Pandas Merge Multiple DataFrames
Merging multiple DataFrames is a common task in data analysis, allowing you to combine data from different sources into a single cohesive DataFrame. Pandas, a powerful data manipulation library in Python, provides several functions to facilitate this process. This article will explore various methods to merge multiple DataFrames using Pandas.
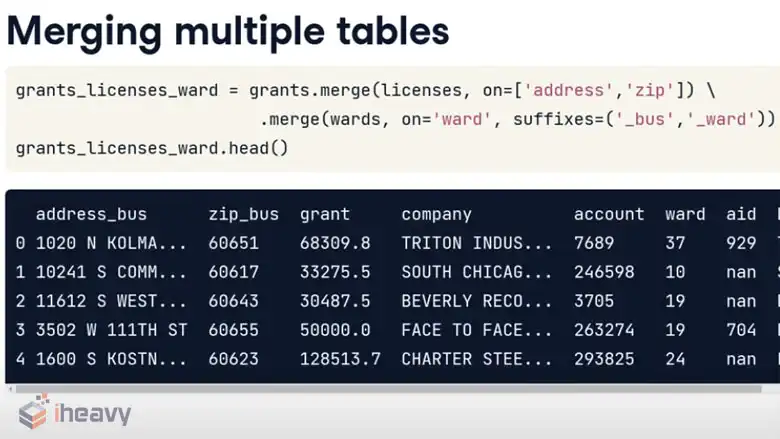
Introduction to Pandas Merging
Pandas offers multiple functions for combining DataFrames, such as merge(), concat(), and join(). These functions allow you to perform different types of merges, including inner, outer, left, and right joins. Understanding these methods and their differences is crucial for effective data manipulation.
Merging with pd.merge()
The merge() function is highly versatile and is used to merge DataFrames based on common columns or indices.
Basic Usage
import pandas as pd
df1 = pd.DataFrame({
‘key’: [‘A’, ‘B’, ‘C’],
‘value1’: [1, 2, 3]
})
df2 = pd.DataFrame({
‘key’: [‘A’, ‘B’, ‘D’],
‘value2’: [4, 5, 6]
})
merged_df = pd.merge(df1, df2, on=’key’, how=’inner’)
print(merged_df)
Merging Multiple DataFrames
You can merge multiple DataFrames by chaining the merge() function.
df3 = pd.DataFrame({
‘key’: [‘A’, ‘B’, ‘C’, ‘D’],
‘value3’: [7, 8, 9, 10]
})
merged_df = pd.merge(df1, df2, on=’key’, how=’inner’)
merged_df = pd.merge(merged_df, df3, on=’key’, how=’inner’)
print(merged_df)
Using pd.concat()
The concat() function concatenates DataFrames along a particular axis (rows or columns).
Concatenating Vertically
df1 = pd.DataFrame({
‘key’: [‘A’, ‘B’, ‘C’],
‘value’: [1, 2, 3]
})
df2 = pd.DataFrame({
‘key’: [‘D’, ‘E’, ‘F’],
‘value’: [4, 5, 6]
})
concatenated_df = pd.concat([df1, df2], axis=0)
print(concatenated_df)
Concatenating Horizontally
df1 = pd.DataFrame({
‘key’: [‘A’, ‘B’, ‘C’],
‘value1’: [1, 2, 3]
})
df2 = pd.DataFrame({
‘value2’: [4, 5, 6]
})
concatenated_df = pd.concat([df1, df2], axis=1)
print(concatenated_df)
Using join()
The join() function is used to combine DataFrames based on their indices.
Basic Usage
df1 = pd.DataFrame({
‘value1’: [1, 2, 3]
}, index=[‘A’, ‘B’, ‘C’])
df2 = pd.DataFrame({
‘value2’: [4, 5, 6]
}, index=[‘A’, ‘B’, ‘D’])
joined_df = df1.join(df2, how=’inner’)
print(joined_df)
Example: Merging Multiple DataFrames
Here’s an example demonstrating the merging of multiple DataFrames with different keys and merge types.
df1 = pd.DataFrame({
‘key1’: [‘A’, ‘B’, ‘C’],
‘value1’: [1, 2, 3]
})
df2 = pd.DataFrame({
‘key2’: [‘A’, ‘B’, ‘D’],
‘value2’: [4, 5, 6]
})
df3 = pd.DataFrame({
‘key3’: [‘A’, ‘B’, ‘C’, ‘D’],
‘value3’: [7, 8, 9, 10]
})
merged_df = pd.merge(df1, df2, left_on=’key1′, right_on=’key2′, how=’outer’)
merged_df = pd.merge(merged_df, df3, left_on=’key1′, right_on=’key3′, how=’outer’)
print(merged_df)
Frequently Asked Questions (FAQ)
How do I merge multiple DataFrames with different keys?
You can use the merge() function with the left_on and right_on parameters to specify different keys for each DataFrame.
What is the difference between merge() and concat()?
merge() is used for SQL-style joins on columns, while concat() is used to concatenate DataFrames along a particular axis (rows or columns).
Can I merge more than three DataFrames?
Yes, you can merge any number of DataFrames by chaining the merge() function multiple times.
Conclusion
Merging multiple DataFrames in Pandas is a powerful way to combine data from various sources into a single DataFrame. Depending on your requirements, you can use merge(), concat(), or join() to achieve the desired result. Understanding the differences between these methods and their appropriate use cases will help you perform efficient and effective data manipulation.