How to Change Column Name in Pandas
Pandas is a powerful library for data manipulation and analysis in Python. One common operation is renaming columns in a DataFrame. This can be useful for making your data more readable, aligning it with certain conventions, or preparing it for further processing. In this article, we will explore various methods to change column names in Pandas and provide practical examples for each method.
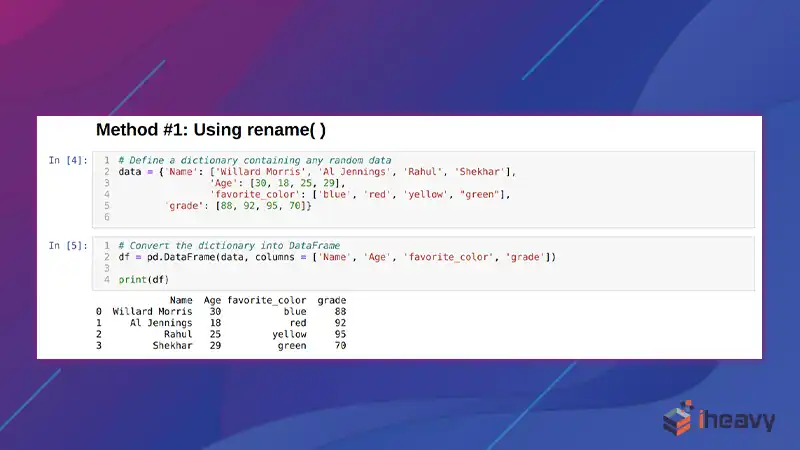
Methods to Change Column Names in Pandas
Using the rename() Method
The rename() method allows you to rename specific columns by passing a dictionary where keys are the old column names and values are the new column names.
import pandas as pd
# Sample DataFrame
data = {'A': [1, 2, 3], 'B': [4, 5, 6]}
df = pd.DataFrame(data)
# Renaming columns using rename()
df_renamed = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
print(df_renamed)
Using the columns Attribute
You can directly modify the columns attribute of a DataFrame to rename all columns. This is useful when you want to rename multiple columns at once.
# Renaming columns using columns attribute
df.columns = ['Column1', 'Column2']
print(df)
Renaming Columns with a List
If you have a list of new column names, you can assign it directly to the columns attribute.
# List of new column names
new_columns = ['Column1', 'Column2']
# Renaming columns using a list
df.columns = new_columns
print(df)
Using the set_axis() Method
The set_axis() method can be used to set new column names. This method can also be used to rename index labels.
# Renaming columns using set_axis()
df = df.set_axis(['Column1', 'Column2'], axis=1, inplace=False)
print(df)
Using a Function with rename()
You can pass a function to rename() to apply a transformation to the column names. This is useful for applying a consistent change to all column names, such as making them uppercase.
# Function to convert column names to uppercase
def to_uppercase(column_name):
return column_name.upper()
# Renaming columns using a function with rename()
df_renamed = df.rename(columns=to_uppercase)
print(df_renamed)
Best Practices
Clear and Consistent Naming
Use clear and consistent column names to improve the readability and maintainability of your DataFrame.
Avoid Conflicts
Ensure that the new column names do not conflict with existing column names to prevent confusion and errors.
Document Changes
Document any changes to column names, especially in collaborative projects, to keep track of modifications.
Frequently Asked Questions
How do I rename multiple columns at once?
You can use the rename() method with a dictionary or assign a list of new column names to the columns attribute.
# Renaming multiple columns
df_renamed = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
Can I rename a single column in a large DataFrame?
Yes, you can use the rename() method to rename a single column without affecting the other columns.
# Renaming a single column
df_renamed = df.rename(columns={'A': 'Column1'})
Is it possible to rename columns based on their position?
Pandas does not provide a direct method to rename columns based on their position. However, you can use the columns attribute along with list indexing to achieve this.
# Renaming columns based on position
new_columns = list(df.columns)
new_columns[0] = 'Column1'
df.columns = new_columns
How can I convert all column names to lowercase?
You can use the rename() method with a function to convert all column names to lowercase.
# Converting all column names to lowercase
df_renamed = df.rename(columns=str.lower)
Can I revert back to the original column names?
If you have stored the original column names, you can reassign them to the columns attribute to revert back.
# Original column names
original_columns = ['A', 'B']
# Reverting to original column names
df.columns = original_columns
Conclusion
Renaming columns in Pandas is a straightforward process that can be accomplished using various methods such as rename(), the columns attribute, and set_axis(). By understanding these methods, you can efficiently manage and manipulate your DataFrame’s column names to suit your data processing needs. Whether you are preparing data for analysis or aligning it with specific conventions, these techniques will help you maintain clear and consistent column names.