@data vs @value: Key Differences and Usage
When developing modern web applications, particularly with frameworks like Blazor or libraries like Vue.js and Angular, developers frequently encounter directives and attributes that help bind data to the UI. Two common concepts are @data and @value, which are used to bind information to HTML elements.
While they may seem similar, they have distinct purposes and implications for how data is managed and displayed. Let’s break down their roles and clarify how they differ.
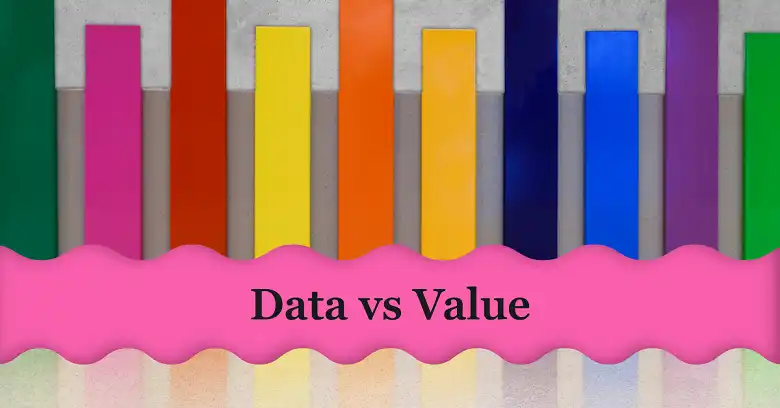
@data: Dynamic Attribute Binding
@data is a dynamic binding mechanism that can be commonly seen in frameworks where attributes need to be attached to HTML elements dynamically. It works as a directive that binds data from the component or script directly into a custom or standard attribute on an HTML element.
Purpose:
- Attaches dynamic data as an attribute to the element.
- Often used when the attribute name itself needs to change dynamically or when you need custom data attributes for a DOM element.
- Primarily used in scenarios where the element must carry metadata or custom information which is later accessed using JavaScript or frameworks.
<!-- Using @data for dynamic attribute binding -->
<button @data-type="buttonType">Click me</button>
In the above example, the buttonType value is dynamically bound to the data-type attribute. You might use this approach in cases where the attribute name or value might change depending on the state of your application.
Key Points:
- Facilitates metadata storage within the element for later use.
- Does not directly impact user interaction but is useful for logic handling behind the scenes.
- Common in scenarios requiring flexible attribute management.
@value: Data Binding for Form Inputs
On the other hand, @value is directly tied to the value of an input element. It is a standard way to bind or set the value of form elements such as text boxes, dropdowns, and checkboxes. This attribute binds the component or model value to the form element and ensures two-way synchronization between the UI and the underlying data model.
Purpose:
- Primarily used for form inputs to bind user input directly to a data property.
- Ensures that as the user interacts with the form, the underlying data is updated in real time.
- Essential in reactive frameworks like Vue.js, Angular, and Blazor for keeping the model and UI in sync.
<!-- Using @value for two-way data binding -->
<input @value="userInput" type="text" />
In this example, the userInput data property is bound to the input field, so when the user types into the text box, the userInput value is updated accordingly.
Key Points:
- Enables real-time synchronization between user input and data.
- Essential for handling forms and capturing user data in applications.
- Plays a key role in interactive applications where the UI reacts to user changes.
What is the difference between @data and @value?
The following table shows the key differences between @data and @value, providing clear examples to illustrate their usage.
Aspect | @data | @value |
Primary Use | Attaching metadata or custom attributes | Binding form inputs to data models |
Common Use Cases | Dynamic attributes, metadata storage | Handling user input, form submissions |
Direct Impact on UI | Indirect (metadata, logic handling) | Direct (updates UI based on user input) |
Two-way Data Binding | No | Yes |
Element Types | All HTML elements | Form elements (input, select, textarea) |
Framework Support | Used in custom directive-based setups | Standard in frameworks like Vue, Blazor |
@data vs @value | When to Use Each
Use @data when you need to bind dynamic or custom attributes that store metadata or for scenarios requiring flexible attribute names or values. This can be especially useful when attaching data-* attributes, which serve as invisible markers that can later be accessed by JavaScript.
Use @value when working with form inputs where user interaction changes the state of the application. It is most appropriate for handling real-time data changes that need to be reflected in the UI, such as capturing user input in forms.
@Data vs @Value in Java/Spring
@data and @value can also be compared in the context of Java/Spring. Here’s more on that note.
@Data: Lombok’s Annotation for Simplified Code
In Java, particularly with Lombok, @Data is an annotation used to automatically generate boilerplate code like getters, setters, equals(), hashCode(), and toString() methods for a class. It’s a shorthand to make the code more readable and maintainable without needing to manually write these methods.
Purpose:
- Simplifies class definitions by generating common methods.
- Applied at the class level.
import lombok.Data;
@Data
public class User {
private String name;
private int age;
}
In the example above, Lombok’s @Data automatically generates getters and setters for the name and age fields, as well as equals(), hashCode(), and toString().
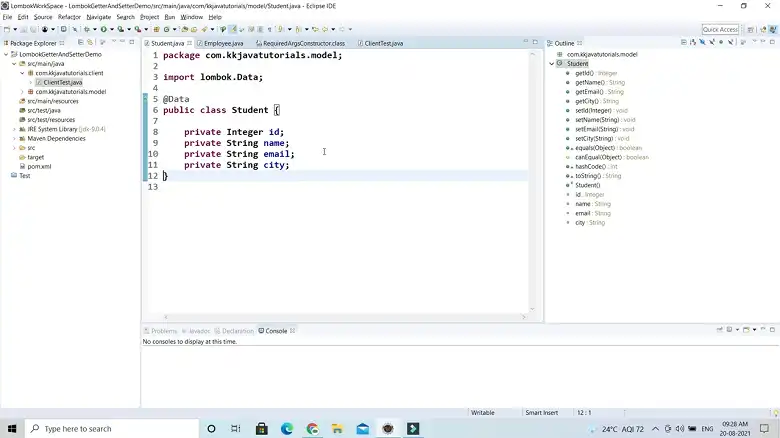
Key Points:
- Used to reduce boilerplate code.
- Affects the structure of the class by generating methods.
@Value: Immutable Data in Spring
In the context of Spring, @Value is an annotation used to inject values into variables from properties files, environment variables, or other sources of configuration. This annotation is particularly important for configuring beans with externalized values.
Purpose:
- Injects values from properties or configuration into Spring-managed beans.
- Used for application settings, constants, or environment-dependent values.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class Config {
@Value("${app.name}")
private String appName;
}
In this example, @Value(“${app.name}”) injects the value of app.name from a properties file into the appName field.
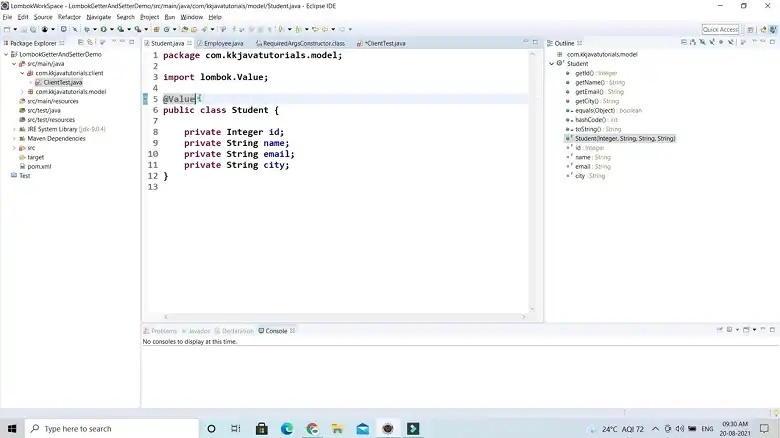
Key Points:
- Used to inject configuration data into Spring beans.
- Allows for external configuration of application settings.
Frequently Asked Questions
Can @data and @value be used together?
Yes, though they serve different purposes, you might use both within the same element for different reasons. For instance, you could use @data to bind a custom attribute for later retrieval and @value to capture user input.
Does @data support two-way binding?
No, @data is typically one-way binding where the attribute reflects a value from the underlying model or state. It does not automatically update back to the model like @value.
Conclusion
In conclusion, while @data and @value may appear similar at first glance, they serve distinct roles in data binding within modern web applications. Choosing the correct one depends on the context—whether you are dealing with form inputs that require real-time data updates or dynamic attribute bindings for logic and metadata storage.