Building Dynamic SQL Statements in SQL Server | Explained
Dynamic SQL allows developers to construct SQL statements dynamically at runtime, providing flexibility in query generation based on varying conditions or parameters. In SQL Server, dynamic SQL is commonly used in scenarios where the structure of the query or the objects involved may change dynamically. In this article, we’ll explore how to build dynamic SQL statements in SQL Server and address common questions related to this topic.
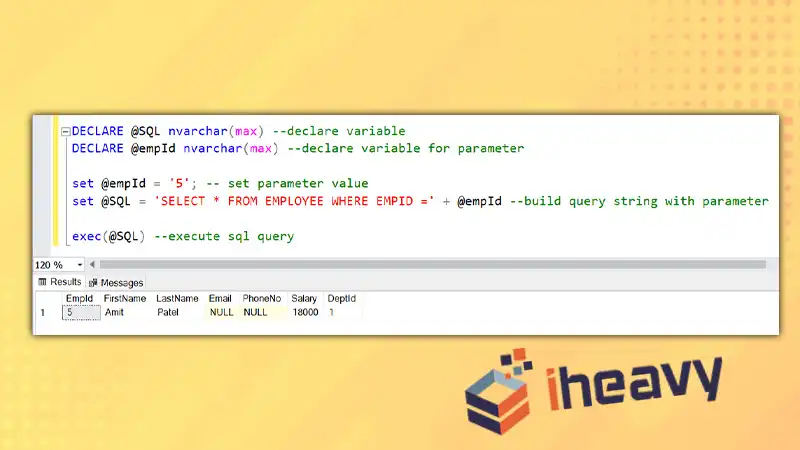
Constructing Dynamic SQL Statements
Dynamic SQL statements in SQL Server are typically built using string concatenation or string manipulation techniques. The following steps outline the process of constructing a dynamic SQL statement:
Define the SQL Statement Structure
Determine the base structure of the SQL statement, including the SELECT, INSERT, UPDATE, DELETE, or EXECUTE statement, along with placeholders for dynamic elements.
Concatenate Dynamic Elements
Concatenate dynamic elements such as column names, table names, conditions, or parameters into the base SQL statement using string concatenation or string interpolation.
Execute the Dynamic SQL Statement
Execute the dynamically constructed SQL statement using methods such as sp_executesql or EXECUTE.
sql
DECLARE @sql NVARCHAR(MAX);
DECLARE @tableName NVARCHAR(50) = 'YourTableName';
DECLARE @columnName NVARCHAR(50) = 'YourColumnName';
DECLARE @condition NVARCHAR(100) = 'YourCondition';
SET @sql = 'SELECT ' + @columnName + ' FROM ' + @tableName + ' WHERE ' + @condition;
EXEC sp_executesql @sql;
Best Practices for Dynamic SQL
When building dynamic SQL statements in SQL Server, consider the following best practices to ensure security, performance, and maintainability:
Parameterization
Whenever possible, use parameterization to pass dynamic values to the SQL statement, rather than concatenating values directly into the string. This helps prevent SQL injection attacks and improves query plan reuse.
Quoting and Escaping
Properly quote and escape dynamic elements to handle special characters and prevent syntax errors. Use functions such as QUOTENAME or QUOTED_IDENTIFIER to safely handle identifiers.
Error Handling
Implement error handling mechanisms to handle exceptions that may occur during the execution of dynamic SQL statements. Use TRY…CATCH blocks to capture and handle errors gracefully.
Frequently Asked Questions (FAQ)
What are the security implications of using dynamic SQL?
Dynamic SQL can expose your application to SQL injection attacks if not implemented properly. Always use parameterization and proper quoting/escaping techniques to mitigate security risks.
Can I use dynamic SQL in stored procedures?
Yes, dynamic SQL can be used within stored procedures to dynamically generate and execute SQL statements based on runtime conditions or parameters.
Are there performance considerations when using dynamic SQL?
Dynamic SQL may have performance implications, especially if executed frequently or in high-volume environments. Be mindful of query plan caching and parameter sniffing issues and consider using options like sp_executesql for parameterized execution.
Conclusion
Dynamic SQL provides developers with the flexibility to construct SQL statements dynamically based on varying requirements or conditions. By following best practices and guidelines for constructing and executing dynamic SQL statements, developers can build robust and secure applications in SQL Server.