CTE vs Subquery | Understanding the Differences and Use Cases
When working with SQL, you’ll often need to write complex queries to extract and manipulate data. Two powerful tools for structuring these queries are Common Table Expressions (CTEs) and subqueries. Understanding when to use each can help optimize your SQL queries for readability and performance.
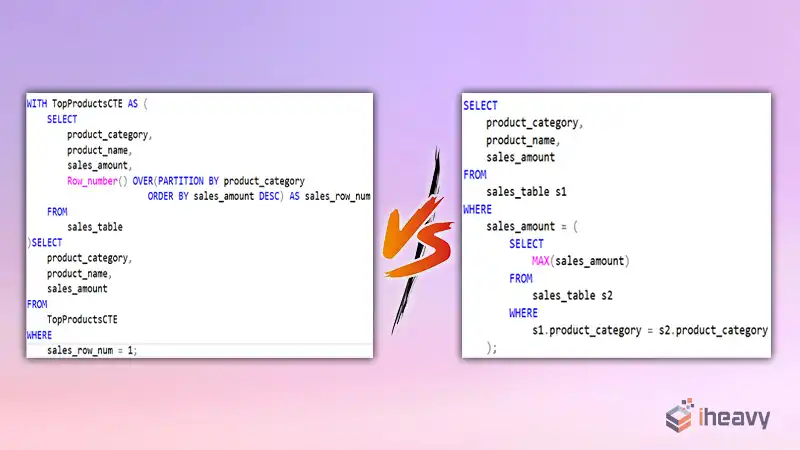
What is a Subquery
A subquery, also known as an inner query or nested query, is a query embedded within another SQL query. Subqueries can be used in various parts of a SQL statement, including the SELECT, FROM, WHERE, and HAVING clauses.
Example of a Subquery
SELECT employee_id, employee_name
FROM employees
WHERE department_id = (SELECT department_id
FROM departments
WHERE department_name = 'Sales');
In this example, the inner query retrieves the department_id for the ‘Sales’ department, and the outer query uses this result to find all employees in that department.
What is a Common Table Expression (CTE)
A Common Table Expression (CTE) is a temporary result set that you can reference within a SELECT, INSERT, UPDATE, or DELETE statement. CTEs are defined using the WITH keyword, followed by the CTE name and the query that generates the result set.
Example of a CTE
WITH SalesDepartment AS (
SELECT department_id
FROM departments
WHERE department_name = 'Sales'
)
SELECT employee_id, employee_name
FROM employees
WHERE department_id = (SELECT department_id FROM SalesDepartment);
In this example, the CTE SalesDepartment retrieves the department_id for the ‘Sales’ department, and the main query uses this result to find all employees in that department.
Key Differences Between CTEs and Subqueries
Readability and Maintainability
CTEs
CTEs are typically more readable and easier to maintain, especially for complex queries. They allow you to break down the query into logical, named parts.
Subqueries
Subqueries can become difficult to read and maintain when nested deeply or when the query logic is complex.
Performance
CTEs
CTEs do not inherently offer performance benefits over subqueries. The performance depends on the SQL engine’s optimization. However, CTEs are often used for recursive queries, which can be more efficient than equivalent subquery approaches.
Subqueries
In some cases, subqueries can be more performant, especially if they are simple and executed only once. The SQL optimizer may handle them more efficiently.
Scope and Reusability
CTEs
CTEs can be referenced multiple times within the same query, making them useful for repetitive calculations or intermediate result sets.
Subqueries
Subqueries are defined and used in place. They cannot be reused within the same query, which may lead to repeated logic.
Recursion
CTEs
CTEs support recursive queries, which are useful for hierarchical or tree-structured data.
Subqueries
Subqueries do not support recursion.
When to Use CTEs
Complex Queries
Use CTEs to simplify complex queries by breaking them into manageable parts.
Recursion
Use CTEs for recursive queries to handle hierarchical data.
Readability
Use CTEs to improve query readability and maintainability.
When to Use Subqueries
Simple Queries
Use subqueries for simple, one-off calculations or filters.
Inline Operations
Use subqueries when you need to perform inline operations within SELECT, WHERE, or other clauses.
Performance
In some cases, subqueries may offer better performance for specific use cases, depending on the SQL optimizer.
Frequently Asked Questions
Can CTEs be used in all SQL databases?
Most modern SQL databases support CTEs, including PostgreSQL, SQL Server, MySQL (version 8.0 and above), and Oracle. However, it’s always best to check your specific database documentation.
Are CTEs more efficient than subqueries?
Not necessarily. The efficiency of CTEs versus subqueries depends on the specific query and the SQL optimizer. While CTEs improve readability and maintainability, they do not always offer performance benefits.
Can subqueries be recursive?
No, subqueries do not support recursion. If you need to perform recursive operations, you should use CTEs, which are designed to handle such cases.
How do CTEs handle large datasets?
CTEs handle large datasets similarly to subqueries. The performance depends on the SQL engine’s ability to optimize the query. For very large datasets, indexing and query optimization techniques should be applied.
Conclusion
Understanding the differences between CTEs and subqueries is crucial for writing efficient and maintainable SQL queries. CTEs offer better readability and are ideal for complex and recursive queries, while subqueries are useful for simple inline operations. By choosing the right approach for your specific use case, you can optimize your SQL queries for both performance and clarity.