How to Reset Index in Pandas | Complete Guide
Pandas is a powerful Python library for data manipulation and analysis. One common task is resetting the index of a DataFrame. Resetting the index can be useful when you’ve made modifications to your DataFrame that have disrupted the original indexing, or when you need to convert a multi-index DataFrame into a flat single-index DataFrame. This article will explore various methods to reset the index in Pandas, with practical examples for each method.
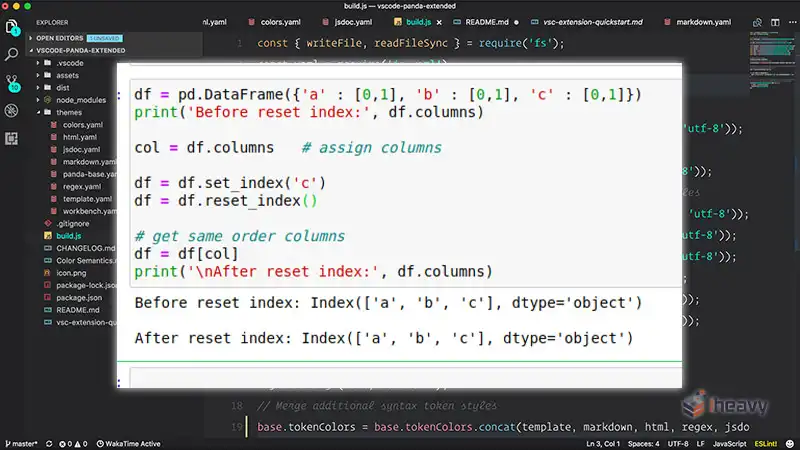
Methods to Reset Index in Pandas
Using the reset_index() Method
The reset_index() method is the most straightforward way to reset the index of a DataFrame. By default, this method creates a new index and moves the old index into a new column.
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
df.set_index('A', inplace=True)
print("Original DataFrame:")
print(df)
# Resetting the index
df_reset = df.reset_index()
print("\nDataFrame after resetting index:")
print(df_reset)
Dropping the Old Index
You can drop the old index entirely by passing the drop=True argument to reset_index(). This is useful when you do not need the old index as a column in your DataFrame.
# Resetting the index and dropping the old index
df_reset = df.reset_index(drop=True)
print("\nDataFrame after resetting index and dropping old index:")
print(df_reset)
Resetting Index of a Multi-Index DataFrame
For a DataFrame with a multi-level index, reset_index() can be used to flatten the index. By default, all levels are reset, but you can specify which levels to reset using the level parameter.
# Sample Multi-Index DataFrame
arrays = [['A', 'A', 'B', 'B'], [1, 2, 1, 2]]
index = pd.MultiIndex.from_arrays(arrays, names=('Letters', 'Numbers'))
df_multi = pd.DataFrame({'Values': [10, 20, 30, 40]}, index=index)
print("Original Multi-Index DataFrame:")
print(df_multi)
# Resetting the multi-level index
df_multi_reset = df_multi.reset_index()
print("\nMulti-Index DataFrame after resetting index:")
print(df_multi_reset)
Resetting Index in Place
If you want to reset the index without creating a new DataFrame, you can use the inplace=True parameter. This modifies the original DataFrame directly.
# Resetting the index in place
df.reset_index(inplace=True)
print("\nDataFrame after resetting index in place:")
print(df)
Best Practices to reset index in pandas
Understand Your Data
Before resetting the index, ensure you understand how the current index affects your data.
Drop Unnecessary Indices
Use drop=True if you do not need the old index, to keep your DataFrame clean and manageable.
In-Place Operations
Use inplace=True for efficiency, especially with large DataFrames, to avoid creating unnecessary copies.
Frequently Asked Questions
How do I reset the index of a DataFrame and drop the old index?
You can reset the index and drop the old index by using the reset_index() method with the drop=True parameter.
df_reset = df.reset_index(drop=True)
Can I reset only specific levels of a multi-index DataFrame?
Yes, you can specify which levels to reset by using the level parameter in the reset_index() method.
df_multi_reset = df_multi.reset_index(level='Letters')
What happens to the original DataFrame when I use reset_index()?
By default, reset_index() returns a new DataFrame and leaves the original DataFrame unchanged. To modify the original DataFrame, use inplace=True.
df.reset_index(inplace=True)
How can I reset the index without adding the old index as a column?
Use the drop=True parameter in the reset_index() method to reset the index without adding the old index as a column.
df_reset = df.reset_index(drop=True)
Is there a way to reset the index only if it’s not already the default integer index?
You can check if the index is default using df.index.is_integer() before resetting it.
if not df.index.is_integer():
df.reset_index(inplace=True)
Conclusion
Resetting the index of a DataFrame is a common and useful operation in Pandas. Whether you need to flatten a multi-index DataFrame, drop the old index, or simply reset the index to the default integer index, Pandas provides flexible methods to achieve this. Understanding these methods and when to use them will help you manage your data more effectively and keep your DataFrames well-organized.