How To Insert Datatable Values Into Database In C#
When working with databases in C#, efficiently inserting data from a DataTable into your database is a common yet crucial task. Whether you’re dealing with a handful of rows or large datasets, understanding how to seamlessly bridge the gap between your in-memory data structures and persistent storage can significantly impact performance and reliability.
By leveraging ADO.NET, you can systematically insert rows from a DataTable into your database, ensuring data integrity and optimizing operations, whether you’re handling routine updates or bulk data imports.
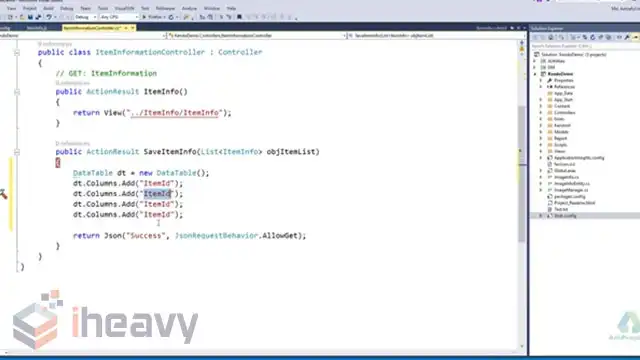
Step-by-Step Guide To Insert Datatable Values Using C#
To insert values from a DataTable into a database in C#, you can use ADO.NET. Below is an example of how to achieve this. The process involves iterating through each row in the DataTable and inserting them into the database using an INSERT SQL statement.
1. Set Up Your Connection
Before you can insert data into the database, you need to establish a connection to it. This involves using the SqlConnection class in ADO.NET.
Steps:
Connection String: The connection string contains information about the database, including the server, database name, authentication details, etc.
Open the Connection: Once you have a connection string, you need to open the connection using the Open() method of the SqlConnection object.
string connectionString = "Server=your_server;Database=your_database;User Id=your_username;Password=your_password;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// Connection is now open and ready to use.
}
Considerations:
Security: Keep the connection string secure, especially if it contains sensitive information like passwords. Consider using integrated security (Windows Authentication) or storing the connection string in a configuration file with encryption.
Connection Pooling: ADO.NET automatically manages a pool of connections to improve performance. When using using, the connection is automatically returned to the pool when done.
2. Prepare Your SQL Command
Next, you need to prepare the SQL command that will be used to insert data into the database. This involves creating an INSERT INTO statement and using parameters to prevent SQL injection.
Steps:
SQL Statement: Write your SQL INSERT INTO statement with placeholders for parameters.
Parameters: Use SqlParameter objects or the AddWithValue method to bind values from the DataTable to the command parameters.
using (SqlCommand cmd = new SqlCommand("INSERT INTO YourTable (Column1, Column2) VALUES (@Value1, @Value2)", connection))
{
cmd.Parameters.AddWithValue("@Value1", someValue1);
cmd.Parameters.AddWithValue("@Value2", someValue2);
// Command is now ready to be executed.
}
Considerations:
SQL Injection: Always use parameters to avoid SQL injection vulnerabilities. Do not concatenate user inputs directly into your SQL queries.
Data Types: Ensure that the data types in your DataTable match the data types expected by the database.
3. Iterate Through the DataTable
You need to loop through each row in the DataTable, extract the data, and execute the SQL command for each row.
Steps:
For Each Loop: Use a foreach loop to iterate through the rows of the DataTable.
Bind Data: For each row, bind the data to the SQL command parameters.
foreach (DataRow row in dataTable.Rows)
{
cmd.Parameters["@Value1"].Value = row["Column1"];
cmd.Parameters["@Value2"].Value = row["Column2"];
// Command parameters are now set with values from the current row.
}
Considerations:
Null Values: Handle potential DBNull values in your DataTable to avoid exceptions.
Batch Processing: If inserting a large number of rows, consider committing in batches or using SqlBulkCopy for performance improvements.
4. Execute the Command
Finally, execute the SQL command using the ExecuteNonQuery() method, which executes the SQL statement against the connection and returns the number of rows affected.
Steps:
ExecuteNonQuery: This method is used when you don’t expect any results to be returned (e.g., with INSERT, UPDATE, or DELETE statements).
Error Handling: Implement error handling to manage exceptions that might occur during the execution.
foreach (DataRow row in dataTable.Rows)
{
cmd.Parameters["@Value1"].Value = row["Column1"];
cmd.Parameters["@Value2"].Value = row["Column2"];
try
{
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
Console.WriteLine("Error inserting row: " + ex.Message);
// Optionally log or handle the error further.
}
}
Considerations:
Transactions: If you’re inserting multiple rows, consider wrapping the operation in a transaction (SqlTransaction) to ensure atomicity (all or nothing).
Exception Handling: Use try-catch blocks to catch and handle any exceptions (e.g., connection issues, data type mismatches).
5. Close the Connection
While the using statement automatically closes the connection, if you opened it manually, make sure to close it using the Close() method.
connection.Close();
Considerations:
Connection Lifecycle: Properly closing the connection ensures it is returned to the pool and resources are freed.
6. Optional: Using SqlBulkCopy
For large datasets, SqlBulkCopy provides a faster way to insert data into a SQL Server database.
using (SqlBulkCopy bulkCopy = new SqlBulkCopy(connection))
{
bulkCopy.DestinationTableName = "YourTable";
try
{
bulkCopy.WriteToServer(dataTable);
}
catch (Exception ex)
{
Console.WriteLine("Error during bulk insert: " + ex.Message);
}
}
Considerations:
Performance: SqlBulkCopy is significantly faster than executing individual INSERT statements, especially for large datasets.
Column Mapping: If your DataTable columns don’t exactly match the database table, you can use ColumnMappings to map them.
Frequently Asked Questions
How can I handle null values in the DataTable when inserting into the database?
When inserting data, you can check for DBNull values in the DataTable and use DBNull.Value to represent null values in the database. For example, cmd.Parameters.AddWithValue(“@Column”, row[“Column”] == DBNull.Value ? DBNull.Value : row[“Column”]);.
Can I insert data from a DataTable into a database using a transaction?
Yes, you can wrap your insert operations in a SqlTransaction. This allows you to ensure that all inserts succeed or fail as a group, providing atomicity and rollback capabilities in case of an error.
What are the security considerations when inserting data from a DataTable?
Always use parameterized queries to prevent SQL injection. Avoid concatenating SQL commands with user input, and ensure your connection strings are securely stored and encrypted if they contain sensitive information.
Concluding Remarks
Inserting DataTable values into a database in C# involves creating a connection, preparing an SQL command, iterating through the DataTable, and executing the command. Error handling, performance considerations, and proper resource management are essential for a robust implementation. For large datasets, SqlBulkCopy can be a more efficient approach.