Converting Pandas DataFrame to Spark DataFrame with Schema
Both Pandas and Apache Spark are widely used tools. Pandas is preferred for small to medium-sized data manipulation due to its simplicity and efficiency. In contrast, Spark excels at handling large-scale data processing and distributed computing.
Converting a Pandas DataFrame to a Spark DataFrame is a common task, especially when scaling from local data analysis to distributed data processing. This article will teach you how to perform this conversion and how to define a schema for the Spark DataFrame.
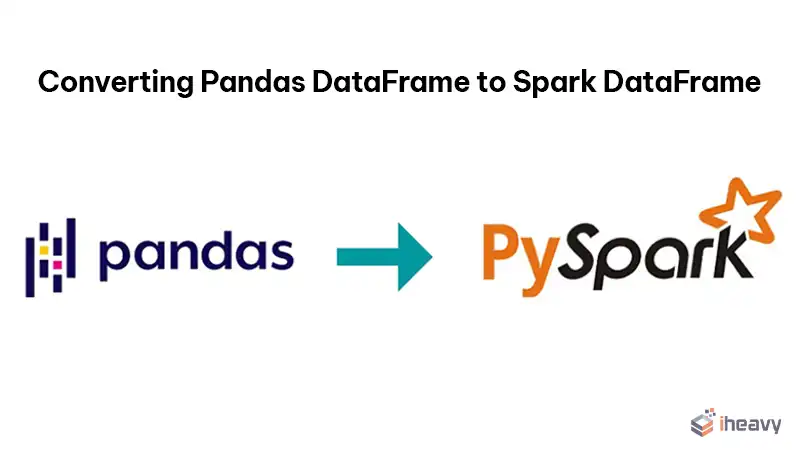
Why Convert from Pandas to Spark?
While Pandas is ideal for data manipulation on a single machine, it struggles with large datasets that exceed the memory capacity of a single machine. Apache Spark, with its distributed computing capabilities, can handle large datasets across a cluster of machines. Therefore, converting a Pandas DataFrame to a Spark DataFrame enables the leveraging of Spark’s distributed processing power.
How to Convert Pandas DataFrame to PySpark DataFrame with Schema?
Before proceeding, ensure that you have both Pandas and PySpark installed. You can install them using pip:
pip install pandas pyspark
Step 1: Create a Pandas DataFrame
First, let’s create a simple Pandas DataFrame:
import pandas as pd
# Sample data
data = {
'Name': ['Alice', 'Bob', 'Catherine'],
'Age': [25, 30, 22],
'City': ['New York', 'Los Angeles', 'Chicago']
}
# Creating Pandas DataFrame
pandas_df = pd.DataFrame(data)
print("Pandas DataFrame:\n", pandas_df)
Step 2: Initialize a SparkSession
Next, initialize a SparkSession. This is the entry point for working with Spark:
from pyspark.sql import SparkSession
# Initialize SparkSession
spark = SparkSession.builder \
.appName("Pandas to Spark DataFrame Conversion") \
.getOrCreate()
Step 3: Define the Schema (Optional)
Defining a schema ensures that the data types are explicitly set, which can be useful for data validation and performance optimization. You can define a schema using the StructType and StructField classes from pyspark.sql.types:
from pyspark.sql.types import StructType, StructField, StringType, IntegerType
# Define the schema
schema = StructType([
StructField('Name', StringType(), True),
StructField('Age', IntegerType(), True),
StructField('City', StringType(), True)
])
Step 4: Convert the Pandas DataFrame to a Spark DataFrame
Finally, convert the Pandas DataFrame to a Spark DataFrame using the createDataFrame method, optionally passing the schema:
# Convert Pandas DataFrame to Spark DataFrame
spark_df = spark.createDataFrame(pandas_df, schema=schema)
print("Spark DataFrame:\n")
spark_df.show()
Complete Code Example
Here is the complete code to convert a Pandas DataFrame to a Spark DataFrame with a schema:
import pandas as pd
from pyspark.sql import SparkSession
from pyspark.sql.types import StructType, StructField, StringType, IntegerType
# Sample data
data = {
'Name': ['Alice', 'Bob', 'Catherine'],
'Age': [25, 30, 22],
'City': ['New York', 'Los Angeles', 'Chicago']
}
# Creating Pandas DataFrame
pandas_df = pd.DataFrame(data)
# Initialize SparkSession
spark = SparkSession.builder \
.appName("Pandas to Spark DataFrame Conversion") \
.getOrCreate()
# Define the schema
schema = StructType([
StructField('Name', StringType(), True),
StructField('Age', IntegerType(), True),
StructField('City', StringType(), True)
])
# Convert Pandas DataFrame to Spark DataFrame
spark_df = spark.createDataFrame(pandas_df, schema=schema)
# Show the Spark DataFrame
spark_df.show()
Frequently Asked Questions
Can I use pandas on a Spark DataFrame?
Pandas-on-Spark DataFrames and Spark DataFrames can be used interchangeably for most purposes. However, it is important to note that when a pandas-on-Spark DataFrame is generated from a Spark DataFrame, a new default index is created.
Is Spark DataFrame faster than pandas DataFrame?
PySpark outperforms Pandas when handling large datasets. It utilizes the computing power of a machine cluster for parallel processing, which can greatly decrease processing times.
Conclusion
Converting a Pandas DataFrame to a Spark DataFrame is a straightforward process that can significantly enhance your data processing capabilities. This conversion allows you to scale your data analysis from a single machine to a distributed computing environment, leveraging the full power of Apache Spark.