How Do You Format a Number With Leading Zeros?
Formatting numbers with leading zeros can be useful in various scenarios, such as preparing data for display or ensuring consistent lengths for numerical identifiers. In SQL, there are several techniques to achieve this, and each has its own advantages depending on the specific database system you are using.
This article will cover common methods to format numbers with leading zeros in SQL, with explanations and examples.
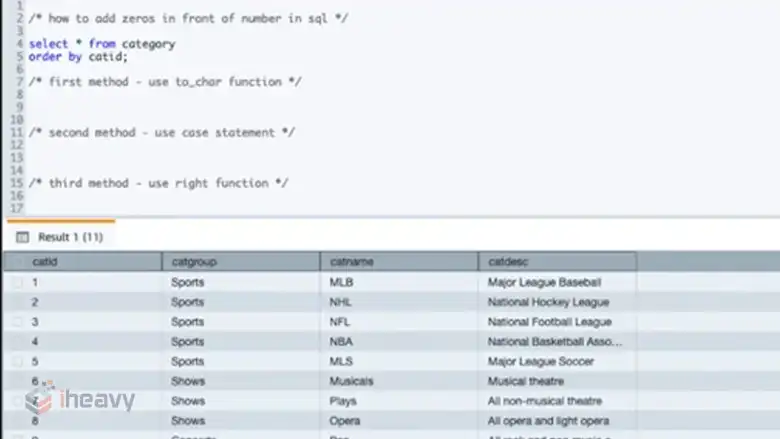
How Do You Format Leading Zeros in SQL?
While the exact syntax might vary slightly between different SQL databases (SQL Server, MySQL, Oracle, PostgreSQL, etc.), the core concept remains the same. Here are the primary methods:
Method 1: Using the LPAD Function
The LPAD (Left Pad) function is a straightforward way to format numbers with leading zeros. It adds a specified character to the left side of a string until the string reaches the desired length. This method is supported by many SQL databases, including MySQL and Oracle.
Syntax:
LPAD(column_name, length, 'pad_string')
Here,
column_name: The column or value you want to pad.
length: The total length of the resulting string.
pad_string: The character to use for padding.
Example:
Suppose we have a table ‘employees’ with an employee_id column containing numeric IDs, and we want each ID to be exactly five digits long.
SELECT
employee_id,
LPAD(employee_id, 5, '0') AS formatted_id
FROM
employees;
In this example, if employee_id is 123, the result will be 00123. The LPAD function pads the number with zeros until the total length is five characters.
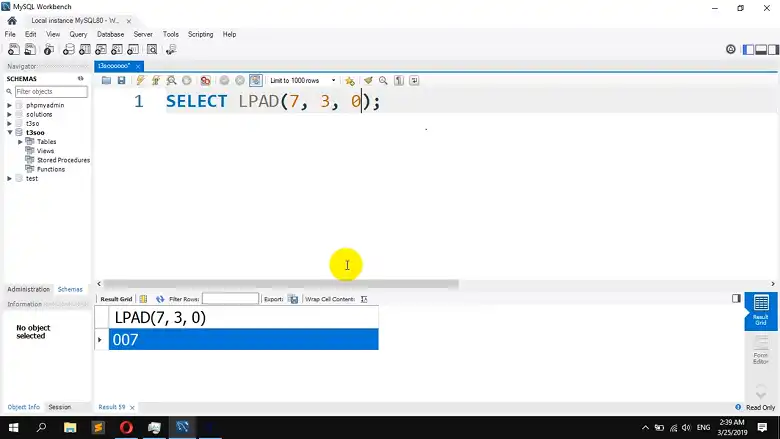
Method 2: Using the FORMAT Function (SQL Server)
In SQL Server, the FORMAT function can be used to achieve similar results. This function is particularly useful for complex formatting needs.
Syntax:
FORMAT(value, 'format_string')
value: The number you want to format.
format_string: The format pattern to apply.
Example:
SELECT
employee_id,
FORMAT(employee_id, '00000') AS formatted_id
FROM
employees;
Here, the FORMAT function ensures that the employee_id is displayed as a five-digit number with leading zeros, such as 00123.
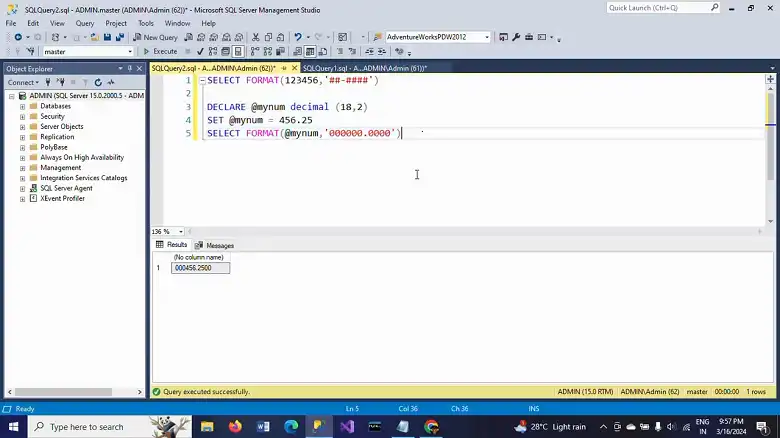
Method 3: Using RIGHT with REPLICATE (SQL Server)
Another approach in SQL Server is to use a combination of the RIGHT and REPLICATE functions. This method offers greater flexibility when dealing with numbers of varying lengths.
Syntax:
RIGHT(REPLICATE('0', desired_length) + CAST(value AS VARCHAR), desired_length)
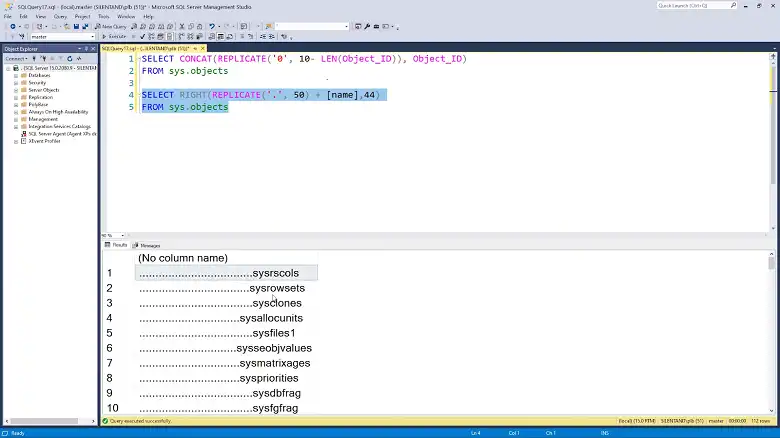
desired_length: The total length of the resulting string.
value: The number to format.
Example:
SELECT
employee_id,
RIGHT(REPLICATE('0', 5) + CAST(employee_id AS VARCHAR), 5) AS formatted_id
FROM
employees;
In this example, REPLICATE(‘0’, 5) generates a string of five zeros. The CAST function converts the employee_id to a string, and RIGHT extracts the rightmost five characters. This method effectively pads the number with leading zeros.
Method 4: Using the TO_CHAR Function (Oracle)
In Oracle databases, the TO_CHAR function is used to convert numbers to strings with specific formatting.
Syntax:
TO_CHAR(value, 'format_string')
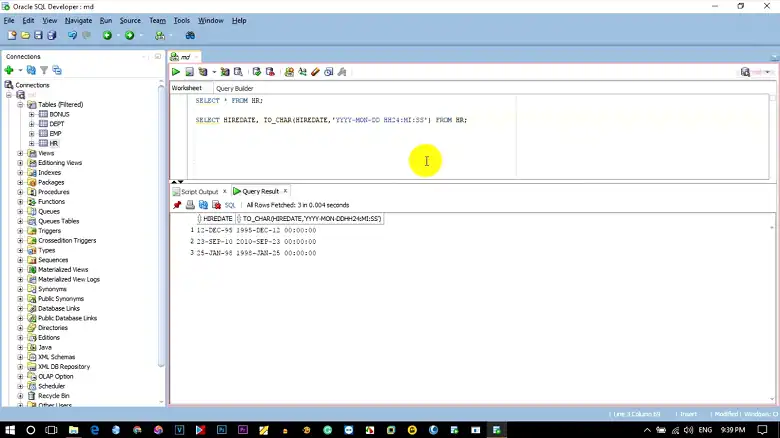
value: The number to format.
format_string: The pattern to apply, using 0 for digits and other characters for literal strings.
Example:
SELECT
employee_id,
TO_CHAR(employee_id, '00000') AS formatted_id
FROM
employees;
The TO_CHAR function in this example formats the employee_id as a five-digit string, ensuring leading zeros for shorter numbers.
Method 5: Using CONCAT with LPAD (PostgreSQL)
In PostgreSQL, you can achieve similar results using a combination of the CONCAT and LPAD functions.
Syntax:
CONCAT('string', LPAD(value::text, desired_length, '0'))
string: An optional string to concatenate.
value: The number to format.
desired_length: The total length of the resulting string.
Example:
SELECT
employee_id,
CONCAT('ID-', LPAD(employee_id::text, 5, '0')) AS formatted_id
FROM
employees;
This example not only formats the number with leading zeros but also adds a prefix (ID-) to each formatted ID, demonstrating the versatility of this method.
Frequently Asked Questions
How to format numbers in SQL query?
To shape date and time information into a specific style, employ the FORMAT function. For altering data from one type to another, such as transforming a number into text, utilize the CAST or CONVERT function.
Can I format numbers with leading zeros in all SQL databases?
Most major SQL databases support formatting numbers with leading zeros, though the specific functions may vary. MySQL, SQL Server, Oracle, and PostgreSQL each provide functions like LPAD, FORMAT, and TO_CHAR.
How can I format negative numbers with leading zeros?
Negative numbers can be formatted similarly, but consider how the minus sign impacts the total length. For example, formatting -12 with a length of 5 would result in -0012.
Conclusion
The methods discussed in this article offer versatile solutions for formatting numbers with leading zeros in SQL. Depending on your database system and specific requirements, you can choose the best approach to achieve your desired results. Happy data formatting!