How to Extract Values from a Nested JSON Field in SQL Server
SQL Server provides robust support for JSON, making it easier to store, query, and manipulate JSON data within your database. One common task is extracting values from nested JSON fields. This article will guide you through the process of extracting values from a nested JSON field in SQL Server, complete with example queries and explanations.
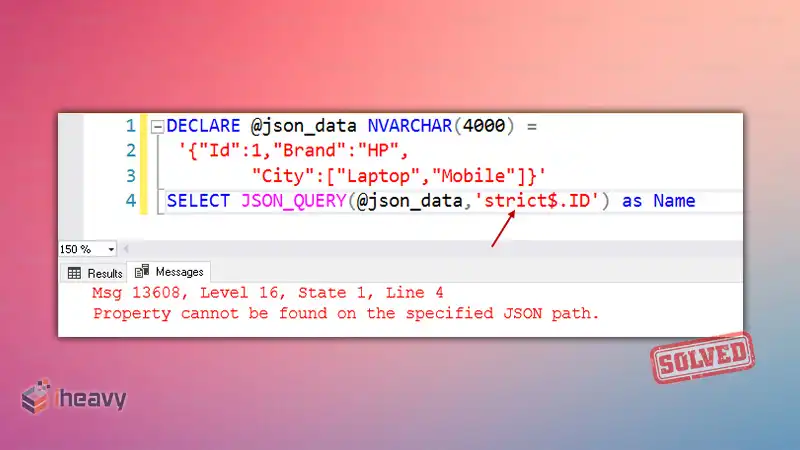
Understanding JSON Functions in SQL Server
SQL Server includes several built-in functions to work with JSON data:
OPENJSON
Parses JSON text and returns objects and properties as rows.
JSON_VALUE
Extracts a scalar value from a JSON string.
JSON_QUERY
Extracts an object or an array from a JSON string.
JSON_MODIFY
Updates the value of a property in a JSON string and returns the updated JSON string.
Example JSON Structure
Consider the following JSON structure stored in a column named JsonData in a table called JsonTable:
{
"id": 1,
"name": "John Doe",
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"postalCode": "12345"
},
"orders": [
{
"orderId": 101,
"product": "Laptop",
"quantity": 1
},
{
"orderId": 102,
"product": "Mouse",
"quantity": 2
}
]
}
Extracting Values from Nested JSON Fields
Extracting Scalar Values
To extract scalar values from a JSON field, use the JSON_VALUE function. For example, to extract the city from the address field:
sql
Copy code
SELECT JSON_VALUE(JsonData, '$.address.city') AS City
FROM JsonTable;
Extracting Objects and Arrays
To extract an entire object or array, use the JSON_QUERY function. For example, to extract the address object:
SELECT JSON_QUERY(JsonData, '$.address') AS Address
FROM JsonTable;
Extracting Values from Nested Arrays
To extract values from nested arrays, use the OPENJSON function. For example, to extract all order details:
SELECT j.[key] AS OrderIndex,
JSON_VALUE(j.value, '$.orderId') AS OrderId,
JSON_VALUE(j.value, '$.product') AS Product,
JSON_VALUE(j.value, '$.quantity') AS Quantity
FROM JsonTable
CROSS APPLY OPENJSON(JsonData, '$.orders') AS j;
Putting It All Together
Combining these functions, you can extract and manipulate nested JSON data effectively. Here is an example query that extracts multiple fields from the JSON structure:
SELECT JSON_VALUE(JsonData, '$.name') AS Name,
JSON_VALUE(JsonData, '$.address.city') AS City,
JSON_VALUE(JsonData, '$.address.state') AS State,
JSON_QUERY(JsonData, '$.orders') AS Orders
FROM JsonTable;
Frequently Asked Questions
Can I update a nested JSON field in SQL Server?
Yes, you can use the JSON_MODIFY function to update a nested JSON field. For example, to update the city in the address field:
UPDATE JsonTable
SET JsonData = JSON_MODIFY(JsonData, '$.address.city', 'NewCity')
WHERE JSON_VALUE(JsonData, '$.id') = 1;
How do I handle missing or null values in JSON?
Use the ISNULL or COALESCE functions to handle missing or null values. For example, to handle a missing state value:
SELECT COALESCE(JSON_VALUE(JsonData, '$.address.state'), 'Unknown') AS State
FROM JsonTable;
Can I index JSON fields in SQL Server?
Yes, you can create computed columns based on JSON values and index those columns to improve query performance. For example:
ALTER TABLE JsonTable
ADD City AS JSON_VALUE(JsonData, '$.address.city');
CREATE INDEX idx_city ON JsonTable(City);
Conclusion
Extracting values from nested JSON fields in SQL Server is straightforward with the built-in JSON functions. By using JSON_VALUE, JSON_QUERY, and OPENJSON, you can efficiently query and manipulate JSON data stored in your database. Understanding these functions and their applications will enable you to work effectively with JSON data in SQL Server.