How to Choose a Random Row as Aggregate Function in Hive
How to Choose a Random Row as Aggregate Function in Hive
Selecting a random row as an aggregate function involves grouping data and then picking a random row from each group. This can be useful in various scenarios, such as sampling data, random selection for A/B testing, or anonymizing datasets.
Unlike traditional databases, Apache Hive does not have a built-in function to directly select a random row as an aggregate. However, there are several workarounds and methods to achieve this functionality. This article will explore how to choose a random row as an aggregate function in Hive.
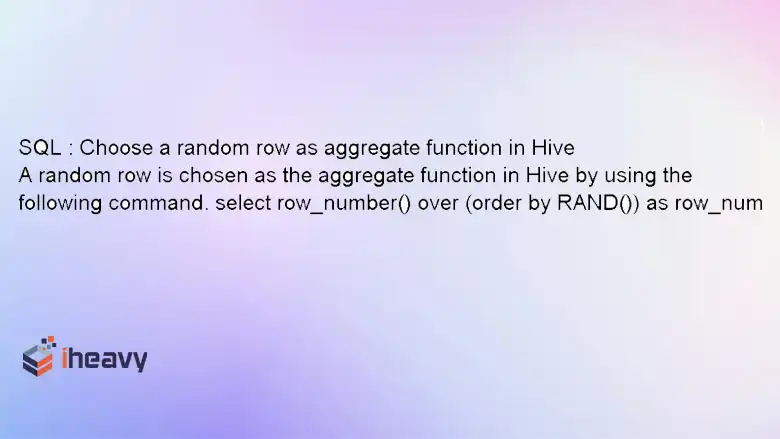
Potential Methods to Choose a Random Row
You can choose a random row as an aggregate function in Hive using three distinct methods, the easiest one being the use of Window functions. However, you can define your own function to achieve the goal as well. For convenience, we’ll also go through a third method that makes use of Lateral view and Explode.
Method 1: Using Window Functions
One method to achieve this is by using Hive’s window functions. But before that, let’s address why you should consider using window functions to choose a random row as an aggregate function in Hive:
- Efficient Sampling:
- Window functions allow you to efficiently sample data without scanning the entire dataset.
- By selecting random rows within each group, you can create representative samples for analysis or testing.
- A/B Testing and Experimentation:
- When conducting A/B tests or experiments, random selection ensures unbiased results.
- Window functions help you choose random treatment groups or variations.
- Data Anonymization:
- For privacy reasons, you might need to anonymize data by selecting random records.
- Window functions provide a straightforward way to achieve this.
Here’s how to implement this in practice:
— Select columns col1, col2, and col3
SELECT
col1,
col2,
col3
FROM (
— Generate row numbers within each group based on random order
SELECT
col1,
col2,
col3,
ROW_NUMBER() OVER (PARTITION BY group_col ORDER BY RAND()) as rn
FROM
your_table
) subquery
— Filter rows where the row number is 1 (randomly selected row)
WHERE
subquery.rn = 1;
In this example:
- group_col is the column by which you want to group the data.
- RAND() generates a random number for each row.
- ROW_NUMBER() OVER (PARTITION BY group_col ORDER BY RAND()) assigns a unique row number to each row within the group, ordered randomly.
- The outer query selects rows where the row number is 1, effectively choosing a random row from each group.
Method 2: Using UDFs (User-Defined Functions)
Let’s explore how to choose a random row as an aggregate function in Hive using User-Defined Functions (UDFs). I’ll provide step-by-step instructions along with code snippets and comments:
1. Create a Custom UDF
First, you’ll need to write a Java class that defines your custom UDF. Let’s call it RandomRowUDF.
The UDF will take an array of rows (e.g., values from a specific column) and return a randomly selected value.
Java Code for RandomRowUDF:
import org.apache.hadoop.hive.ql.exec.UDF;
import org.apache.hadoop.io.Text;
public class RandomRowUDF extends UDF {
public Text evaluate(String[] rows) {
int randomIndex = (int) (Math.random() * rows.length);
return new Text(rows[randomIndex]);
}
}
2. Compile and Package the UDF:
Compile the Java code and package it into a JAR file (e.g., randomrowudf.jar).
3. Register the UDF in Hive:
Upload the JAR file to HDFS or a local directory accessible by Hive. In Hive, execute the following commands:
ADD JAR /path/to/randomrowudf.jar;
CREATE TEMPORARY FUNCTION random_row AS ‘com.yourpackage.RandomRowUDF’;
4. Use the UDF in Your Query:
Now you can use the random_row UDF in your queries.
Example query:
— Select the group column and a random value from col1
SELECT
group_col,
random_row(collect_list(col1)) AS random_col1
FROM
your_table
GROUP BY
group_col;
Explanation:
- collect_list(col1) collects all values from col1 within each group.
- random_row UDF selects a random value from the collected list.
Remember that UDFs provide flexibility but may have performance implications. Choose the method that best suits your specific use case.
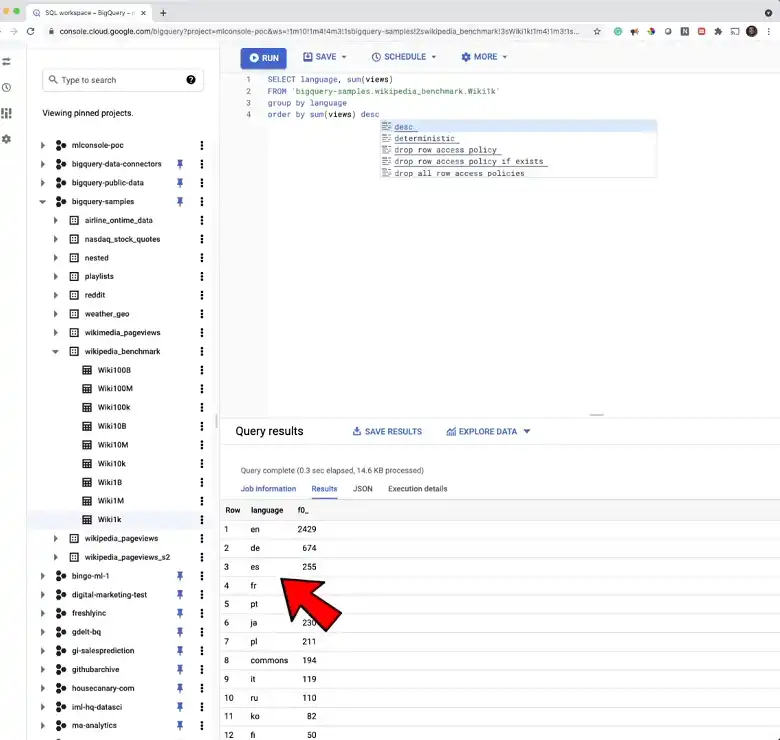
Method 3: Using Lateral View and Explode
You can also use LATERAL VIEW and EXPLODE to achieve similar results. This method is more complex but can be useful in certain scenarios.
What is LATERAL VIEW?
The LATERAL VIEW clause is used in conjunction with generator functions (such as EXPLODE) to manipulate complex data types like arrays, maps, and structs. It generates a virtual table containing one or more rows based on the output of the generator function. For each original output row, LATERAL VIEW applies the rows generated by the function.
Syntax: LATERAL VIEW [OUTER] generator_function(expression [, …]) [table_alias] AS column_alias [, …]
Example:
SELECT * FROM person
LATERAL VIEW EXPLODE(ARRAY(30, 60)) AS c_age
LATERAL VIEW EXPLODE(ARRAY(40, 80)) AS d_age;
This query creates multiple rows for each combination of c_age and d_age.
What is EXPLODE?
EXPLODE is a generator function that splits an array or map into individual rows. It creates a new row for each non-null element in the array or map.
Example:
SELECT c_age, COUNT(1) FROM person
LATERAL VIEW EXPLODE(ARRAY(30, 60)) AS c_age
LATERAL VIEW EXPLODE(ARRAY(40, 80)) AS d_age
GROUP BY c_age;
This query counts occurrences of c_age values.
How to Use LATERAL VIEW and EXPLODE to Choose a Random Row?
Here’s a complete example of how to utilize these two functions together to choose a random row as an aggregate function.
— Select the columns we need for the final result
SELECT
group_col,
col1,
col2,
col3
FROM (
— Create a subquery to generate row numbers for each group
SELECT
group_col,
col1,
col2,
col3,
ROW_NUMBER() OVER (PARTITION BY group_col ORDER BY RAND()) as rn
FROM
your_table
) temp_table
— Use LATERAL VIEW to explode the array into separate rows
LATERAL VIEW
explode(array(col1, col2, col3)) exploded_table AS col
— Filter only the rows with row number = 1 (randomly chosen row)
WHERE
temp_table.rn = 1;
In this example:
- The outer query selects the desired columns (group_col, col1, col2, and col3) from the intermediate result.
- The subquery generates row numbers (rn) for each group of group_col using the ROW_NUMBER() window function.
- The LATERAL VIEW clause explodes the array formed by col1, col2, and col3 into separate rows.
- Finally, we filter only the rows where the row number is 1 (i.e., a randomly chosen row).
Random Row Selection: Choosing the Right Approach
Let’s summarize the advantages and disadvantages of the three methods for choosing a random row as an aggregate function in Hive: window functions, user-defined functions (UDFs), and LATERAL VIEW with EXPLODE.
Method | Advantages | Limitations |
Window Functions | – Simple to use with built-in functions like ROW_NUMBER() or RAND() for random ordering. | – Requires sorting the entire dataset, which can be expensive for large tables. |
– Provides flexibility for custom ordering within partitions. | – May not be truly random if data distribution is skewed. | |
– Works well for small to medium-sized datasets. | – Limited to the capabilities of available window functions. | |
User-Defined Functions | – Customizable and extensible by writing your own UDFs. | – Requires additional development effort to create and maintain UDFs. |
– Can handle complex logic and custom randomization algorithms. | – Performance overhead due to invoking UDFs. | |
– Suitable for scenarios where built-in functions fall short. | – May not be as optimized as built-in functions. | |
LATERAL VIEW with EXPLODE | – Efficiently handles arrays and maps by exploding them into separate rows. | – Requires understanding of LATERAL VIEW and EXPLODE. |
– Works well for scenarios where you want to select random elements from an array. | – Limited to array or map data types. | |
– Can be combined with other LATERAL VIEW operations for more complex transformations. | – May not be as straightforward as window functions or UDFs. |
Frequently Asked Questions
Can I use the LIMIT clause to select a random row?
No, the LIMIT clause alone cannot ensure randomness within each group. It simply limits the number of rows returned by the query.
Is using RAND() efficient for large datasets?
Using RAND() can be computationally expensive for large datasets as it generates a random number for each row. It’s advisable to test performance on your specific dataset.
Are there any built-in Hive functions to select a random row?
Hive does not have a direct built-in function to select a random row as an aggregate. The methods described above are common workarounds.
Conclusion
In summary, window functions are convenient but resource-intensive, UDFs offer customization but come with development overhead, and LATERAL VIEW with EXPLODE is efficient for array-based random selection. Each method has its own advantages when it comes to choosing a random row as an aggregate function. So. feel free to choose the method that best aligns with your specific use case and dataset size.