Cannot Perform an Aggregate Function on an Expression Containing an Aggregate or a Subquery | Solved
Aggregate functions in SQL, such as COUNT, SUM, AVG, MIN, and MAX, operate on sets of values to return a single value that summarizes the data. These functions are commonly used in conjunction with the GROUP BY clause to perform calculations on groups of rows rather than individual rows.
The error message “Cannot perform an aggregate function on an expression containing an aggregate or a subquery” typically occurs when an attempt is made to nest aggregate functions or subqueries in a manner that SQL cannot resolve.
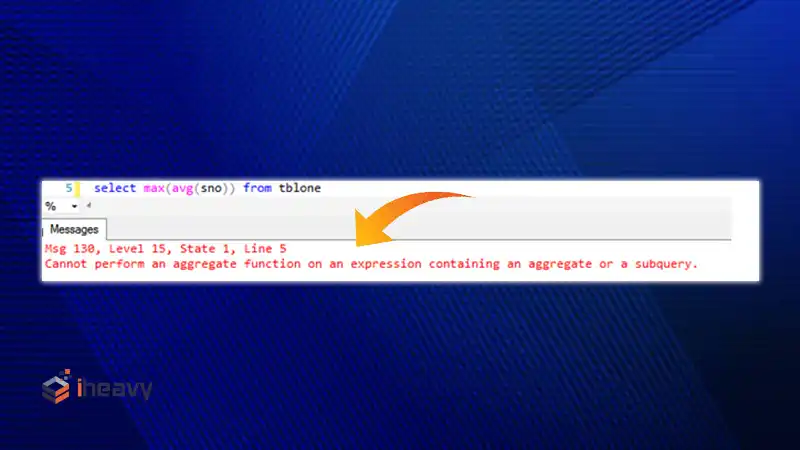
What Triggers the “Cannot Perform an Aggregate Function on an Expression Containing an Aggregate or a Subquery” Error?
This error typically occurs in SQL queries when attempting to use an aggregate function like SUM(), AVG(), COUNT(), etc., on a result that already contains an aggregate function or a subquery. Essentially, it’s a safeguard against potential logical inconsistencies in the query’s results.
Consider the following example:
SELECT SUM(AVG(sales_amount))
FROM sales_data;
In this query, an attempt is made to calculate the average sales amount for each group of rows and then sum up those averages. However, SQL cannot perform aggregate functions like AVG within another aggregate function like SUM directly.
Similarly, subqueries that return aggregate results can also trigger this error:
SELECT MAX(sales_amount)
FROM sales_data
WHERE sales_amount = (SELECT AVG(sales_amount) FROM sales_data);
Here, the subquery calculates the average sales amount, which is then compared to the sales amount in the outer query. This nesting of aggregate functions within subqueries leads to the error.
How to Resolve the Aggregate Function Error
To address this error, it’s necessary to refactor the SQL query to avoid nesting aggregate functions or subqueries in a way that SQL cannot process.
Option 1: Use Common Table Expressions (CTEs)
Common Table Expressions (CTEs) can help break down complex queries into more manageable parts. By calculating intermediate results with CTEs, you can avoid nesting aggregate functions directly. Here’s an example:
WITH avg_sales AS (
SELECT AVG(sales_amount) AS average_sales
FROM sales_data
)
SELECT SUM(average_sales)
FROM avg_sales;
In this query, the average sales amount is calculated in a CTE, and then the SUM function is applied to the result in the main query.
Option 2: Use Subqueries Wisely
Instead of nesting aggregate functions directly, consider using subqueries to filter data before applying aggregate functions. For instance:
SELECT SUM(sales_amount)
FROM (
SELECT sales_amount
FROM sales_data
WHERE sales_amount > (SELECT AVG(sales_amount) FROM sales_data)
) AS filtered_sales;
Here, the subquery filters the sales data based on a condition involving the average sales amount before applying the SUM function in the outer query.
Frequently Asked Questions
Can I use aggregate functions inside subqueries?
You can use aggregate function inside subqueries. But be cautious not to nest them in a way that creates ambiguity for the database engine.
Which clause cannot be used with an aggregate function?
You can’t use aggregate functions in a WHERE clause. This restriction exists because aggregate functions are designed to operate on groups of rows, not on individual rows.
Conclusion
When encountering the error message “cannot perform an aggregate function on an expression containing an aggregate or a subquery,” it’s essential to understand what it means and how to address it effectively. By restructuring your queries thoughtfully and leveraging techniques like nested queries, CTEs, or analytic functions, you can overcome this obstacle and produce accurate results.