Hash Join vs. Nested Loop Join
When optimizing SQL queries, understanding the different join algorithms can be extremely beneficial. Two of the most common join methods are Hash Join and Nested Loop Join. Hash joins often require more time to retrieve the initial row compared to nested-loop joins. This is because the database system must construct a hash table before any results can be returned.
However, in certain situations, the overall query execution time can be faster with hash joins, especially for larger datasets. That explains only one scenario while this article explains more, illustrating the mechanisms, use cases, and performance considerations for both methods.
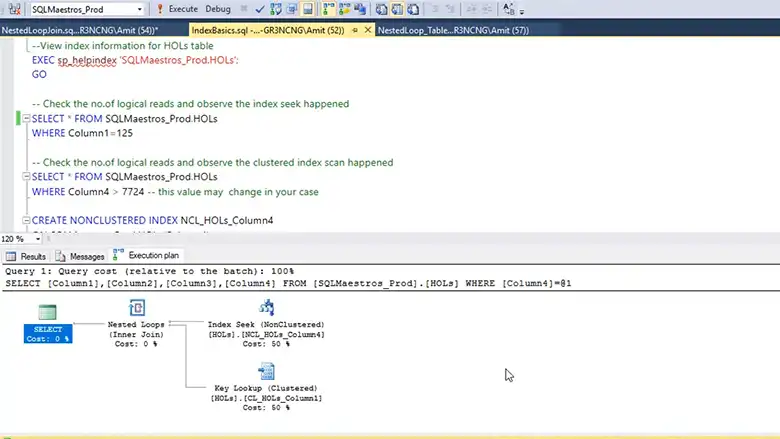
Hash Join
A Hash Join is typically used for equi-joins (joins based on equality conditions). It involves building a hash table on the smaller dataset and then probing the hash table with each row from the larger dataset. This method is highly efficient for large datasets where sorting is not feasible.
What Are the Phases of Hash Join?
Hash joins work in two phases. They are:
Build Phase: The smaller dataset, often called the “build input,” is scanned, and a hash table is created based on the join key. Each entry in the hash table contains the join key and the corresponding row data.
SELECT orders.order_id, customers.customer_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id;
Here, a hash table is built using the customer_id from the customers table, which is smaller in size.
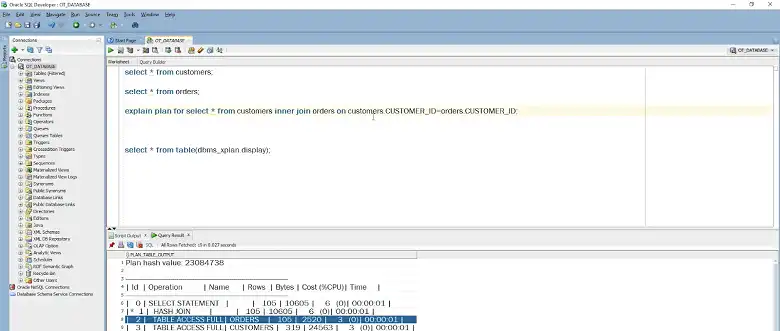
Probe Phase: The larger dataset, known as the “probe input,” is scanned. For each row, the join key is looked up in the hash table. If a match is found, the rows are combined.
This approach ensures that each row from the larger dataset only needs to be processed once, making the Hash Join particularly effective for large datasets where the smaller table fits into memory.
When to Use Hash Join?
Hash joins are more efficient for large datasets, while merge joins are better suited for tables that are already sorted. PostgreSQL’s query optimizer intelligently selects the most appropriate join method based on factors like table size and data organization.
Nested Loop Join
A Nested Loop Join is more straightforward but can be less efficient for large datasets. It involves scanning one dataset (the outer loop) and, for each row in this dataset, scanning the other dataset (the inner loop) to find matching rows. It is usually more suitable for small datasets.
How Nested Loop Join Works
Here’s how a nested loop join works.
Outer Loop: The first dataset is scanned row by row. This dataset is often referred to as the “outer input.”
SELECT orders.order_id, customers.customer_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id;
Here, the orders table might be the outer input, meaning each order is processed individually.
Inner Loop: For each row in the outer dataset, the second dataset (inner input) is scanned. The join condition is evaluated for each pair of rows.
In cases where the inner input is not indexed, the performance of a Nested Loop Join can degrade significantly, as it may involve a large number of comparisons.
What Are the Differences Between Hash Join and Nested Loops?
The table below highlights the key differences between Hash Join and Nested Loop Join, helping you choose the appropriate join method based on your specific requirements.
Feature | Hash Join | Nested Loop Join |
Primary Use Case | Best for equi-joins on large datasets | Suitable for smaller datasets or complex join conditions |
Algorithm Mechanism | Builds a hash table for the smaller dataset, probes with the larger dataset | Scans one dataset (outer loop), compares with the other dataset (inner loop) |
Data Size Suitability | Efficient for large datasets | More efficient for small datasets |
Index Requirement | Does not require indexes | Benefits significantly from indexes |
Memory Usage | Memory-intensive (requires memory to store hash table) | Less memory-intensive |
Join Condition Flexibility | Optimized for equality conditions | Handles a wide range of join conditions, including inequalities |
Performance | Fast when the smaller table fits into memory; can degrade if it doesn’t | Can be slow for large datasets without indexes; efficient with indexes |
Impact of Indexes | Minimal impact from indexes | Dramatically improves performance with selective indexes |
Complexity | More complex algorithm | Simpler algorithm |
Typical Use Cases | Data warehousing, large transactional systems | Smaller datasets, situations where indexes are available |
Performance Considerations
- Data Size: Hash Joins tend to perform better with larger datasets, especially when the smaller table can fit entirely into memory. Nested Loop Joins are more efficient with smaller datasets or when the outer dataset has significantly fewer rows.
- Indexing: Nested Loop Joins benefit greatly from indexes on the join keys, which can significantly reduce the number of comparisons. Hash Joins do not require indexes but do require memory to store the hash table.
- Memory Usage: Hash Joins can be memory-intensive, particularly if the hash table becomes too large to fit in memory. Nested Loop Joins, while less memory-intensive, can be computationally expensive.
- Join Conditions: Hash Joins are optimized for equality conditions, while Nested Loop Joins can handle a broader range of conditions, including inequalities.
Frequently Asked Questions
Can Nested Loop Joins be used for non-equality joins?
Yes, Nested Loop Joins are versatile and can handle a variety of join conditions, including inequalities and complex expressions.
Is hash join faster than merge join?
Merge joins are often more efficient than hash joins, especially when dealing with large datasets or when the joining columns are already sorted. They require less memory and can often execute faster. However, hash joins can be faster in cases where the tables are not sorted and there’s sufficient memory to build a hash table.
What is the complexity of Hash Join?
Hash joins have a time complexity of O(m+n), meaning the time taken increases linearly with the size of the tables (m and n). This is because the algorithm needs to scan both tables once.
Conclusion
Understanding when to use Hash Join or Nested Loop Join is essential for optimizing SQL queries. While Hash Joins are powerful for large datasets and equi-joins, Nested Loop Joins offer flexibility and efficiency for smaller datasets and complex join conditions.