Divide by Zero Error Encountered in SQL | But Why?
Divide by zero errors are among the most common issues encountered in SQL queries, particularly when dealing with mathematical calculations or aggregations. A divide by zero error occurs when an attempt is made to divide a number by zero, leading to an undefined result.
This not only disrupts query execution but can also cause applications to crash or behave unpredictably. Understanding how to prevent and handle these errors is crucial for building robust SQL queries.
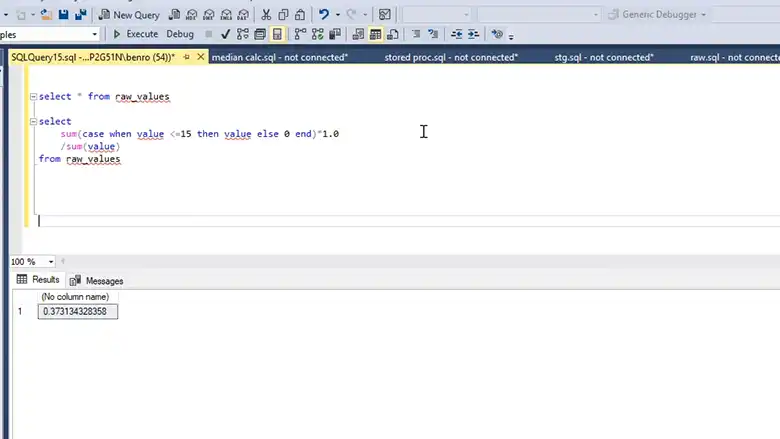
What Causes a Divide by Zero Error?
In SQL, a divide by zero error occurs when the denominator in a division operation evaluates to zero. Since division by zero is mathematically undefined, SQL Server and other database systems generate an error, halting the query’s execution. This can happen in a variety of scenarios:
Data Input Errors: Users or processes might enter zero in fields that are used as denominators.
Null Values: Columns that contain NULL values might be interpreted as zero in certain operations.
Dynamic Calculations: Calculations that derive their denominator from other columns or expressions can inadvertently result in zero.
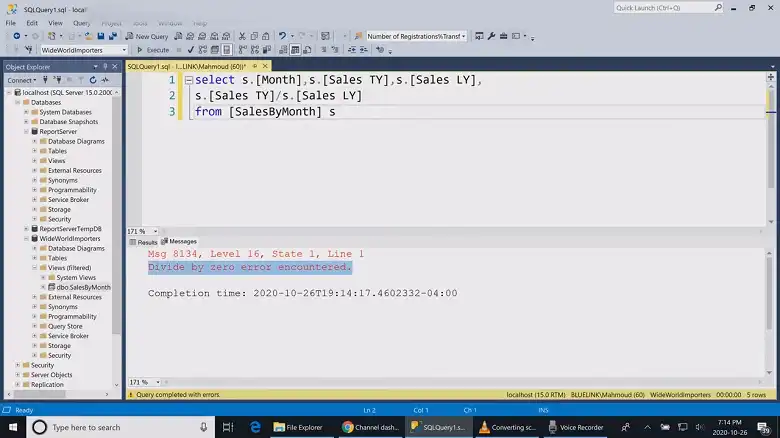
How to Avoid Divide by Zero?
Though a divide by zero is not something you can fix once it occurs, you can take preventative measures to make sure you don’t encounter it in the first place. Here’s how.
1. Using Conditional Logic
One of the most straightforward methods to prevent divide by zero errors is to use conditional logic to check the denominator before performing the division. This can be done using the CASE statement in SQL:
SELECT
numerator / CASE
WHEN denominator = 0 THEN NULL
ELSE denominator
END AS result
FROM table_name;
In this approach, if the denominator is zero, the division is bypassed, and NULL is returned instead.
2. Leveraging NULLIF Function
The NULLIF function can be particularly useful for avoiding divide by zero errors. This function returns NULL if the two arguments are equal; otherwise, it returns the first argument. It can be used to ensure that a zero denominator is treated as NULL, avoiding the error.
SELECT
numerator / NULLIF(denominator, 0) AS result
FROM table_name;
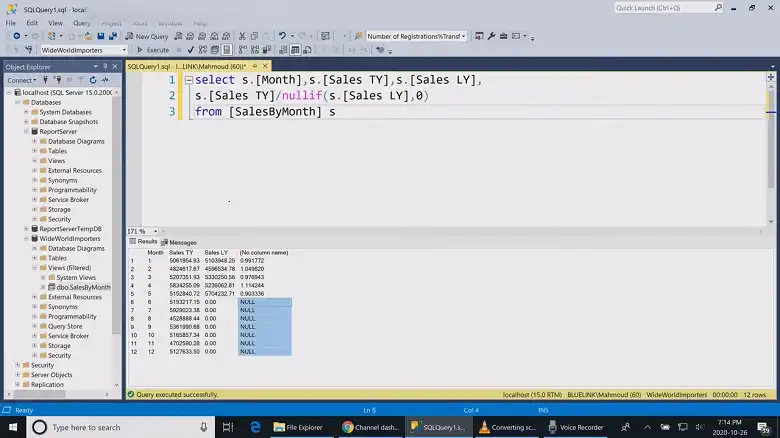
This method is concise and effective, making it a popular choice among SQL developers.
3. Implementing Default Values
In some cases, you might want to replace a zero denominator with a default value, such as 1, to ensure that the division operation can proceed. This can be done using the ISNULL or COALESCE functions:
SELECT
numerator / ISNULL(denominator, 1) AS result
FROM table_name;
Or
SELECT
numerator / COALESCE(NULLIF(denominator, 0), 1) AS result
FROM table_name;
Both approaches allow you to define a fallback value that is used when the denominator is zero.
Impact of Divide by Zero Errors
Divide by zero errors can have significant impacts on your database and applications:
Query Failures: A divide by zero error will stop the execution of a query, potentially leaving transactions incomplete or data unprocessed.
Application Crashes: If the error occurs in a critical part of the application, it might lead to crashes or unhandled exceptions.
Data Integrity Issues: Misinterpreting or mishandling divide by zero scenarios can lead to incorrect data being stored or displayed, undermining the integrity of your data.
Proper error handling and validation in SQL can mitigate these risks, ensuring your queries run smoothly and your data remains reliable.
Frequently Asked Questions
How to avoid divide by zero error in Oracle SQL?
Dividing by zero in SQL can produce unexpected results or even halt your query. To prevent this, use the `NULLIF` function. It replaces a value with `NULL` if it matches a specified value. For example, `NULLIF (denominator, 0)` will return `NULL` if the denominator is zero, avoiding the division error.
Can divide by zero errors be ignored?
Ignoring divide by zero errors is not recommended as it can lead to incomplete query execution and unpredictable application behavior. It’s better to handle them explicitly using conditional logic or functions like NULLIF.
How do you fix a division by zero error?
If a divide by zero error has already occurred, you can fix it by revising the query to include one of the prevention techniques mentioned above.
Conclusion
Divide by zero errors in SQL can disrupt query execution and compromise data integrity if not properly handled. However, by implementing the right strategies—such as using conditional logic, leveraging the NULLIF function, or applying default values—you can effectively prevent these errors and ensure that your queries run smoothly.
These approaches not only mitigate the risk of encountering divide by zero errors but also enhance the overall robustness and reliability of your database operations.