Converting JSON to SQLite | A Comprehensive Guide
Working with data often involves transforming it from one format to another. JSON (JavaScript Object Notation) is a popular data interchange format due to its simplicity and readability. SQLite is a lightweight, self-contained SQL database engine.
Converting JSON data to an SQLite database can be useful for various applications, including data analysis, mobile applications, and local data storage. This article provides a detailed guide on how to convert JSON data to SQLite.
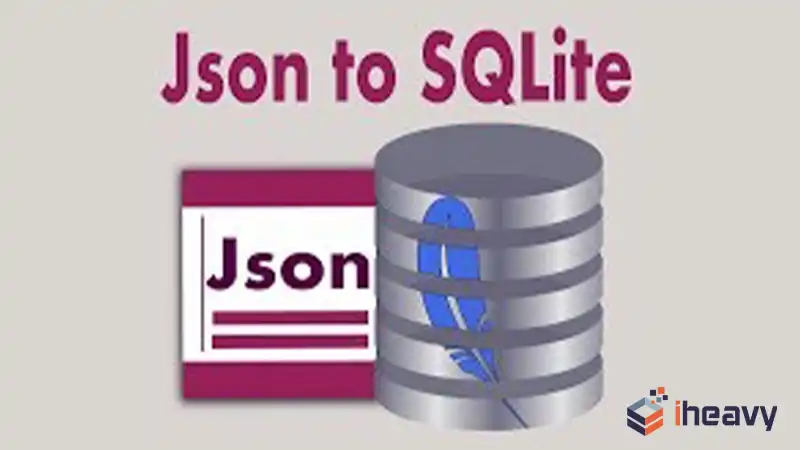
Understanding JSON and SQLite
JSON (JavaScript Object Notation)
JSON is a text-based format for representing structured data. It is widely used for data interchange between web clients and servers. JSON data is organized in a key-value format, making it easy to read and write.
SQLite
SQLite is a C-language library that provides a relational database management system. It is known for being lightweight, fast, and easy to integrate into applications. SQLite databases are stored in a single file, making them portable and convenient for local storage.
Steps to Convert JSON to SQLite
Converting JSON to SQLite involves several steps, including parsing the JSON data, creating an SQLite database, and inserting the data into the database tables.
Step 1: Parse the JSON Data
First, you need to parse the JSON data to extract the information you want to store in the SQLite database. You can use various programming languages to achieve this, such as Python, JavaScript, or Node.js. Here, we’ll use Python due to its simplicity and powerful libraries.
Step 2: Create an SQLite Database
Next, create an SQLite database and define the schema (tables and columns) that will hold the JSON data. This step involves establishing a connection to the database and executing SQL commands to create the necessary tables.
Step 3: Insert JSON Data into SQLite
Finally, insert the parsed JSON data into the SQLite database tables. This step involves preparing SQL INSERT statements and executing them to populate the tables with the JSON data.
Example Code
Below is an example of how to convert JSON data to SQLite using Python.
Sample JSON Data
[
{
"id": 1,
"name": "Alice",
"age": 30,
"city": "New York"
},
{
"id": 2,
"name": "Bob",
"age": 25,
"city": "Los Angeles"
}
]
Python Code
import json
import sqlite3
# Sample JSON data
json_data = '''
[
{
"id": 1,
"name": "Alice",
"age": 30,
"city": "New York"
},
{
"id": 2,
"name": "Bob",
"age": 25,
"city": "Los Angeles"
}
]
'''
# Parse JSON data
data = json.loads(json_data)
# Create SQLite database and table
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS people (
id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER,
city TEXT
)
''')
# Insert JSON data into SQLite table
for person in data:
c.execute('''
INSERT INTO people (id, name, age, city)
VALUES (?, ?, ?, ?)
''', (person['id'], person['name'], person['age'], person['city']))
# Commit the changes and close the connection
conn.commit()
conn.close()
Potential Issues and Solutions
Data Type Mismatch
Ensure that the data types in the JSON data match the column types in the SQLite database schema. For instance, if a column is defined as an integer, ensure that the corresponding JSON values are integers.
Handling Nested JSON
If the JSON data contains nested objects, you may need to flatten the structure or create additional tables to represent the nested data. This may involve additional parsing and SQL commands.
Large JSON Files
For large JSON files, consider using a streaming parser to process the data in chunks, reducing memory usage and improving performance.
Frequently Asked Questions
Can I use other programming languages to convert JSON to SQLite?
Yes, you can use other programming languages like JavaScript (Node.js), Java, or Ruby. The process involves similar steps: parsing JSON, creating an SQLite database, and inserting data.
How can I handle updates to the JSON data?
You can use SQL UPDATE statements to modify existing records in the SQLite database based on changes in the JSON data. Ensure you have a unique identifier in your JSON data to match records in the database.
Is there a way to automate this process?
Yes, you can create a script that periodically reads JSON data from a file or an API and updates the SQLite database. You can also use tools like Apache NiFi or Talend for more complex data integration tasks.
Conclusion
Converting JSON data to SQLite involves parsing the JSON, creating an appropriate database schema, and inserting the data into the database. This process can be efficiently implemented using various programming languages, with Python being a popular choice due to its powerful libraries and ease of use. By understanding and implementing the steps outlined in this article, you can effectively manage and utilize JSON data within an SQLite database.