cbind in Pandas: A Comprehensive Guide
When working with datasets in Python, you’ll often need to combine data from multiple sources. One powerful way to achieve this is through column-binding, also known as concatenating data frames. In Pandas, we can accomplish column-binding using the pd.concat() function.
This article goes deep into the intricacies of combining DataFrames in Pandas, providing a comprehensive understanding of the process and its nuances.
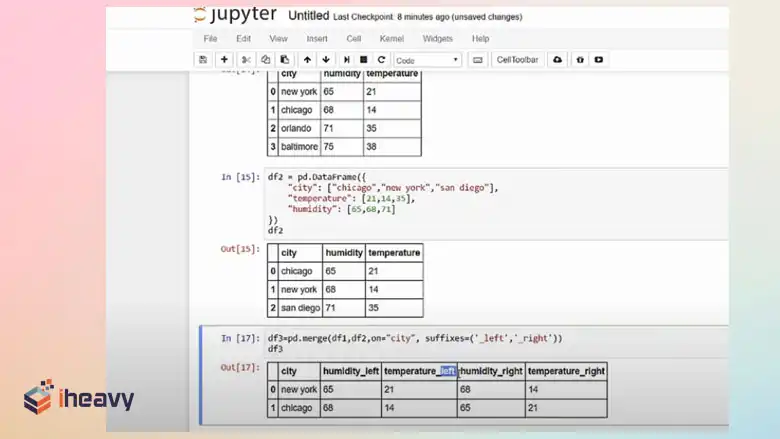
What Does cbind() Do?
cbind() is a function in R used to combine multiple vectors, matrices, or data frames by columns. The name itself is a contraction of “column bind.”
Essentially, it stacks the objects horizontally, creating a new object with the combined columns. The number of rows in all objects to be combined must be identical for cbind() to work correctly.
Example:
# Create vectors
x <- c(1, 2, 3)
y <- c(4, 5, 6)
# Combine vectors using cbind
combined <- cbind(x, y)
print(combined)
This code will output a matrix with two columns:
What Is the Equivalent of cbind in Pandas?
Pandas doesn’t have a direct equivalent to cbind, but pd.concat serves as the primary tool for achieving similar results. It offers greater flexibility and control over the merging process.
Column binding (or cbind) allows us to horizontally concatenate data frames, combining their columns. If you’re familiar with R, you’ll recognize this as similar to the cbind() function. However, there’s a key difference: while R’s cbind() ignores index alignment, Pandas’ concat() function tries to align indices. Let’s dive into how to use it effectively.
How to cbind a DataFrame in Python?
To concatenate DataFrames column-wise using pd.concat, set the axis parameter to 1:
import pandas as pd
# Sample DataFrames
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'C': [7, 8, 9], 'D': [10, 11, 12]})
# Concatenate column-wise
combined_df = pd.concat([df1, df2], axis=1)
print(combined_df)
Output:
In this code, axis=1 specifies that the concatenation should be performed along the columns. The resulting DataFrame combined_df will have columns from both df1 and df2.
Key Considerations
- Index Alignment: Unlike R, Pandas considers indices when concatenating DataFrames. If the indices of the input DataFrames do not align, the resulting DataFrame might have missing values or unexpected behavior.
- Overlapping Columns: When DataFrames share column names, pd.concat creates a hierarchical index by default. To avoid this, use the ignore_index=True parameter.
- Join Methods: For more complex merging based on specific columns, consider using pd.merge or join methods.
Example with Index Alignment
import pandas as pd
# Sample DataFrames with different indices
df1 = pd.DataFrame({'A': [1, 2, 3]}, index=[0, 1, 2])
df2 = pd.DataFrame({'C': [7, 8, 9]}, index=[1, 2, 3])
# Concatenate with alignment
combined_df = pd.concat([df1, df2], axis=1)
print(combined_df)
Output:
In this example, the indices of df1 and df2 partially overlap. pd.concat aligns the DataFrames based on their indices, resulting in missing values where indices don’t match.
Handling Mismatched Indices
To ensure proper alignment and avoid unexpected results, it’s often recommended to reset the indices of DataFrames before concatenation:
combined_df = pd.concat([df1.reset_index(drop=True), df2.reset_index(drop=True)], axis=1)
Output:
By using reset_index(drop=True), we create new indices starting from 0 for both DataFrames, ensuring correct alignment during concatenation.
Frequently Asked Questions
Does cbind match row names?
This function aligns data based on row labels, so it doesn’t require identical row counts or matching row label order across datasets.
What is the difference between merge and cbind in R?
Data can be combined either vertically (by rows) or horizontally (by columns). R uses `rbind()` and `cbind()` to combine matrices in these ways, respectively. For combining DataFrames, the more versatile `merge()` function offers greater flexibility than `cbind()`.
Conclusion
Understanding pd.concat is crucial for effectively combining DataFrames in Pandas. By considering index alignment, overlapping columns, and join methods, you can achieve the desired results. While pd.concat provides flexibility, it’s essential to choose the appropriate method based on your specific data and requirements.