Count How Many Times a Value Appears in a Column SQL
In SQL, counting how many times a specific value appears in a column is a common operation. This task can be useful in various scenarios, such as identifying the most frequent values, detecting anomalies, or simply summarizing data.
There are several ways to achieve this in SQL, depending on the database system and the specifics of the query. This article will explore different methods to count occurrences of a value in a column, providing detailed explanations for each approach.
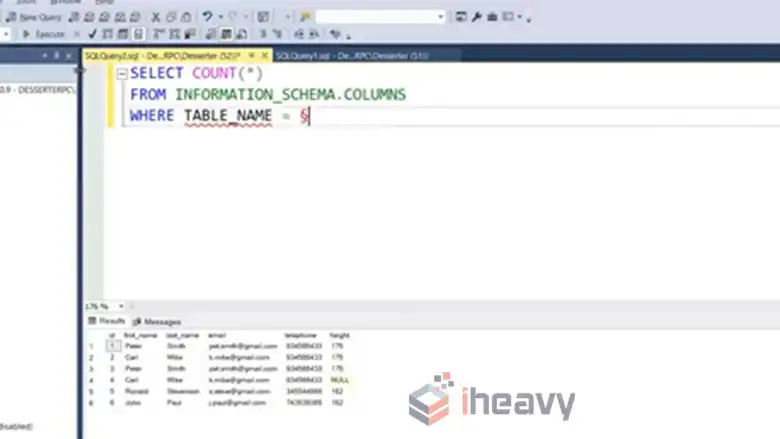
How to Count Occurrences of a Value in an SQL Column?
Below are the top 5 methods that you can use to efficiently count value occurrences in an SQL column.
1. Using the COUNT() Function with GROUP BY
One of the most straightforward ways to count the occurrences of each value in a column is by using the COUNT() function in combination with the GROUP BY clause. This method allows you to count the number of times each unique value appears in the specified column.
SELECT column_name, COUNT(*) AS value_count
FROM table_name
GROUP BY column_name;
Here’s what happens:
- SELECT column_name, COUNT(*): This retrieves the unique values in column_name and counts how many times each one appears.
- FROM table_name: This specifies the table where the column is located.
- GROUP BY column_name: This groups the results by each unique value in the column, so the count is calculated for each group.
For instance, if you have a table orders with a column product_id, you could use the query to count how many times each product_id appears.
2. Counting Specific Values with WHERE
If you’re interested in counting the occurrences of a specific value rather than all unique values, you can use the WHERE clause in conjunction with the COUNT() function.
SELECT COUNT(*) AS value_count
FROM table_name
WHERE column_name = 'specific_value';
In this query:
- SELECT COUNT(*): Counts all rows where the condition is true.
- WHERE column_name = ‘specific_value’: Filters the rows to include only those where column_name equals the value you’re interested in.
For example, if you wanted to know how many times the product_id 123 appears in the orders table, you would replace ‘specific_value’ with 123.
3. Using COUNT() with a Subquery
Another method is to use a subquery to count the occurrences of each value and then select the one you’re interested in. This is particularly useful when you need to count a specific value but also want to see other values or columns in the same query.
SELECT value_count
FROM (
SELECT column_name, COUNT(*) AS value_count
FROM table_name
GROUP BY column_name
) AS subquery
WHERE column_name = 'specific_value';
This query works as follows:
- The inner query (SELECT column_name, COUNT(*) …) performs the counting as in the first method.
- The outer query selects the count for the specific value using the WHERE clause.
This method might be more complex, but it can be useful when integrating the count into more detailed or combined queries.
4. Using the HAVING Clause for Filtering Counts
The HAVING clause is often used in conjunction with GROUP BY to filter groups based on aggregate functions. If you want to count how many values appear more than a certain number of times, you can use HAVING.
SELECT column_name, COUNT(*) AS value_count
FROM table_name
GROUP BY column_name
HAVING COUNT(*) > 5;
In this case:
- HAVING COUNT(*) > 5: Filters the results to show only those values that appear more than 5 times.
This approach is useful when you want to identify values that exceed a certain frequency threshold.
5. Window Functions for Cumulative Counting
Window functions, available in many SQL dialects, allow you to perform calculations across a set of table rows that are related to the current row. COUNT() can be used as a window function to create cumulative counts without collapsing the rows into groups.
SELECT column_name,
COUNT(*) OVER (PARTITION BY column_name) AS value_count
FROM table_name;
This query works by:
- PARTITION BY column_name: Dividing the rows into partitions based on the value in column_name.
- COUNT(*) OVER (…): Counting the number of rows within each partition, providing the count for each row in the original table.
This method retains all original rows while adding a column that shows the count of each value.
Frequently Asked Questions
Can I count occurrences of multiple specific values in a single query?
Yes, you can count occurrences of multiple specific values by using the IN clause with WHERE.
What is count( ) in SQL?
COUNT( ) is an SQL function used to count the total number of rows in a table. It includes all rows, even those with NULL values. This is helpful for determining the overall size of a dataset.
What is the Countif formula in SQL?
The COUNTIFS function counts the number of cells that meet multiple conditions. You specify a range of cells and the criteria for each range. The function returns the count of rows where all conditions are true.
Conclusion
This article has provided a detailed overview of different methods for counting occurrences of values in a column using SQL. Understanding these techniques can greatly enhance your ability to analyze and interpret data efficiently. If you have any further queries or methods you want to share, feel free to do so. Thanks for reading!