Error – How to Escape Single Quote in SQL
Dealing with strings in SQL can sometimes be tricky, especially when it comes to including single quotes within the strings. If not handled properly, single quotes can cause SQL syntax errors or even lead to SQL injection vulnerabilities. This article explores how to escape single quotes in SQL to ensure your queries run smoothly and securely.
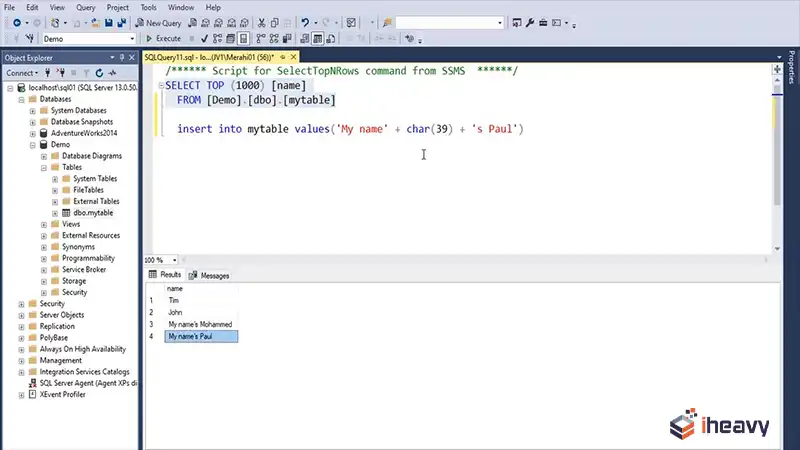
Understanding the Single Quote Problem
In SQL, single quotes are used to delimit string literals. For instance, the string O’Reilly would be written as ‘O’Reilly’. However, SQL interprets the single quote inside the string as the end of the string, leading to a syntax error.
Example Error Message
Sql
SELECT * FROM authors WHERE last_name = 'O'Reilly';
This will result in an error because SQL does not know how to handle the single quote within the string.
Methods to Escape Single Quotes in SQL
Using Two Single Quotes
The most common way to escape a single quote in SQL is by using two single quotes. This tells SQL to treat the two single quotes as a literal single quote.
Sql
SELECT * FROM authors WHERE last_name = 'O''Reilly';
Using Backslash (Not universally supported)
Some SQL databases, like MySQL, support using a backslash before the single quote to escape it. However, this is not a standard SQL practice and might not work in all SQL databases.
Sql
SELECT * FROM authors WHERE last_name = 'O\'Reilly';
Using Parameterized Queries
Using parameterized queries or prepared statements is a best practice for escaping single quotes. This approach not only escapes special characters but also protects against SQL injection attacks.
Python with SQLAlchemy
from sqlalchemy import create_engine, text
engine = create_engine('postgresql://user:password@localhost/mydatabase')
query = text("SELECT * FROM authors WHERE last
Python
from sqlalchemy import create_engine, text
engine = create_engine('postgresql://user:password@localhost/mydatabase')
query = text("SELECT * FROM authors WHERE last_name = :last_name")
result = engine.execute(query, last_name="O'Reilly")
for row in result:
print(row)
Using SQL Functions
Some SQL databases provide specific functions to handle single quotes within strings. For instance, PostgreSQL offers the quote_literal function.
Example in PostgreSQL
Sql
SELECT * FROM authors WHERE last_name = quote_literal('O'Reilly');
Practical Example
Let’s say you have a table named customers and you need to insert a customer with a last name that contains a single quote.
Incorrect Approach
Sql
INSERT INTO customers (first_name, last_name) VALUES ('John', 'O'Reilly');
This will produce a syntax error.
Correct Approach
Using Two Single Quotes
Sql
INSERT INTO customers (first_name, last_name) VALUES ('John', 'O''Reilly');
Using a Parameterized Query in Python
Python
from sqlalchemy import create_engine, text
engine = create_engine('postgresql://user:password@localhost/mydatabase')
query = text("INSERT INTO customers (first_name, last_name) VALUES (:first_name, :last_name)")
engine.execute(query, first_name='John', last_name="O'Reilly")
Frequently Asked Questions
Why should I use parameterized queries to handle single quotes?
Parameterized queries not only handle special characters like single quotes automatically but also protect your database from SQL injection attacks, making your application more secure.
Can I use backslashes to escape single quotes in all SQL databases?
No, not all SQL databases support using backslashes to escape single quotes. This method is primarily supported by MySQL but not by databases like PostgreSQL or SQL Server. It’s safer to use two single quotes or parameterized queries.
What should I do if my database doesn’t support backslashes for escaping?
If your database does not support backslashes for escaping single quotes, you should use two single quotes or parameterized queries to handle single quotes within strings.
Conclusion
Handling single quotes in SQL is crucial for writing correct and secure SQL queries. The most common methods include using two single quotes or parameterized queries. While some databases support using backslashes, this is not a universal solution. By understanding and implementing these methods, you can ensure your SQL queries handle single quotes effectively and securely, avoiding syntax errors and protecting against SQL injection vulnerabilities.