Self Join in SQL Example: Employee Manager
A self join is a join in which a table is joined with itself. This can be particularly useful when analyzing hierarchical data, such as employee-manager relationships within an organization. In such a scenario, each employee has an associated manager, and managers are also employees of the company.
In this article, I’ll explain how to tackle such a scenario with a proper example and explanation that’s easy to understand. So, why bother waiting? Let’s get started.
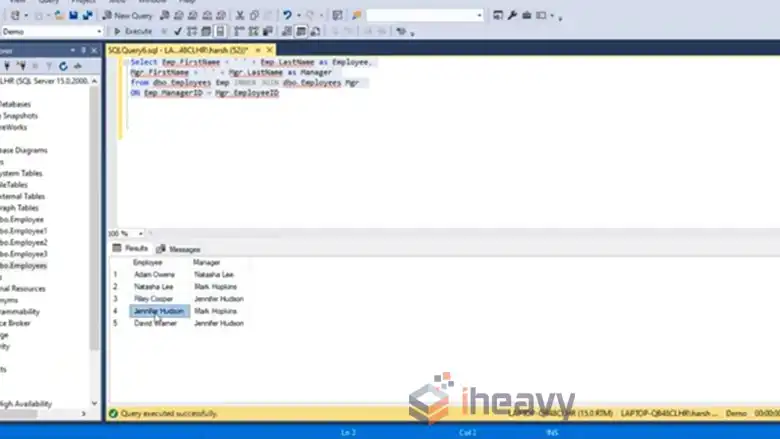
What Is Self Join in SQL?
A self-join is a SQL join where a table is joined to itself. It’s used to compare data within the same table, similar to joining two different tables. This is often employed to find hierarchical relationships or duplicate values.
Let’s consider an employees table with columns: employee_id, name, and manager_id.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
name VARCHAR(50),
manager_id INT
);
To find the name of each employee and their corresponding manager’s name, we can use a self-join:
SELECT e.name AS EmployeeName, m.name AS ManagerName
FROM employees e
LEFT JOIN employees m ON e.manager_id = m.employee_id;
In this query:
- e is an alias for the employees table representing employees.
- m is an alias for the employees table representing managers.
- The LEFT JOIN ensures that all employees are included, even if they don’t have a manager.
The result will show a list of employees and their respective managers.
How Many Tables Do You Need to Create a Self Join?
You only need one table to perform a self-join. Despite the name suggesting otherwise, a self-join involves joining a table with itself within a single SQL query. It’s essentially treating the same table as two separate tables for the purpose of the join.
This allows you to compare or relate data within the same table, such as finding hierarchical relationships or duplicate values.
To achieve this, you’ll typically use aliases to differentiate between the two instances of the table in the join condition. This creates a virtual relationship between rows within the same table, enabling you to extract the desired information.
Setting Up the Employee Table
Consider a simple Employees table with the following columns:
- EmployeeID: Unique identifier for each employee.
- EmployeeName: The name of the employee.
- ManagerID: References the EmployeeID of the employee’s manager.
Here’s how the table might look:
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
EmployeeName VARCHAR(100),
ManagerID INT
);
The ManagerID is a foreign key that references the EmployeeID in the same table, which establishes the hierarchical relationship.
Performing a Self Join
To retrieve data showing each employee along with their manager, you can use a self join. In this case, the table is joined to itself, matching the ManagerID of one row with the EmployeeID of another row.
Basic Self Join:
SELECT
e.EmployeeName AS Employee,
m.EmployeeName AS Manager
FROM
Employees e
JOIN
Employees m ON e.ManagerID = m.EmployeeID;
This query selects the EmployeeName from the Employees table twice. The first reference (e) is for the employee, while the second (m) is for the manager. The join condition e.ManagerID = m.EmployeeID matches each employee to their manager by linking the ManagerID of the employee with the EmployeeID of the manager.
Using Aliases for Clarity
Aliases (e and m) are used to distinguish between the two instances of the Employees table. This method improves readability and reduces ambiguity.
Handling Employees Without Managers
Some employees might not have a manager, such as the CEO. To include such employees in the result, you can use a LEFT JOIN. Here’s how you do a self join with a left join in SQL.
SELECT
e.EmployeeName AS Employee,
COALESCE(m.EmployeeName, 'No Manager') AS Manager
FROM
Employees e
LEFT JOIN
Employees m ON e.ManagerID = m.EmployeeID;
Here, COALESCE is used to replace any NULL values in the Manager column with the text ‘No Manager’. The LEFT JOIN ensures that all employees are listed, even if they don’t have a corresponding manager.
Understanding the Hierarchical Structure
If you want to display the entire hierarchy, including multiple levels of management, you can extend the self join logic by joining the table multiple times. However, this approach is more complex and typically requires recursive queries or Common Table Expressions (CTEs) for deeper levels of hierarchy.
Frequently Asked Questions
Can I use a self join for multi-level hierarchies?
Yes, but handling multi-level hierarchies using self joins can be complex. For such cases, you might consider using recursive queries or Common Table Expressions (CTEs) to traverse the hierarchy effectively.
Is self join same as inner join SQL?
An INNER JOIN combines rows from different tables based on matching values. A SELF JOIN combines rows from the same table based on matching values, allowing comparisons within the table.
What is the disadvantage of self join in SQL?
Self-joins can be complex and inefficient, especially when dealing with large datasets. The added complexity of joining a table to itself can make queries harder to understand and optimize.
Conclusion
This article explored the concept of self-joins in SQL using an employee-manager example. I tried to demonstrate how to establish a relationship between rows within the same table to retrieve hierarchical data. I hope this explanation clarifies the concept of self-joins. If you have any further questions or require more complex examples, feel free to ask. Thank you for reading!