_engine('sqlite:///example.db')
metadata = MetaData(bind=engine)
table = Table('table_name', metadata, autoload=True)
columns = [c for c in table.columns if c.name != 'unwanted_column']
query = select(columns)
Result = engine.execute(query)
Example Code
Here’s an example of selecting all columns except one using dynamic SQL in SQL Server:
DECLARE @columns NVARCHAR(MAX);
SELECT @columns = STRING_AGG(column_name, ', ')
FROM information_schema.columns
WHERE table_name = 'your_table_name' AND column_name != 'unwanted_column';
DECLARE @sql NVARCHAR(MAX);
SET @sql = 'SELECT ' + @columns + ' FROM your_table_name';
EXEC sp_executesql @sql;
Frequently Asked Questions
Is there a SQL standard way to exclude a column in a SELECT statement?
No, SQL does not provide a standard way to exclude a specific column. You need to list the columns you want to include or use dynamic SQL.
Can I use a wildcard to exclude columns in SQL?
SQL does not support wildcards for excluding columns directly. You need to use techniques such as dynamic SQL or views.
What are the performance implications of excluding a column?
Excluding large columns like BLOBs or CLOBs can improve query performance by reducing the amount of data transferred and processed.
Conclusion
Selecting all columns except one in SQL can be achieved through various methods, depending on the complexity and requirements of your database schema. Explicit column names are suitable for small tables, while dynamic SQL and views are better for larger, more complex schemas. By understanding these methods, you can optimize your SQL queries and manage your database more efficiently.