Column Name or Number of Supplied Values Does Not Match Table Definition
When working with SQL databases, encountering errors is part of the learning and debugging process. One common error in SQL Server is the “Column name or number of supplied values does not match table definition.”
This error typically arises when there is a mismatch between the number of columns specified in an INSERT statement and those defined in the table. In this article, we will explore the causes of this error, how to diagnose it, and various strategies to resolve it.
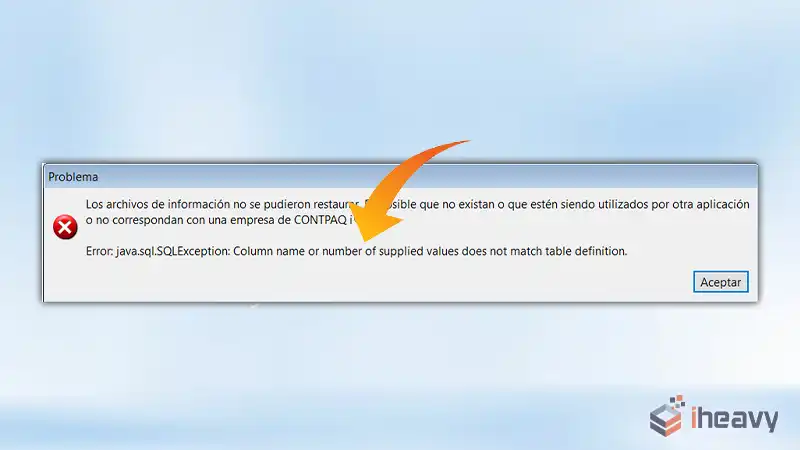
Understanding the Error
The error message “Column name or number of supplied values does not match table definition” indicates that the number of values being inserted into a table does not align with the number of columns defined in the table, or the column names specified do not match the table’s schema.
This can happen due to various reasons, such as omitting column names in an INSERT statement or incorrectly specifying the number of values.
Common Causes of the Error
Missing Column Names in the INSERT Statement
If you omit column names in an INSERT statement, SQL Server expects you to provide values for all columns in the table, in the exact order they are defined.
-- Table definition
CREATE TABLE Employees (
EmployeeID INT,
FirstName NVARCHAR(50),
LastName NVARCHAR(50),
HireDate DATE
);
-- Incorrect INSERT statement
INSERT INTO Employees VALUES (1, 'John', 'Doe');
In this example, the INSERT statement does not include a value for the HireDate column, resulting in an error.
Incorrect Number of Values Supplied
Providing more or fewer values than the number of columns in the table will also trigger this error.
-- Incorrect INSERT statement with too many values
INSERT INTO Employees (EmployeeID, FirstName, LastName, HireDate)
VALUES (1, 'John', 'Doe', '2023-01-01', 'ExtraValue');
Mismatched Column Names
Specifying incorrect or non-existent column names in the INSERT statement can cause this error.
-- Incorrect INSERT statement with mismatched column name
INSERT INTO Employees (EmployeeID, FirstName, LastName, DateOfHire)
VALUES (1, 'John', 'Doe', '2023-01-01');
How to Resolve the Error
Specify Column Names Explicitly
To avoid this error, always specify the column names explicitly in your INSERT statement. This practice not only prevents errors but also makes your code more readable and maintainable.
-- Correct INSERT statement
INSERT INTO Employees (EmployeeID, FirstName, LastName, HireDate)
VALUES (1, 'John', 'Doe', '2023-01-01');
Ensure the Correct Number of Values
Verify that the number of values matches the number of specified columns.
-- Correct INSERT statement with matching number of values
INSERT INTO Employees (EmployeeID, FirstName, LastName)
VALUES (1, 'John', 'Doe');
Match Column Names with Table Definition
Ensure that the column names in your INSERT statement match the column names defined in the table.
-- Correct INSERT statement with matching column names
INSERT INTO Employees (EmployeeID, FirstName, LastName, HireDate)
VALUES (1, 'John', 'Doe', '2023-01-01');
Example Code
Here is an example demonstrating the correct way to insert data into a table:
-- Create table
CREATE TABLE Products (
ProductID INT,
ProductName NVARCHAR(100),
Category NVARCHAR(50),
Price DECIMAL(10, 2)
);
-- Correct INSERT statement
INSERT INTO Products (ProductID, ProductName, Category, Price)
VALUES (1, 'Laptop', 'Electronics', 999.99);
Frequently Asked Questions
How can I avoid the “Column name or number of supplied values does not match table definition” error?
To avoid this error, always specify the column names in your INSERT statement and ensure the number of values matches the number of columns specified.
What should I do if I encounter this error?
If you encounter this error, review your INSERT statement to ensure that the column names match the table definition and that the number of values provided corresponds to the specified columns.
Can I insert values into only some columns of a table?
Yes, you can insert values into only some columns of a table by specifying the column names in your INSERT statement. The columns not specified will be set to their default values or NULL if no default value is defined.
-- Insert values into some columns
INSERT INTO Products (ProductID, ProductName)
VALUES (2, 'Smartphone');
Conclusion
The “Column name or number of supplied values does not match table definition” error is a common issue in SQL Server that arises from mismatches between the specified columns and values in an INSERT statement. By explicitly specifying column names and ensuring the correct number of values, you can avoid this error and ensure smooth data insertion into your tables. Understanding this error’s root causes and solutions will enhance your SQL querying skills and database management capabilities.