How to Drop a Constraint in SQL | Easy Methods
In SQL, constraints are rules applied to table columns to enforce data integrity and ensure the accuracy and reliability of the data within the database. However, there are situations where you may need to drop a constraint, such as when modifying the schema, fixing data issues, or changing business requirements.
This article will guide you through the process of dropping constraints in SQL, covering various types of constraints and providing example code snippets.
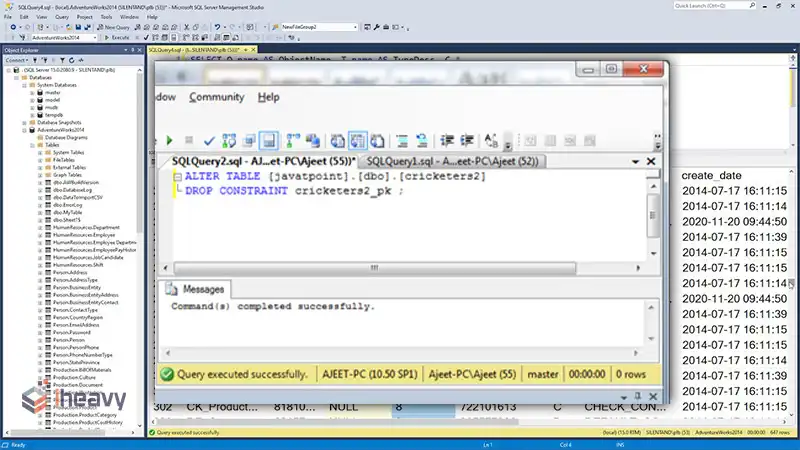
Types of Constraints
Before diving into how to drop constraints, it’s essential to understand the different types of constraints that can be applied to SQL tables:
Primary Key Constraint
Ensures that a column (or combination of columns) uniquely identifies each row in the table.
Foreign Key Constraint
Ensures that the value in a column (or combination of columns) matches values in another table, maintaining referential integrity.
Unique Constraint
Ensures that all values in a column (or combination of columns) are unique.
Check Constraint
Ensures that all values in a column satisfy a specific condition.
Default Constraint
Provides a default value for a column when no value is specified.
Not Null Constraint
Ensures that a column cannot have NULL values.
Dropping Constraints
Dropping a Primary Key Constraint
To drop a primary key constraint, you typically use the ALTER TABLE statement followed by DROP CONSTRAINT. The exact syntax can vary depending on the SQL database you are using.
ALTER TABLE table_name
DROP CONSTRAINT constraint_name;
For example, in SQL Server:
ALTER TABLE Employees
DROP CONSTRAINT PK_Employees;
In MySQL:
ALTER TABLE Employees
DROP PRIMARY KEY;
Dropping a Foreign Key Constraint
To drop a foreign key constraint, you need to know the name of the constraint.
ALTER TABLE table_name
DROP CONSTRAINT constraint_name;
For example, in SQL Server:
ALTER TABLE Orders
DROP CONSTRAINT FK_Orders_Customers;
In MySQL:
ALTER TABLE Orders
DROP FOREIGN KEY FK_Orders_Customers;
Dropping a Unique Constraint
Dropping a unique constraint involves the same syntax as dropping other constraints.
ALTER TABLE table_name
DROP CONSTRAINT constraint_name;
For example, in SQL Server:
ALTER TABLE Products
DROP CONSTRAINT UQ_Products_Code;
In MySQL:
ALTER TABLE Products
DROP INDEX UQ_Products_Code;
Dropping a Check Constraint
To drop a check constraint, use the ALTER TABLE statement followed by DROP CONSTRAINT.
ALTER TABLE table_name
DROP CONSTRAINT constraint_name;
For example, in SQL Server:
ALTER TABLE Employees
DROP CONSTRAINT CK_Employees_Age;
In MySQL:
ALTER TABLE Employees
DROP CHECK CK_Employees_Age;
Dropping a Default Constraint
Dropping a default constraint also uses the ALTER TABLE statement.
ALTER TABLE table_name
DROP CONSTRAINT constraint_name;
For example, in SQL Server:
ALTER TABLE Orders
DROP CONSTRAINT DF_Orders_OrderDate;
In MySQL:
ALTER TABLE Orders
ALTER COLUMN OrderDate DROP DEFAULT;
Dropping a Not Null Constraint
To drop a not null constraint, you need to alter the column to allow NULL values.
ALTER TABLE table_name
ALTER COLUMN column_name DROP NOT NULL;
For example, in SQL Server:
ALTER TABLE Employees
ALTER COLUMN LastName NVARCHAR(50) NULL;
In MySQL:
ALTER TABLE Employees
MODIFY COLUMN LastName VARCHAR(50) NULL;
Frequently Asked Questions
How do I find the name of a constraint in SQL?
You can query the system catalog or information schema tables to find the names of constraints. For example, in SQL Server:
SELECT name
FROM sys.key_constraints
WHERE type = 'PK' AND parent_object_id = OBJECT_ID('Employees');
In MySQL:
SELECT constraint_name
FROM information_schema.table_constraints
WHERE table_name = 'Employees';
Can I drop multiple constraints at once?
No, you need to drop each constraint individually using separate ALTER TABLE statements.
What happens to the data when I drop a constraint?
Dropping a constraint does not affect the existing data in the table. However, it removes the rule that enforces data integrity, so future data modifications may violate the integrity that the constraint was enforcing.
Conclusion
Dropping constraints in SQL is a common task when modifying the database schema or adapting to new requirements. This article has provided a comprehensive guide on how to drop various types of constraints, complete with example code for different SQL databases. Understanding how to manage constraints effectively ensures that your database maintains data integrity while allowing for necessary changes and updates.