How to Fix java.lang.NullPointerException | [Answered]
The java.lang.NullPointerException is one of the most common exceptions encountered by Java developers. It occurs when an application attempts to use an object reference that has not been initialized (i.e., it points to null). This article will explain the causes of this exception and provide strategies to identify and fix it effectively.
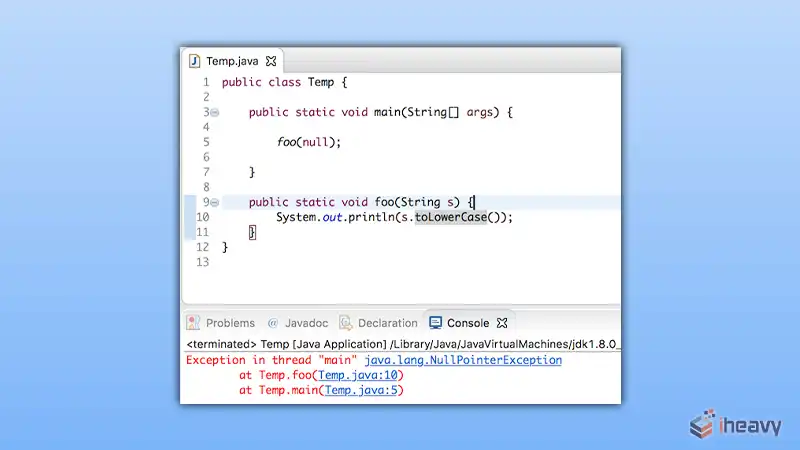
Understanding the java.lang.NullPointerException
A NullPointerException is thrown when an application tries to:
Call the instance method of a null object.
Access or modify the field of a null object.
Take the length of a null array.
Access or modify the slots of a null array.
Throw a null as if it were a Throwable value.
Potential Causes of java.lang.NullPointerException
Uninitialized Object
String str = null;
System.out.println(str.length()); // Throws NullPointerException
Missing new Keyword
Person person;
person = new Person(); // Correct way
person.name = "John"; // Throws NullPointerException if `new Person()` is missing
Returning Null from Methods
public String getName() {
return null; // Method returns null
}
public void printName() {
System.out.println(getName().length()); // Throws NullPointerException
}
Identifying the Source of the Exception
To effectively fix a NullPointerException, it’s crucial to identify its source. Here are some strategies:
Examine Stack Trace
The stack trace provides the exact line of code where the exception occurred.
Debugging
Use debugging tools to step through the code and inspect variable values.
Add Null Checks
Before accessing objects or calling methods, add checks to ensure they are not null.
Example Code
Null Check Example
public class Example {
public static void main(String[] args) {
String str = null;
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("String is null");
}
}
}
Using Optional to Avoid NullPointerException
Java 8 introduced the Optional class to handle null values more gracefully.
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String str = null;
Optional<String> optionalStr = Optional.ofNullable(str);
optionalStr.ifPresent(s -> System.out.println(s.length()));
}
}
Best Practices to Prevent NullPointerException
Initialize Variables
Always initialize variables, preferably at the time of declaration.
Use Default Values
Assign default values to variables that can be null.
Avoid Returning Null
Return empty collections or optional instead of null.
Leverage Annotations
Use annotations like @NonNull and @Nullable to indicate nullability.
Frequently Asked Questions
How can I find where a NullPointerException occurs in my code?
Examine the stack trace provided in the exception message. It points to the exact line of code where the NullPointerException occurred.
Can a NullPointerException occur in a try-catch block?
Yes, a NullPointerException can occur in a try block. You can catch and handle it within the catch block.
Are there any tools to help prevent NullPointerException?
Yes, static code analysis tools like FindBugs, PMD, and SonarQube can help detect potential null pointer issues in your code.
Conclusion
The java.lang.NullPointerException is a common but avoidable exception in Java. By understanding its causes and employing best practices such as null checks, proper initialization, and using the Optional class, developers can significantly reduce the occurrence of this exception. Implementing these strategies ensures more robust and error-resistant code.