How to Map Native SQL Results to OneToMany with SqlResultSetMapping in JPA
Java Persistence API (JPA) provides a way to bridge the gap between relational databases and object-oriented programming. One common task is to execute native SQL queries and map the results to entity relationships, such as OneToMany.
This article explains how to map native SQL results to a OneToMany relationship using SqlResultSetMapping in JPA.
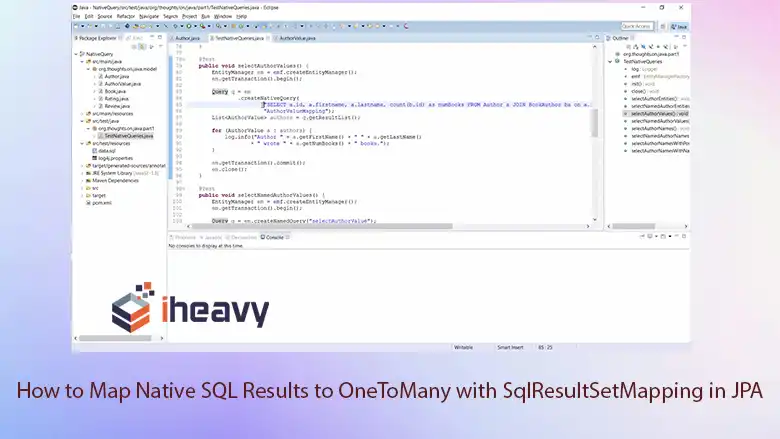
Understanding SqlResultSetMapping
SqlResultSetMapping is an annotation used to map the results of native SQL queries to entity classes. This is especially useful when you need to execute complex queries that JPQL (Java Persistence Query Language) cannot handle efficiently.
Prerequisites
Before you begin, ensure you have the following:
- A basic understanding of JPA and entity relationships.
- A JPA project setup with entities and a database connection.
- Familiarity with native SQL queries.
A Step-by-Step Guide of Using SqlResultSetMapping in JPA
Step 1: Define the Entities
Consider two entities: Author and Book. An Author can have multiple Books, representing a OneToMany relationship. The OneToMany relationship allows an author to have multiple books (like a writer with a shelf full of their works), and each book points back to its author (like a signed dedication inside the cover).
Author Entity
The Author entity represents an author in your application. It has the following attributes:
id: A unique identifier for each author (usually generated automatically).
name: The name of the author.
books: A @OneToMany relationship with the Book entity. This means that an author can have multiple books associated with them. The mappedBy attribute specifies that the relationship is managed by the author field in the Book entity.
The @Entity annotation indicates that this class is an entity mapped to a database table.
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Book> books;
// Getters and setters
}
Book Entity
The Book entity represents a book in your application. It has the following attributes:
id: A unique identifier for each book.
title: The title of the book.
author: A @ManyToOne relationship with the Author entity. This means that each book is associated with a single author. The @JoinColumn annotation specifies the foreign key column (author_id) that links the book to its author.
The @Entity annotation indicates that this class is an entity mapped to a database table.
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// Getters and setters
}
Step 2: Define the SqlResultSetMapping
The @SqlResultSetMappings annotation allows you to define named result mappings for native SQL queries. In our case, we’re creating a mapping named “AuthorBookMapping.”
Inside the @SqlResultSetMapping, we specify how the SQL query results should be mapped to our Java entities (Author and Book).
@SqlResultSetMappings({
@SqlResultSetMapping(
name = "AuthorBookMapping", // Define a named result mapping called "AuthorBookMapping"
entities = {
@EntityResult(entityClass = Author.class, fields = {
@FieldResult(name = "id", column = "author_id"), // Map SQL column "author_id" to Author's "id" field
@FieldResult(name = "name", column = "author_name") // Map SQL column "author_name" to Author's "name" field
}),
@EntityResult(entityClass = Book.class, fields = {
@FieldResult(name = "id", column = "book_id"), // Map SQL column "book_id" to Book's "id" field
@FieldResult(name = "title", column = "book_title"), // Map SQL column "book_title" to Book's "title" field
@FieldResult(name = "author", column = "author_id") // Map SQL column "author_id" to Book's "author" field
})
}
)
})
@Entity
public class Author {
// Existing fields and annotations
}
Remember that this mapping helps JPA understand how to populate the Author and Book entities when executing native SQL queries. It ensures that the results are correctly associated with the corresponding entity fields.
Step 3: Execute the Native SQL Query
We’re constructing a native SQL query that retrieves information from both the Author and Book tables. The query performs a LEFT JOIN between the Author and Book tables based on the author_id relationship.
// Create a native SQL query to retrieve author and book information
String sql = "SELECT a.id as author_id, a.name as author_name, b.id as book_id, b.title as book_title " +
"FROM Author a LEFT JOIN Book b ON a.id = b.author_id";
// Execute the query using the EntityManager and the "AuthorBookMapping" result mapping
List<Object[]> results = entityManager.createNativeQuery(sql, "AuthorBookMapping").getResultList();
// Process the results
for (Object[] result : results) {
Author author = (Author) result[0]; // Extract the Author entity from the first element of the result array
Book book = (Book) result[1]; // Extract the Book entity from the second element of the result array
System.out.println("Author: " + author.getName() + ", Book: " + book.getTitle());
}
Here, we used the entityManager.createNativeQuery(sql, “AuthorBookMapping”) method to create a native query. The “AuthorBookMapping” argument specifies the result mapping we defined earlier.
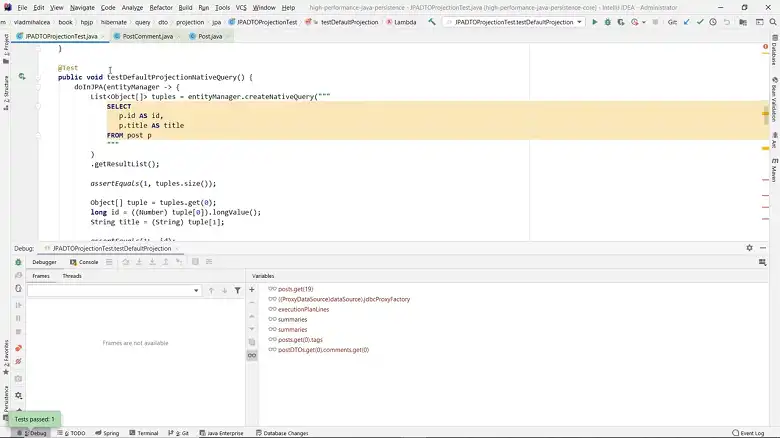
The getResultList() method retrieves the query results as a list of Object[] arrays. Each array contains the mapped data for an author-book pair. In the loop, we extracted the Author and Book entities from each result array.
Step 4: Handling the Results
1. Create an Author Map (authorMap):
Initialize a Map<Long, Author> called authorMap. This map will store authors by their unique IDs (Long type).
2. Iterate Through Query Results:
For each result in the query results (each result is an Object[] array):
- Extract the Author entity from the first element (result[0]).
- Extract the Book entity from the second element (result[1]).
3. Author Handling:
If the author is not already in the map (!authorMap.containsKey(author.getId())):
- Add the author to the map using their ID as the key (author.getId()).
- Initialize an empty list of books for the author (author.setBooks(new ArrayList<>())).
If a book exists (i.e., book != null):
- Associate the book with the author by setting the book’s author reference (book.setAuthor(author)).
- Add the book to the author’s list of books (author.getBooks().add(book)).
4. Create the List of Authors:
Finally, create a list of authors by extracting the values from the authorMap.
// Create a map to store authors by their IDs
Map<Long, Author> authorMap = new HashMap<>();
// Iterate through the query results
for (Object[] result : results) {
Author author = (Author) result[0]; // Extract the Author entity
Book book = (Book) result[1]; // Extract the Book entity
// If the author is not already in the map, add them and initialize their books list
if (!authorMap.containsKey(author.getId())) {
authorMap.put(author.getId(), author);
author.setBooks(new ArrayList<>());
}
// If a book exists, associate it with the author
if (book != null) {
book.setAuthor(author);
author.getBooks().add(book);
}
}
// Create a list of authors from the map values
List<Author> authors = new ArrayList<>(authorMap.values());
This process ensures that the OneToMany relationship between authors and books is correctly maintained. Each author has their associated books, and each book points back to its author.
Frequently Asked Questions
Can I use JPQL instead of native SQL for complex queries?
JPQL can handle many complex queries, but native SQL is more powerful for certain scenarios, such as using database-specific features or performance optimizations.
How do I handle multiple result sets with SqlResultSetMapping?
You can define multiple EntityResult annotations within a single SqlResultSetMapping to handle multiple result sets.
Can I map the results to DTOs (Data Transfer Objects) instead of entities?
Yes, you can map the results to DTOs using ConstructorResult in SqlResultSetMapping.
Conclusion
Mapping native SQL results to OneToMany relationships in JPA using SqlResultSetMapping provides flexibility and control over complex queries. By following the steps outlined in this article, you can efficiently manage entity relationships and handle advanced database operations. This approach ensures that you can leverage the full power of SQL while maintaining the benefits of JPA for ORM (Object-Relational Mapping).