Correlated Column Is Not Allowed in Predicate | Solved
The “correlated column is not allowed in predicate” error can be confusing for those not deeply familiar with SQL’s issues. But it essentially relates to the rules governing the use of subqueries and how columns from outer queries are referenced within them.
Let’s have a tour to what this error means, why it occurs, and how to resolve it.
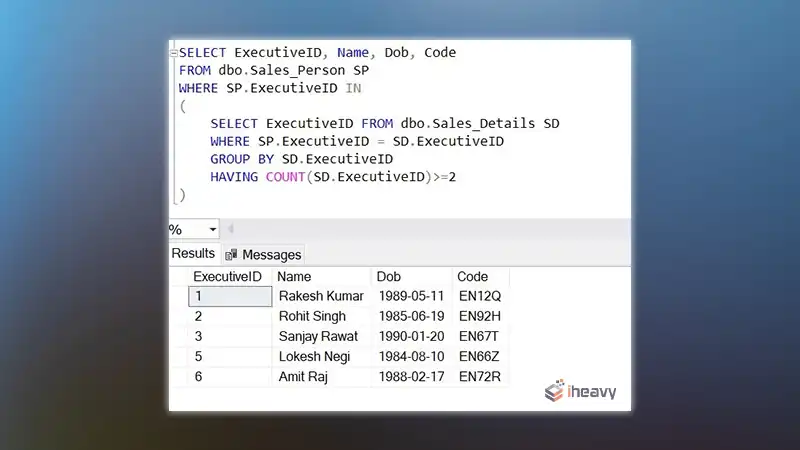
What is a Correlated Subquery in SQL?
A correlated subquery is a subquery that references columns from the outer query. Unlike a regular subquery, which is independent and can be executed on its own, a correlated subquery depends on the outer query for its values. It’s evaluated once for each row processed by the outer query.
Example of a Correlated Subquery:
SELECT employee_id, name
FROM employees e
WHERE salary > (SELECT AVG(salary)
FROM employees
WHERE department_id = e.department_id);
In this example, the subquery relies on the department_id from the outer query (e.department_id). It calculates the average salary for the department of each employee in the outer query.
What Causes the “Correlated Column is Not Allowed in Predicate” Error?
This error typically occurs when the SQL syntax rules prohibit the use of a correlated column in certain parts of a query. Predicates, which are conditions in the WHERE, HAVING, or ON clauses, sometimes restrict correlated references to ensure logical and executional integrity.
Common Scenarios
- Subqueries in SELECT Clause: If a correlated subquery is used improperly within the SELECT clause, the database might throw this error.
- JOIN Conditions: Using correlated columns in the ON clause of a JOIN can also lead to this error.
- Derived Tables or Common Table Expressions (CTEs): When these are involved, referencing outer query columns might be restricted.
Resolving the Error
To resolve the “correlated column is not allowed in predicate” error, consider the following approaches:
- Restructure the Query: Sometimes, rewriting the query to avoid the correlated subquery can resolve the issue.
- Use Alternative Joins or Subqueries: Depending on the context, an INNER JOIN or LEFT JOIN might serve the same purpose as a subquery.
- Nested Subqueries: Ensure that subqueries are correctly nested and that their scopes are properly defined.
How to Optimize Subquery in SQL Server?
To optimize your subqueries and make them faster and clearer, consider using EXISTS or NOT EXISTS instead of IN or NOT IN when checking for a value in a result set. Joins can also be more efficient than subqueries, particularly when combining data from multiple tables.
Consider the following erroneous query:
SELECT e1.employee_id, e1.name
FROM employees e1
WHERE EXISTS (SELECT 1
FROM employees e2
WHERE e1.department_id = e2.department_id
AND e2.salary > e1.salary);
If this causes an error due to correlated columns in the predicate, restructuring it might help:
SELECT e1.employee_id, e1.name
FROM employees e1
JOIN employees e2 ON e1.department_id = e2.department_id
WHERE e2.salary > e1.salary;
Frequently Asked Questions
How to avoid correlated subquery in SQL Server?
It’s generally recommended to use JOIN operations instead of correlated subqueries when solving data queries, whenever feasible. Correlated subqueries should be reserved for scenarios where they are essential, such as handling negative data questions or other situations where they are the sole method to obtain the required information.
How do you handle when subquery returns more than one row?
The initial step involves rewriting the query such that the subquery returns only one row.
Conclusion
Resolving the “correlated column is not allowed in predicate” error in SQL queries requires understanding its causes and adopting appropriate solutions. By rethinking query structures, using JOIN operations effectively, and minimizing reliance on correlated subqueries, you can achieve efficient and error-free database operations. These practices will not only improve query performance but also enhance overall code clarity and maintainability.