How to Create an Empty Array of Struct in Hive?
Hive, while powerful for data warehousing, can present challenges when dealing with complex data structures like arrays of structs. Creating an empty array of struct is one such challenge.
Directly assigning an empty array to a struct column often results in errors. Therefore, you have to use walkarounds for creating such arrays. In this comprehensive guide, we’ll explore different approaches and provide detailed examples.
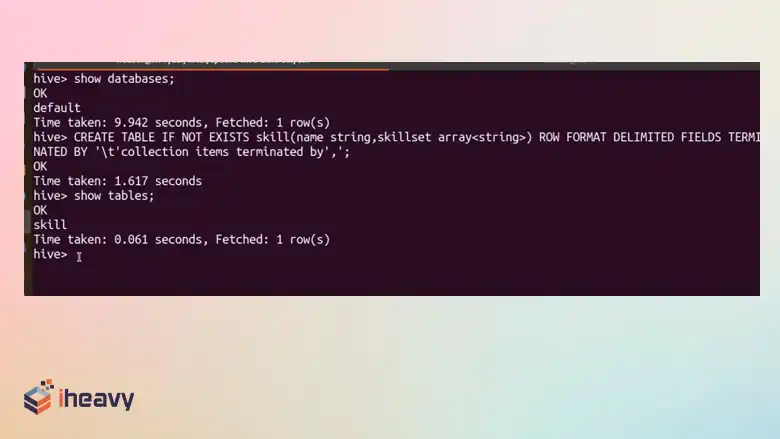
How Do I Create an Empty Array of Struct in Hive?
An array of structs in Hive allows you to store structured data within an array. However, creating an empty array of structs isn’t as straightforward if not impossible. Let’s break down the walkaround solutions for you.
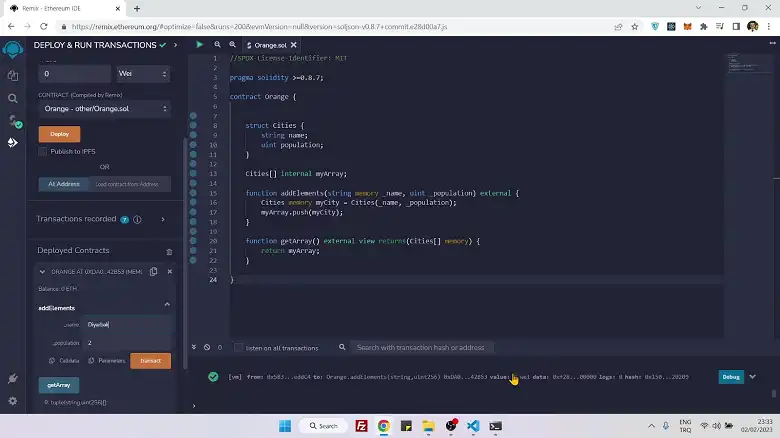
Solution 1: Using CASE Statements
The CASE statement allows you to handle conditions and return different values based on those conditions. We’ll leverage it to create an empty array of the correct type.
Example:
Suppose we want to create an empty array of structs when the employee’s department is not “Engineering.” Otherwise, we want to include a single struct with default values.
SELECT
emp_id, -- Select the employee ID
emp_name, -- Select the employee name
CASE
WHEN department <> 'Engineering' THEN -- If the department is not 'Engineering'
array() -- Create an empty array for job_info
ELSE -- If the department is 'Engineering'
array(named_struct('name', NULL, 'jobslots', NULL)) -- Create an array with a single named struct containing null values for 'name' and 'jobslots'
END AS job_info -- Assign the result of the CASE expression to the job_info column
FROM employees; -- Select data from the employees table
In this example:
- If the department is not “Engineering,” we return an empty array.
- Otherwise, we create a struct with NULL values for name and jobslots.
Solution 2: Collecting Structs
When working with arrays of structs resulting from joins or other operations, consider using collect_list or collect_set. These functions automatically handle empty arrays.
Example:
Suppose we have a join between the employees and departments tables. We want to collect the job information for each employee.
SELECT
e.emp_id, -- Select the employee ID from the employees table (alias 'e')
e.emp_name, -- Select the employee name from the employees table (alias 'e')
collect_list(named_struct('name', NULL, 'jobslots', NULL)) AS job_info -- Create a named struct with null values for 'name' and 'jobslots', collect them into a list for each employee
FROM employees e -- Select data from the employees table with alias 'e'
JOIN departments d ON e.department_id = d.department_id; -- Join employees table with departments table based on matching department IDs
Solution 3: Leveraging collect_list and Joins
1. Create a dummy table: This table will be used to generate an empty array.
2. Join the dummy table: Join the dummy table with your main table on a condition that will never be met.
3. Use collect_list: Aggregate the desired struct columns using collect_list to create an empty array.
-- Create a dummy table with a single column
CREATE TABLE dummy_table (dummy_col INT);
-- Create the main table with columns for ID, other data, and an array of structs
CREATE TABLE your_table (id INT, other_cols STRING, array_of_structs ARRAY<STRUCT<field1:STRING, field2:INT>>);
-- Insert data into your_table, creating an empty array of structs for each row
INSERT INTO your_table
SELECT
id,
other_cols,
-- Use collect_list to aggregate structs, but since there's no matching row in dummy_table, the result will be an empty array
collect_list(STRUCT(NULL AS field1, NULL AS field2)) AS array_of_structs
FROM your_table a
-- Left join with dummy_table on a condition that will never be true
LEFT OUTER JOIN dummy_table b ON a.id = b.dummy_col;
Solution 4: Using inline Function
You can also use the inline function to directly create an empty array of structs. Here’s how.
-- Create an empty array of structs using the inline function
INSERT INTO your_table
SELECT
id,
other_cols,
inline(ARRAY<STRUCT(field1:STRING, field2:INT)>()) AS array_of_structs
FROM your_table;
Important Considerations While Creating an Empty Array of Struct
While creating an empty array of struct in Hive, you should keep the following things in mind.
Data Types: Ensure the data types of the struct fields match the expected schema of your array of structs column.
Performance: The performance of these methods can vary depending on data volume and table structure. Consider testing different approaches to find the optimal solution.
Null Handling: If null values are acceptable in your array, the third approach might be sufficient. However, if you need an empty array, the first two methods are more suitable.
Frequently Asked Questions
Can you create an empty array of structs without using NULL values?
Unfortunately, due to Hive’s type system, NULL values are necessary to match the desired array type.
Can I directly assign an empty array to a struct column in Hive?
No, Hive doesn’t support direct assignment of empty arrays to struct columns.
Is it possible to modify the elements within the empty array after creation?
Yes, you can use Hive’s built-in array functions like array_append, array_remove, etc. to modify the array after creating it.
Conclusion
Creating an empty array of struct in Hive might seem tricky, but with these methods, it’s achievable. Understanding your specific use case will help you choose the best approach. Have you tried creating empty arrays of structs in Hive before? Share your experiences and challenges in the comments!