How to Use JSON_ARRAYAGG in JSON_OBJECT Without Escaping Content in MySQL
In MySQL, working with JSON data has become increasingly common due to the flexibility and power it provides for handling complex data structures. One common requirement is to aggregate JSON arrays within JSON objects without escaping the content.
This article will guide you through the process of achieving this using JSON_ARRAYAGG and JSON_OBJECT functions.
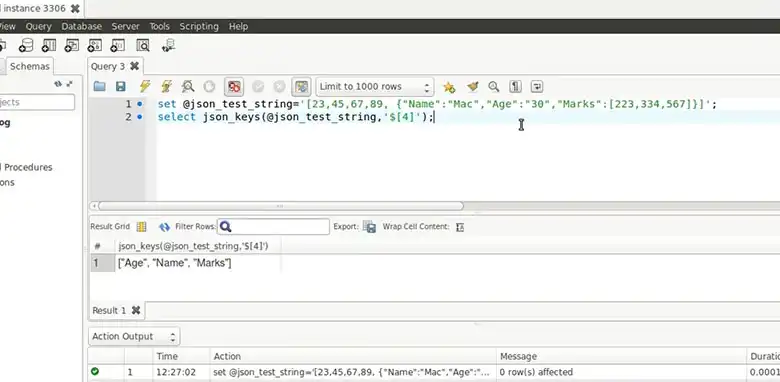
What is JSON_ARRAYAGG?
JSON_ARRAYAGG is a powerful MySQL function used to aggregate values into a single JSON array. It is particularly useful for converting data from multiple rows in a table into a JSON array format. This function can be employed to create complex JSON structures by aggregating various data points into an array.
Key Features of JSON_ARRAYAGG
Aggregation: Combines multiple values into a single JSON array.
Flexibility: Works with a wide range of data types, making it versatile for different use cases.
Nested Structures: Can be used in conjunction with other JSON functions to create nested JSON objects and arrays.
Basic Usage of JSON_ARRAYAGG
Here’s a basic example of how JSON_ARRAYAGG can be used:
SELECT JSON_ARRAYAGG(column_name) AS json_array FROM table_name;
This query will aggregate the values from column_name in table_name into a JSON array.
Example: Consider a table named employees with the following data:
id | name |
1 | Alice |
2 | Bob |
3 | Charlie |
Using JSON_ARRAYAGG, you can aggregate these names into a JSON array:
SELECT JSON_ARRAYAGG(name) AS names_array FROM employees;
The result would be:
{
"names_array": ["Alice", "Bob", "Charlie"]
}
What is JSON_OBJECT?
Before diving into the solution, it’s essential to understand what this function does as well.
JSON_OBJECT(key, value, …): This function creates a JSON object by pairing keys and values. For instance, JSON_OBJECT(‘name’, ‘John’, ‘age’, 30) would produce {“name”: “John”, “age”: 30}.
The Problem with Escaping Content
When combining these functions, you might encounter issues where JSON content gets escaped, leading to undesirable results. For example, trying to nest a JSON array within a JSON object can result in the array being treated as a string:
SELECT JSON_OBJECT('data', JSON_ARRAYAGG(value)) FROM my_table;
Instead of getting {“data”: [1, 2, 3]}, you might get {“data”: “[1, 2, 3]”}.
The Solution: Using JSON_ARRAYAGG in JSON_OBJECT Without Escaping Content
To correctly nest a JSON array within a JSON object without escaping the content, you can use the following approach:
1. Ensure JSON Data Type Consistency: Make sure the data being aggregated is of JSON type. You can achieve this by casting values to JSON if necessary.
2. Use a Subquery: Leveraging a subquery can help in creating the desired JSON structure without escaping issues.
Here is a step-by-step example:
1. Create Sample Data: Assume you have a table named my_table with two columns: id and value.
CREATE TABLE my_table (
id INT,
value VARCHAR(255)
);
INSERT INTO my_table (id, value) VALUES
(1, 'John'),
(2, 'Jane'),
(3, 'Doe');
2. Aggregate JSON Array: Use JSON_ARRAYAGG in a subquery to aggregate the values into a JSON array.
SELECT JSON_ARRAYAGG(value) AS json_array FROM my_table;
This will produce the array [“John”, “Jane”, “Doe”].
3. Nest the JSON Array in a JSON Object: Use the aggregated JSON array within JSON_OBJECT.
SELECT JSON_OBJECT(‘data’, (SELECT JSON_ARRAYAGG(value) FROM my_table)) AS json_object;
This will produce the desired result: {“data”: [“John”, “Jane”, “Doe”]}.
Here’s the complete SQL query:
SELECT JSON_OBJECT('data', (SELECT JSON_ARRAYAGG(value) FROM my_table)) AS json_object;
This approach ensures that the JSON array is correctly nested within the JSON object without escaping its content.
Frequently Asked Questions
How to get value from JSON array object in MySQL?
To retrieve a specific item from a JSON array in MySQL, you should use the `->` operator combined with the `[item_number]` JSON path syntax.
How to create a JSON array of JSON objects?
You can create a JSON array of JSON objects by using JsonParse. This function generates a JSON array or JSON object from a given string. It returns a handle to the created JSON array or JSON object.
Conclusion
Handling JSON data in MySQL can be powerful yet tricky, especially when it comes to combining functions like JSON_ARRAYAGG and JSON_OBJECT. By following the steps outlined in this article, you can ensure that your JSON content is properly structured and free from unwanted escaping. This technique can be useful for creating complex JSON documents directly within your SQL queries.