Troubleshooting ‘Fail to Update State React’
React’s state management is a critical part of creating dynamic, interactive web applications. However, many developers—especially those new to React—encounter issues where the state fails to update as expected.
Understanding the root causes of these problems and how to resolve them is key to becoming proficient with React. And this article can be your friend in need regarding that aspect. So, let’s begin.
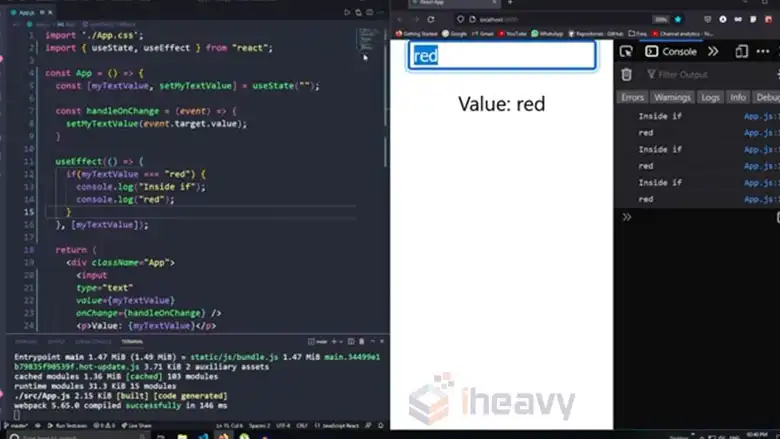
Why Is React Not Updating on State Change?
Here are top 5 reasons why updating state can become an issue in React along with workarounds to avoid this problem.
1. State Mutations
One of the most common mistakes that lead to state update failures is directly mutating the state. In React, state should be treated as immutable. This means that instead of modifying the state directly, you should create a new object or array and update the state with this new value.
Example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
count++; // Direct mutation (This won't trigger a re-render)
setCount(count); // State update might not work as expected
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
Here, count++ directly mutates the state. While setCount(count) is called, it might not trigger a re-render because React does not detect that the state has changed significantly.
Corrected Version:
const increment = () => {
setCount(prevCount => prevCount + 1); // Correct approach: using a new state value
};
By using setCount(prevCount => prevCount + 1), you ensure that React sees the state change, which triggers a re-render.
2. Asynchronous Nature of setState
React’s setState (or useState in function components) is asynchronous. This means that if you try to immediately use the state after setting it, you might not get the updated value.
Example:
const handleClick = () => {
setCount(count + 1);
console.log(count); // Logs the old value, not the updated one
};
console.log(count) logs the old state because setState hasn’t completed yet.
Corrected Version:
const handleClick = () => {
setCount(prevCount => {
console.log(prevCount + 1); // Correctly logs the expected new value
return prevCount + 1;
});
};
By using the callback form of setCount, you get the most recent state, ensuring correct behavior.
3. Batch Updates in React
React may batch multiple state updates into a single re-render for performance reasons. However, this can lead to unexpected results if you are not aware of it.
Example:
const handleMultipleUpdates = () => {
setCount(count + 1);
setCount(count + 2);
setCount(count + 3);
console.log(count); // This might not give you the expected value
};
Due to batching, count might not update as expected because React batches the state updates.
Corrected Version:
const handleMultipleUpdates = () => {
setCount(prevCount => prevCount + 1);
setCount(prevCount => prevCount + 2);
setCount(prevCount => prevCount + 3);
};
Using the functional form ensures that each update has access to the most recent state, preventing issues related to batching.
4. Incorrect Dependency Array in useEffect
When using the useEffect hook, the dependency array plays a critical role in determining when the effect runs. If you forget to include a state variable in the dependency array, the effect might not run when that state updates, leading to state inconsistencies.
import React, { useState, useEffect } from 'react';
function ExampleComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Effect ran');
}, []); // Empty array means this effect runs only on mount
const increment = () => setCount(count + 1);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
Here, the useEffect hook does not react to changes in count because count is not included in the dependency array.
Corrected Version:
useEffect(() => {
console.log('Effect ran, count is:', count);
}, [count]); // Now effect runs every time `count` changes
By including count in the dependency array, the effect now runs whenever count changes, ensuring that the component behaves as expected.
5. State Update Not Triggering Re-render
If a state update doesn’t trigger a re-render, it might be due to how the new state value compares to the old one. React uses shallow comparison to determine if a re-render is necessary.
Example:
const [name, setName] = useState({ firstName: 'John', lastName: 'Doe' });
const updateName = () => {
const newName = name;
newName.firstName = 'Jane';
setName(newName); // This might not trigger a re-render
};
Here, the newName object is the same reference as name, so React sees no difference and does not trigger a re-render.
Corrected Version:
const updateName = () => {
setName({ ...name, firstName: 'Jane' }); // Create a new object to trigger re-render
};
By creating a new object using the spread operator, you ensure React sees the state change, leading to the expected re-render.
Frequently Asked Questions
Why can’t we update state directly in React?
Altering the state directly can prevent React from recognizing changes. This means your component won’t update its display as it should, leading to unexpected behavior. These issues can be challenging to identify and fix.
Why is setState asynchronous?
setState is asynchronous because React batches multiple state updates to optimize performance. This asynchronous behavior allows React to minimize re-renders and improve application efficiency.
How do I ensure my state updates correctly when using previous state values?
Always use the functional form of setState (e.g., setState(prevState => prevState + 1)). This ensures that you work with the most recent state, avoiding issues related to asynchronous updates.
Conclusion
Understanding these common pitfalls and best practices in state management will help you avoid many of the issues that can arise when working with React. Mastering these techniques is a significant step towards building more reliable and efficient React applications.