How to Comment Out Multiple Lines in Python Shortcut
Commenting is an essential practice in programming. It allows developers to annotate code, making it easier to understand and maintain. In Python, comments are marked by the hash symbol (#).
While commenting out single lines is straightforward, commenting out multiple lines can take more time than required when not using the right techniques. In this guide, I’ll show you several effective techniques and shortcuts for commenting out multiple lines in Python efficiently.
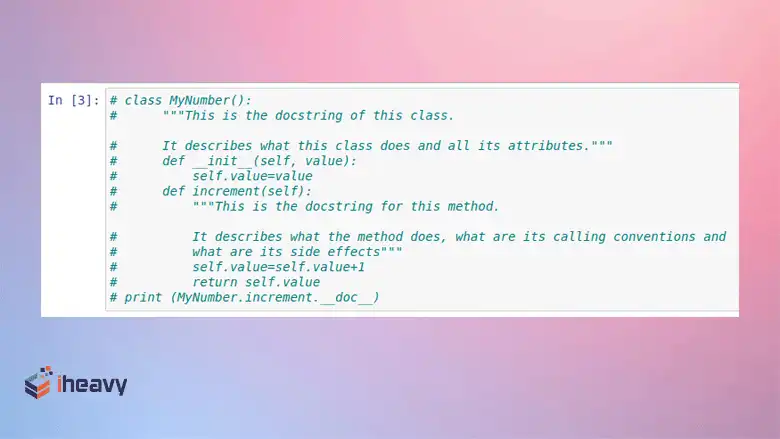
How Do I Comment Out Multiple Lines in Python?
Before diving into multiple-line comments, let’s quickly review single-line comments. A single-line comment in Python starts with a ‘#’ and extends to the end of the line. Here’s an example:
# This is a single-line comment
print(“Hello, world!”) # This comment is at the end of a line
With that out of the way, let’s now focus on how to comment out multiple lines of code. Here’s a couple of options worth trying.
Method 1: Using Multiple Single-Line Comments
The most straightforward way to comment out multiple lines is by placing a # at the beginning of each line. While this is simple, it can be time-consuming for large blocks of code.
# This is a comment
# spanning multiple lines
# in Python
print(“Hello, world!”)
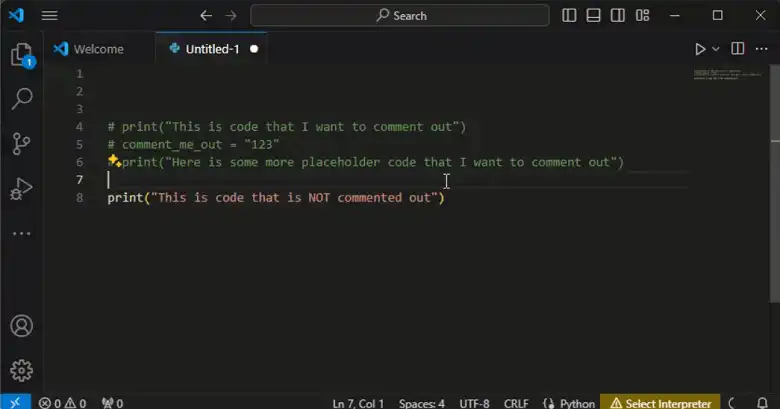
Method 2: Using Triple Quotes
Python does not have a specific syntax for multi-line comments. However, you can use triple quotes (”’ or “””) to create a multi-line string that is not assigned to any variable. Although this is not technically a comment, it can serve the same purpose for documenting code sections.
”’
This is a multi-line comment
using triple single quotes.
”’
print(“Hello, world!”)
“””
This is another multi-line comment
using triple double quotes.
“””
print(“Hello again!”)
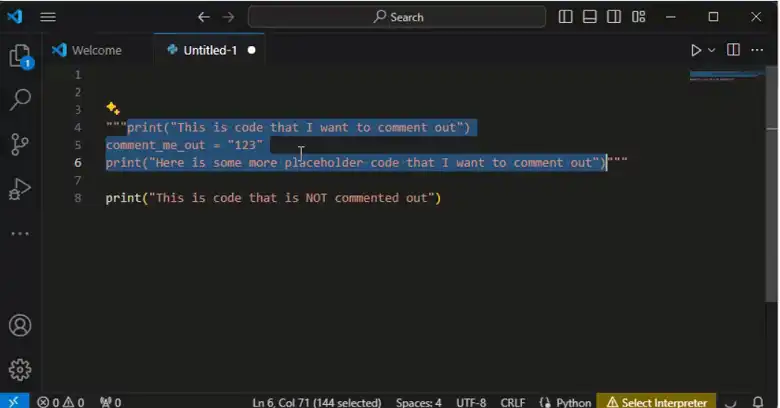
IDE Shortcuts for Commenting Multiple Lines
Modern Integrated Development Environments (IDEs) and text editors provide shortcuts to comment and uncomment multiple lines efficiently. Here are some common shortcuts for popular IDEs:
VS Code
Comment Multiple Lines: Select the lines you want to comment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Uncomment Multiple Lines: Select the lines you want to uncomment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
You can also add your own shortcut combination in VS code. To set a keyboard shortcut, start by selecting the settings icon located at the screen’s bottom left. From there, navigate to ‘Keyboard Shortcuts’ and locate the option labeled “toggle block…”. Choose this option and input the keyboard combination you prefer.
PyCharm
Comment Multiple Lines: Select the lines you want to comment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Uncomment Multiple Lines: Select the lines you want to uncomment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Jupyter Notebook
Comment Multiple Lines: Select the lines you want to comment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Uncomment Multiple Lines: Select the lines you want to uncomment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Sublime Text
Comment Multiple Lines: Select the lines you want to comment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Uncomment Multiple Lines: Select the lines you want to uncomment and press Ctrl + / (Windows/Linux) or Cmd + / (Mac).
Frequently Asked Questions
How do I comment out a block of code?
To comment out a block of code, simply highlight the desired code block and then press CTRL+Shift+/ (slash).
How do you break two lines in Python?
Ensure you use a single backslash ( \ ) to signify a line break. Using a double backslash ( \\ ) may lead to errors.
How to split Python code into multiple lines?
In Python, you can’t split a statement into multiple lines just by pressing Enter. Instead, use the backslash ( \ ) to indicate that a statement continues on the next line.
Conclusion
There are several ways to comment out multiple lines in Python, depending on your needs and preferences. Whether you use multiple single-line comments, triple quotes, or IDE shortcuts, it’s essential to choose a method that enhances your workflow and code readability. Have more thoughts? Comment below. And happy coding!