What Is the Alternative for DISTINCT Keyword in SQL?
In SQL, the DISTINCT keyword is commonly used to remove duplicate records from query results. It ensures that only unique values are returned from a column or a combination of columns.
However, there are situations where DISTINCT may not be the most efficient or appropriate option, especially in complex queries or performance-critical applications. This article explores various alternatives to DISTINCT in SQL, providing detailed examples and explanations for each method.
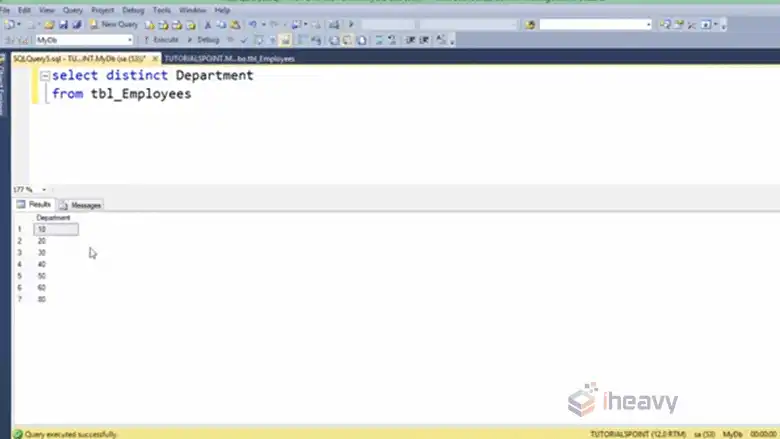
Why Consider Alternatives to DISTINCT?
Here are a few reasons why you might want to consider a different approach instead of using DISTINCT.
Performance: DISTINCT can be resource-intensive, especially on large datasets.
Flexibility: Alternatives may offer more flexibility in handling complex data manipulation tasks.
Readability: Certain alternatives can make queries more readable and maintainable.
How Can I Get Distinct Values in SQL Without DISTINCT?
This section explores various techniques to achieve the same result without using DISTINCT.
1. Using GROUP BY
One of the most common alternatives to DISTINCT is the GROUP BY clause. It groups rows that have the same values in specified columns into aggregated data.
Example:
SELECT name, COUNT(*)
FROM employees
GROUP BY name;
This query groups employees by their names and counts how many times each name appears in the dataset.
The GROUP BY clause organizes rows into groups with the same name. This is particularly useful when you want to apply aggregate functions like COUNT, SUM, AVG, etc., to each group.
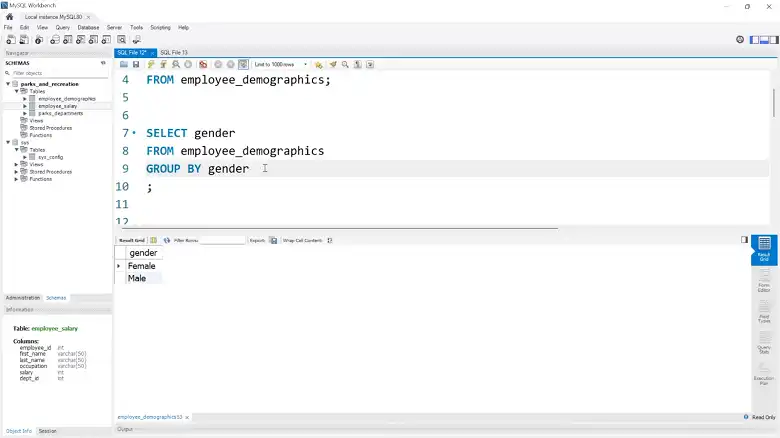
2. Using ROW_NUMBER() with Common Table Expressions (CTE)
ROW_NUMBER() is a window function that assigns a unique sequential integer to rows within a partition. It can be combined with Common Table Expressions (CTE) to remove duplicates.
Example:
WITH RankedEmployees AS (
SELECT name, ROW_NUMBER() OVER (PARTITION BY name ORDER BY id) AS rn
FROM employees
)
SELECT name
FROM RankedEmployees
WHERE rn = 1;
This query assigns a rank to each employee within partitions of the same name and selects only the first occurrence.
The ROW_NUMBER() function assigns a number to each row within the same partition (in this case, name). The CTE (RankedEmployees) then selects only the first occurrence (rn = 1), effectively filtering out duplicates.
3. Using UNIQUE Constraint
While not a direct alternative to DISTINCT, applying a UNIQUE constraint on a column ensures that all values are unique at the database schema level.
Example:
CREATE TABLE unique_employees (
id INT PRIMARY KEY,
name VARCHAR(100) UNIQUE
);
Here, the UNIQUE constraint on the name column ensures that no duplicate names can be inserted into the unique_employees table. This constraint prevents duplicates from being entered into the database in the first place, ensuring data integrity.
4. Using DISTINCT ON in PostgreSQL
PostgreSQL provides the DISTINCT ON feature, which allows you to specify distinct rows based on specific columns. This feature isn’t available in other SQL dialects like MySQL or SQL Server.
Example:
SELECT DISTINCT ON (name) id, name, department
FROM employees
ORDER BY name, id;
This query selects distinct names along with their corresponding id and department. DISTINCT ON (name) ensures that only the first occurrence of each name is returned, based on the ORDER BY clause, which determines which row to keep.
5. Using EXISTS Clause
The EXISTS clause is used to test for the existence of rows in a subquery. It can be employed to retrieve unique records.
Example:
SELECT name
FROM employees e1
WHERE EXISTS (
SELECT 1
FROM employees e2
WHERE e1.name = e2.name
);
This query checks for the existence of duplicate names and returns distinct results. The EXISTS clause checks if there are any rows in the subquery (e2) with the same name as the outer query (e1). If so, it returns the name, effectively filtering duplicates.
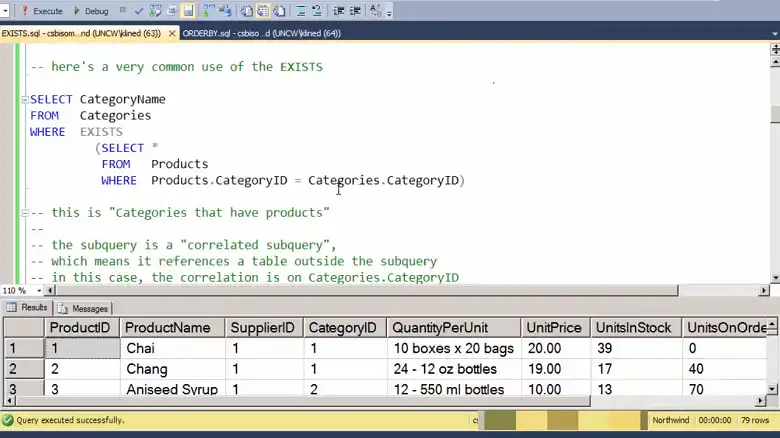
6. Using DISTINCT with Aggregate Functions
Sometimes combining DISTINCT with aggregate functions like MAX, MIN, or SUM can be more efficient than using DISTINCT alone.
Example:
SELECT name, MAX(salary) AS highest_salary
FROM employees
GROUP BY name;
Frequently Asked Questions
How to avoid distinct in SQL?
You might want to avoid DISTINCT when dealing with very large datasets where performance is a concern. In such cases, using GROUP BY or indexing might be more efficient.
Can I use DISTINCT with multiple columns?
Yes, DISTINCT can be used with multiple columns to return unique combinations of those columns. For example: SELECT DISTINCT name, department FROM employees;
Is unique and distinct the same in SQL?
DISTINCT is a query-level operation that removes duplicates from result sets. In contrast, UNIQUE is a constraint that enforces uniqueness at the database schema level.
Conclusion
While the DISTINCT keyword is a powerful tool for removing duplicates in SQL, there are several alternatives and techniques that can be more efficient or appropriate depending on the specific requirements of your queries. By understanding these alternatives, you can optimize your SQL queries for performance and readability.